Current React interview questions focus on checking how well you get and use the core React concepts.
Landing a React job is not so easy. Interviews hit you with questions about components, state, hooks, and performance. If you’re not ready, you might freeze up.
I’ve put together 15 must-know React interview questions — all of which are real questions I’ve been asked in the last three months. You’ll get explanations and answers to help you stay sharp and confident.
What Are The Most Important React Interview Questions?
React interviews AREN’T just about knowing hooks and components! Interviewers easily spot when you’re just memorizing answers (I’ve caught many students doing this).
Here’s the truth — if you’ve never built a React app, start there before interviews. Many developers struggle with basic coding tasks despite knowing the theory perfectly.
You need to show how you’ve used React in ACTUAL projects. Even a simple “todo” app (or something more complex) demonstrates your practical understanding!
My best advice? Build something real before interviewing. It’ll make a bigger difference than memorizing React interview questions. Showing your actual code helps you land the first React job.
Want more? Read “Typescript Interview Questions: 15 Most Recent Questions I Was Asked in 2025“
1 What Is React, And Why Would You Choose It? — React Interview Questions
React (from smart folks at Facebook) is a JavaScript tool for building modern websites. React is a JS library (not a full framework, FYI!) that makes creating interactive UIs (think buttons, forms, etc.) easier and faster.
Think of React as LEGO blocks for websites. You get small, reusable pieces of code — “components” — that snap together to build web elements.
Rect lets you write HTML-like code (thanx to JSX – JavaScript XML) directly in JavaScript. Plus, React uses a one-way data flow — data moves in one direction, making debugging less of a headache.
When answering React interview questions, focus on its strengths. First, mention components. Second, talk about the speed, reusability, and easy debugging. Third, discuss the huge community (over 40% of web developers use React).
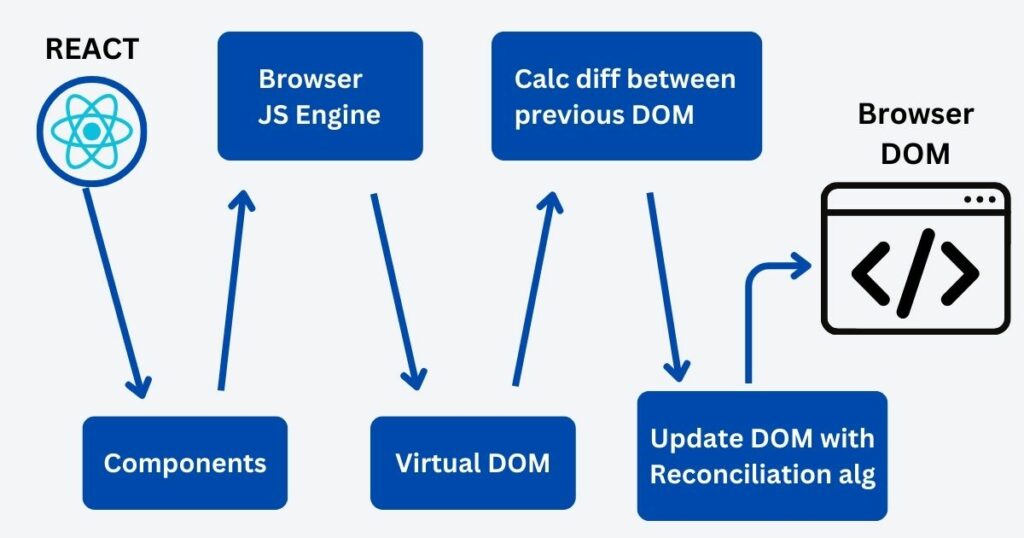
2 What Is The Virtual DOM, And How Does It Help React?
Virtual DOM (or “V-DOM”) is a lightweight copy of the real DOM that React uses to make updates faster.
When you make changes to your app (like clicking a button), React creates a new Virtual DOM and compares it to the old one (“diffing”). Only the changed parts get updated (“reconciliation”) in the real DOM—this saves time and energy!!
Explain that the Virtual DOM is like a “blueprint” or a “draft.” Mention that it’s NOT actually faster than the real DOM. The magic happens when React uses this “blueprint” to make smart updates.
My fav explanation is: “The Virtual DOM helps React plan efficient changes before making them.”
Don’t forget to mention “reconciliation” and “diffing” — Interviewers eat that up!
3 What Is State In React, And How Is It Different From Props?
State makes your React app interactive!
State is React’s notepad. When you write something new on it, React notices and updates the screen. When state changes, the component updates automatically
State changes make components re-render (that’s the whole point).
State lives inside the component. A good rule of thumb is to keep state local and use useState hook to change it.
Props are like mail that comes from outside — from the parent component. Props stay the same unless the parent updates them.
Props are read-only. The difference? State can change (you control it), but props can’t (they’re set in stone).
When explaining state vs. props, say state is a component’s memory, and props are like labels.
4 How Do You Handle State In React? When Should You Lift State Up? How Does State Management Work In React?
State management with useState
hooks in React works in two main ways:
- Local State: Lives inside one component
- Lifted State: Shared between components through a parent
Let’s say you’ve got a Parent component that controls the count
state. The Child component gets the count
value and a function to update it. The Child displays the count but cannot change it directly. Instead, it calls a function (passed from the Parent) to update the count
.
function Parent() { const [count, setCount] = useState(0); return <Child count={count} onIncrease={() => setCount(count + 1)} />; } function Child({ count, onIncrease }) { return <button onClick={onIncrease}>Clicked {count} times</button>; }
When you need to share state between components, you “lift state up” to their closest common parent. Then, you pass it down as a prop — just a way to send data to another component.
State belongs where it is needed the most. Only lift state up when multiple components need to share it
For bigger apps, state in the wrong place will lead you to problems. If you put it too high, you cause unnecessary re-renders that slow things down. Use global state management tools like Context API or Redux to share state without passing props.
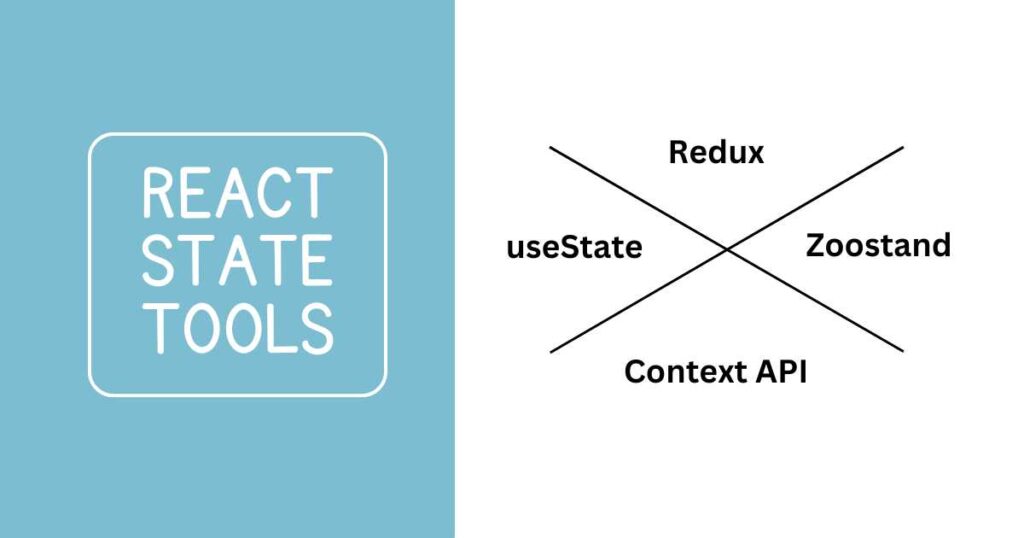
5 What Is The Purpose Of Redux Or Other State Management Tools In React? When And Why Do You Use Redux? Can You Walk Through a Basic Redux Flow Step By Step?
State management tools (Redux is the big name) keep your React app organized. These tools (Redux, Zustand, Recoil, and many others) track state in React apps. Instead of passing props everywhere, Redux stores state in one place (the store).
React’s built-in state is great (for small apps), but once your project grows, managing state gets messy real fast. Suddenly, data is all over the place, and tracking changes feel like a nightmare.
Redux is a predictable state container for JavaScript apps. It helps store, manage, and update state.
The Redux flow starts by calling a store.dispatch()
and passing in an action object that describes what changes should happen. Reducers take those actions and update the state. The latest state is then pulled into components using selectors and stored.getState()
.
Redux basic flow:
- Store – Holds the global state.
- Dispatch – Dispatches an action to change the store.
- Action – A plain JavaScript object with a
type
field. - Reducer – A pure function takes old state + action → returns new state.
- Selector – Grab specific state data.
Using Redux makes sense when your app has a lot of shared state. It keeps everything organized and predictable. But for smaller apps, Redux might be too much. To only avoid props drilling, Context.API is a better choice
6 How Does Redux Middleware Work? What Is The Purpose Of Redux-Thunk And Redux-Saga?
Middleware in Redux acts as a bridge between the Actions and the Reducers. It manages side effects (like API calls) before the action reaches the reducer. Out of the box, Redux can only handle synchronous actions.
Middleware intercepts actions before they hit the reducer
Redux-Thunk allows performing asynchronous tasks (resolving Promises) and then dispatching another action when the data is ready. Think of it as delaying (intercepting) actions until they are ready. For most cases, Thunk is great.
Redux-Saga uses generator functions to handle side effects and build complex flows. For complex cases (tons of API calls or background tasks), Saga is the better.
If you don’t use middleware, async logic ends up inside components, making them hard to read/manage.
In interviews, explain middleware’s benefits. Walk through how it helps manage side effects (mention both Thunk and Saga), and share a practical example.
7 Can You Explain Context API In React And When You Might Use It?
React introduced Context API in React 16.3 to fix the “prop drilling” issues. With Context, you store state in a central place, outside the component. Then, any component (no matter how deep) can grab it.
Context API is NOT a state management tool. It doesn’t handle state updates like Redux. Instead, Context API is a dependency injection library that just provides data to components.
Context API VS State management tool
- Context API does not manage state logic.
- Context itself doesn’t have built-in tools for updating state — no reducers or middleware.
- Every time Context updates, all components using it re-render
If you need real state management, Redux (or Zustand) is better. Context API is best to avoid props drilling.
8 Can You Walk Through A Simple Example Of Using React Router For Navigation?
React Router allows users to move between pages by swapping out React components.
React Router handles navigation without reloads and makes single-page apps feel like multi-page apps!
First, we need to add React Router to our project and wrap your app with <BrowserRouter> component.
Then, set up <Routes> for your pages (like Home, About, or Contact). <Routes> map URLs to components so each <Route> shows a different React component.
Use <Links> instead of regular <a> anchor tags. Create <Links> to different pages in your app. These links don’t reload the whole page, which makes websites feel faster.
import { BrowserRouter, Routes, Route, Link } from 'react-router-dom'; function App() { return ( <BrowserRouter> <nav> <Link to="/">Home</Link> <Link to="/about">About</Link> </nav> <Routes> <Route path="/" element={<Home />} /> <Route path="/about" element={<About />} /> </Routes> </BrowserRouter> ); }
The navigate() function provides automatic page changes. For example, you can send users straight to their dashboard after someone logs in!!
Answering React interview questions, explain the main parts. Talk about <BrowserRouter> (the wrapper), <Links> (the navigation buttons), and <Routes> (the content switcher).
Mention that React Router improves performance and user experience (UX). Talk about how it helps with bookmarking pages and keeping browser history working properly.
9 How Do You Fetch Data In A React Application? Integrate 3rd Party API? How do you handle errors during data fetching in React?
Calling the API in React is simple. You send a request, get the response, and update the UI.
React devs often use the useEffect
hook for basic cases. Fetch API
or Axios
fetch data, and we put the result in the component’s state.
To integrate a 3rd-party API:
- Obtain API Credentials (API key, store it securely in
.env
or AWS Secret Manager) - Make API Requests (
fetch
oraxios
insideuseEffect
) - Handle Responses (format data, update state)
- Manage Loading and Errors (provide UI feedback with pop-ups, spinners or messages)
- Optimize Performance (cache, paginate, avoid redundant API calls)
Handling errors properly is key. Wrapping API calls in a try/catch
block helps prevent crashes. Always check if the request was successful before using the data. Common API errors include:
- Network issues (user is offline, slow connection, API server down)
- Invalid responses (incorrect data format, missing fields)
- Rate limits (too many requests)
- Unauthorized access (invalid or missing API keys, expired tokens)
When testing, use mock APIs. Mocking lets you test different scenarios: slow responses, missing data, or even API failures.
Having one custom hook — “useFetchData” — to make data fetching reusable. This abstract reusable hook handles fetching, error handling, and loading states across multiple components.
Use caching tools like React Query to speed up repeated requests and avoid dedups for better performance. React Query saves previous data and re-fetch only when needed!! Always clean up your useEffect
to avoid memory leaks.
Interviewers also love hearing about performance boosts (like debouncing inputs, caching, lazy loading, and using API pagination).
10 How Do You Optimize Performance In A React Application? — React interview questions
To optimize a React app, follow these:
- Centralize State: Move shared state to a global store (Redux, Zustand, Context API).
- Avoid Re-renders: Use
React.memo
oruseMemo()
to prevent unnecessary re-renders. - Lazy Load: Split code into chunks with
React.lazy()
. - Virtualize Lists: Use libraries like
react-window
for large lists.
When components re-render too much, things slow down. React has built-in tools (Memo
, useMemo()
, useCallback()
) that stop components from updating when they don’t need to.
Another “must” is lazy loading with React.lazy()
and <Suspense>
. Instead of loading everything at once, React loads parts of the app when the user actually needs them.
Large lists also pop up performance issues, like choppy scrolling. Virtualization is a trick that only loads visible parts of a list — not thousands of hidden items.
Read more about “UI UX Optimization: 9 Proven Strategies That Actually Work in 2025“
Always use React DevTools to find performance bottlenecks
11 How Do You Handle Forms In React? What Is Your Fav Option?
I prefer using controlled components for my basic forms. These forms use state to store input values. When you type in a field, React updates the state, and the input reflects the new value. You always know what’s happening and can validate input as the user types.
import { useState } from "react"; function MyForm() { const [name, setName] = useState(""); return ( <form> <input value={name} onChange={(e) => setName(e.target.value)} /> // Controlled input field <button type="submit">Submit</button> </form> ); }
For complex forms, libraries like Formik or React Hook Form make life way easier. They handle state, validation, and even form submission!! React Hook Form is lightweight and easy to use, but Formik is great for bigger apps!!
import { Formik, Form, Field } from "formik"; <Formik initialValues={{ name: "" }} onSubmit={values => console.log(values)}> <Form> <Field name="name" type="text" /> <button type="submit">Submit</button> </Form> </Formik>
Always validate your inputs before submission. For example, check for empty fields or invalid data types. If you’re building a login form, check if the username
and password
fields are filled out. If not, show an error message.
On React interview questions about forms:
- Explain controlled components (how they sync state with inputs).
- Talk about validation and error handling.
- Mention tools or libraries (Formik or React Hook Form).
12 How Do You Deal With Error Boundaries In In React? — React interview questions
Error boundaries prevent an entire app from crashing. They catch errors inside React components and display a fallback UI instead.
The easiest way to handle this is by using libraries like react-error-boundary
. It wraps them inside higher-order components to provide error boundaries.
Provide a fallback UI when using error boundaries. Show a message, a button to refresh, or something helpful. Log errors with services like Sentry or LogRocket.
Error boundaries work for render errors but not async errors (like API calls failing) or event handlers. You need additional error handling to handle those.
13 How Do You Handle Authentication In A React App? — React interview questions
One way to authenticate is using JSON Web Token (JWT). When a user logs in, the BE server creates a token and sends it back to FE.
The React apps store JWT tokens usually in:
- localStorage — Easy to use, vulnerable to XSS attacks.
- sessionStorage — requiring users to log in again after closing the browser, still vulnerable to XSS attacks.
- Cookies — most secure options when using HTTP-only, Secure, and SameSite attributes.
Every time the user makes an HTTP request, the FE app includes the token to prove the user is legit. The BE server checks the token and decides if the request is allowed.
Send tokens only over HTTPS
and use cookies with HTTP-only
and Secure
flags, which means JavaScript can’t access it (prevents XSS attacks).
14 What Are Common/Best Ways To Style React Components? What Are Your Recommendations To Style React App? Why?
React doesn’t come with built-in styling rules. You gotta pick a way to style your components, and there are a bunch of options.
Inline styles
Inline styles are easy but kinda bad. Inline styles mean putting styles right inside the style
prop of a React component. They don’t support pseudo-classes (:hover
or :focus
), and they can make your JSX a mess.
Pros
- Simple
- Works anywhere
- No extra setup
Cons
- No pseudo-classes (
:hover
,:focus
) - Bad performance
- Messy JSX
jsx
function App() { return ( <button style={{ background: "blue", color: "white", padding: "10px" }}> Click Me </button> ); }
Inline styles are good for small tweaks but not for large projects. They make HTML look messy and harder to keep CSS styles consistent across a site.
Plain CSS
The classic plain old CSS in a .css
file is simple but has issues. Styles can clash, so most devs no longer use plain CSS alone.
Pros
- Simple
- Works anywhere
- No extra setup
Cons
- Styles can clash
- No scoping
- Hard to scale in big apps
- Name collisions
/* styles.css */ .button { background: blue; color: white; padding: 10px; }
import "./styles.css"; function App() { return <button className="button">Click Me</button>; }
CSS works well for small websites or simple pages. But when sites grow, plain CSS becomes difficult to manage. You have to repeat yourself a lot, and it’s easy to forget where a rule is.
CSS Modules
CSS Modules scope styles to a specific .module.css file, so you avoid global conflicts. Use it for medium-sized projects, but it gets messy in bigger apps.
Pros
- No style clashes
- Works with SASS/LESS
- Great for small/medium projects
Cons
- More to set up
- More boilerplate
- Harder to override styles
/* Button.module.css */ .button { background: blue; color: white; padding: 10px; }
import styles from "./Button.module.css"; // Import as a module const Button = () => { return <button className={styles.button}>Click me</button>; }; export default Button;
CSS modules don’t leak into other parts of the page. But it requires a build process, a bit harder to start using.
SASS
SASS (or SCSS) is one of the most popular CSS preprocessors. SASS and LESS add variables, nesting, functions and mixins to CSS. These make styles more reusable and easier to organize.
Pros
- Variables, loops, functions, mixins.
- Nesting
- Partials and Imports
- A mature ecosystem
Cons
- Requires a preprocessor
- Can lead to bloated CSS
- Learning Curve
- Seperate styling files
SASS helps keep things clean in big projects. However, it needs a compiler to compile .scss to regular .css before a browser can use it.
$button-bg: blue; $button-color: white; .button { background-color: $button-bg; color: $button-color; padding: 10px; }
import './Button.scss'; function SASSButton() { return <button className="button">Click Me</button>; }
SASS saves time in big projects. It makes CSS more powerful and reusable. It helps developers write cleaner and shorter code.
Styled-components
Styled-components — a CSS-in-JS library — is super popular. It lets you write CSS inside JavaScript files using template literals. It’s great for component-based styles but slows things down if overused.
Pros
- Scoped styles (no clashes)
- Supports dynamic styles – easier to override styles
- Easy to maintain
Cons
- Slow for large apps
- Extra dependencies
import styled from "styled-components"; const Button = styled.button` background: blue; color: white; padding: 10px; `; function App() { return <Button>Click Me</Button>; }
Styled-components keep styles close to the components they belong to. It makes it easy to use JS logic inside styles. Some may dislike it because it can slow down performance at runtime.
Tailwind
Tailwind is a utility-first CSS framework. Instead of writing custom CSS, you use pre-made classes, like bg-blue-500
. It’s great for fast development.
Pros
- Provides pre-designed classes:
flex
,grid
,p-4
,text-center
- No separate stylesheets
- Highly customizable via
tailwind.config.js
- Purges unused classes
Cons
- Takes time to learn the utility classes
- JSX can become cluttered
className="px-4 py-2 bg-blue-500 text-white"
function TailwindButton() { return ( <button className="bg-blue-500 text-white py-2 px-4 hover:bg-blue-700"> Click Me </button> ); }
Many developers like Tailwind because it’s flexible. It also reduces the need for extra stylesheets. But it has a learning curve.
Material-UI (MUI)
MUI is a popular React UI framework based on Google’s Material Design principles. It provides a set of pre-built components with built-in styling.
Pros
- Highly customizable
- Follows Google Material Design principles
- Over 50 well-structured UI components
- Uses Emotion by default
Cons
- Uniform look
- Extra dependencies
- Hard to override default styles
import React from 'react'; import Button from '@mui/material/Button'; function MUIButton() { return ( <Button variant="contained" color="primary"> Click Me </Button> ); }
Material-UI is modern and consistent. But it adds a lot of extra code. Some developers find it hard to customize.
15 How Do You Test A React Component?
Testing React components means writing tests to simulate how users interact with your app. Four tools stand out — Jest, Vitest, RTL, and Cypress.
Jest is fast, well-supported, and has everything: mocking, spying, snapshots. It is a bit slow, but hey, so is quality work!!
Vitest is the faster alternative to Jest. Built for Vite, it’s super smooth. Vitest has built-in TypeScript support and ESM comtatibility.
React Testing Library (RTL) focuses on interactions and integration tests. RTL runs tests in a simulated DOM. It is fast and it’s perfect for quick feedback and TDD.
Cypress is the REAL browser-based tester. It runs tests inside an actual browser, catching real-world bugs. It clicks, types, scrolls — just like a user would. Cypress is slower) than RTL because it loads the entire app in a browser and runs REAL interactions.
For the best testing setup, use a mix of all four: Jest or Vitest for unit tests, RTL for component behavior, and Cypress for full app/end-to-end testing.
Conclusion: Essential React Interview Questions
The React ecosystem keeps evolving, so stay curious and keep learning.
Practice explaining these concepts in simple terms. Be ready to discuss your experience with React. And don’t stress if you don’t know everything.