Svelte vs. React Javascript—this is going to be epic! I’ll explore two powerful JavaScript tools for building user interfaces (UI).
In this post, I show their syntax, state management, reactivity, and other features.
You will find trending charts, comparison tables, code examples, and helpful infographics.
Need to choose between React and Svelte? I can help you with it!
What To Look At To Compare Differences Between Svelte vs React
Several key factors come into play when I compare Svelte and React for the next projects. The learning curve, performance, and overall place in the web development world (including community and job market) are the elements that most impact my decision.
Both Svelte and React have relatively low entry barriers, especially for developers familiar with JavaScript.
With its JSX syntax and component-based architecture, React might feel more like a traditional web development practice.
However, Svelte lets you write less code (most of the time)! Its reactive syntax and compiler “magic” allow you to write components with much less boilerplate. And no need for complex state management setups!
While React rerenders EVERYTHING and then compares at runtime to see what has changed, Svelte’s compiler generates optimized vanilla JavaScript at build time. This results in smaller bundle sizes and better runtime performance than React.
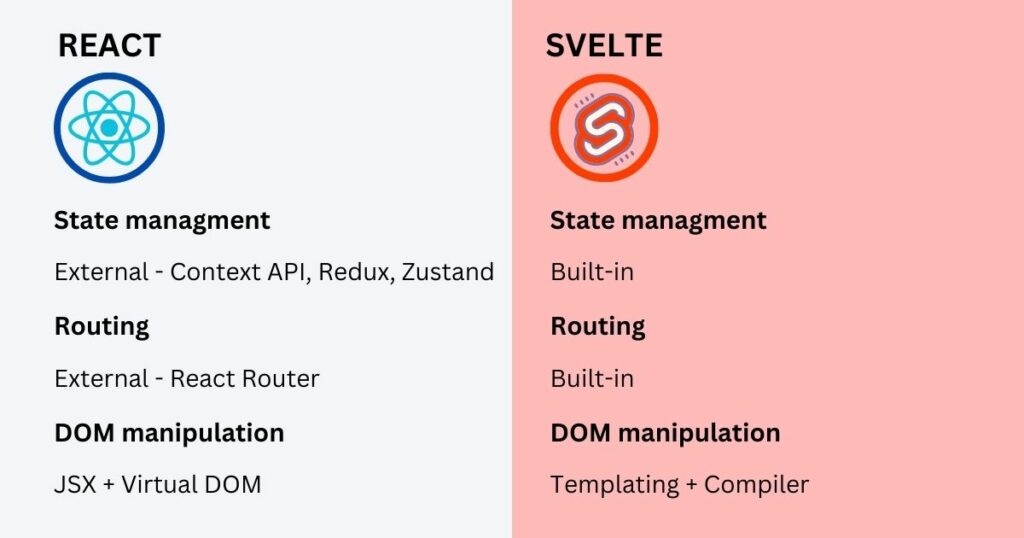
Svelte has a rapidly growing community and ecosystem. But at this moment, React’s popularity in the web development niche has made it a standard choice for many developers and companies.
As you can see, each side has pros and cons. Let’s examine them in more detail!
A Brief Overview Of Svelte and React
Svelte and React are popular JavaScript tools for building user interfaces (UI) in Web Development.
React was first introduced by Jordan Walke, a software engineer at Facebook, in 2013. It was later open-sourced in May 2013.
On the other hand, Rich Harris released Svelte in November 2016. Svelte was designed to address common pain points in front-end development that other frameworks, including React, struggle with.
What Is Svelte Javascript Framework
Svelte is a modern JavaScript framework other than the React or Vue paradigm. Instead of interpreting code at runtime, Svelte shifts much of the work to compile time.
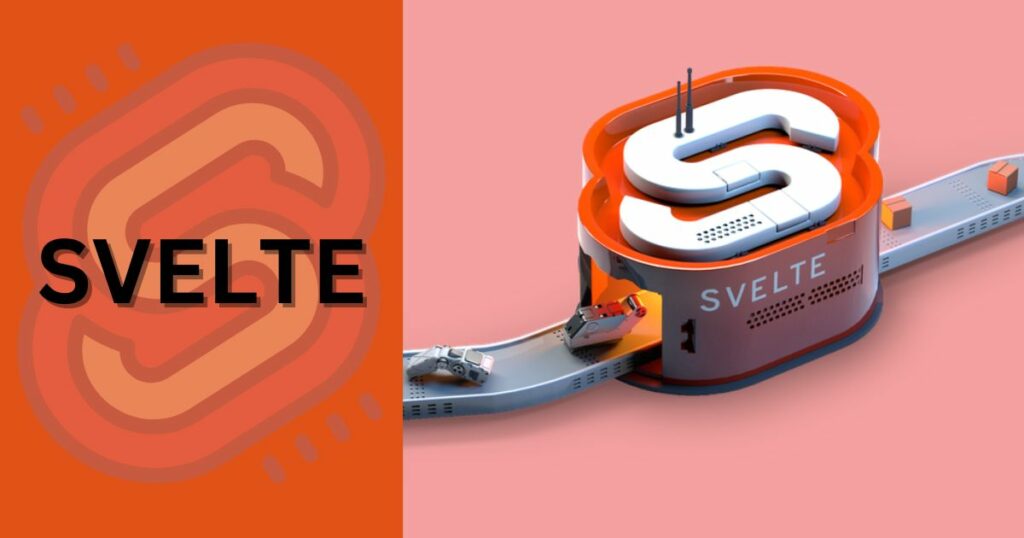
While compiling, Svelte converts your app into ideal JavaScript! Unlike React, it doesn’t need a virtual DOM to compare the current and updated UI states. Instead, a compiler manipulates the DOM.
Svelte aims to be simple and approachable, and its syntax is clean and intuitive. Svelte has a more straightforward API and requires less boilerplate code than most FE libraries.
Svelte Compiler
The Svelte compiler is at the core of what makes Svelte a unique framework. It analyzes your components and provides JavaScript code that directly manipulates the DOM.
During the compilation process, the compiler generates code that only includes the necessary functionality. No runtime overhead! No extra live computation!
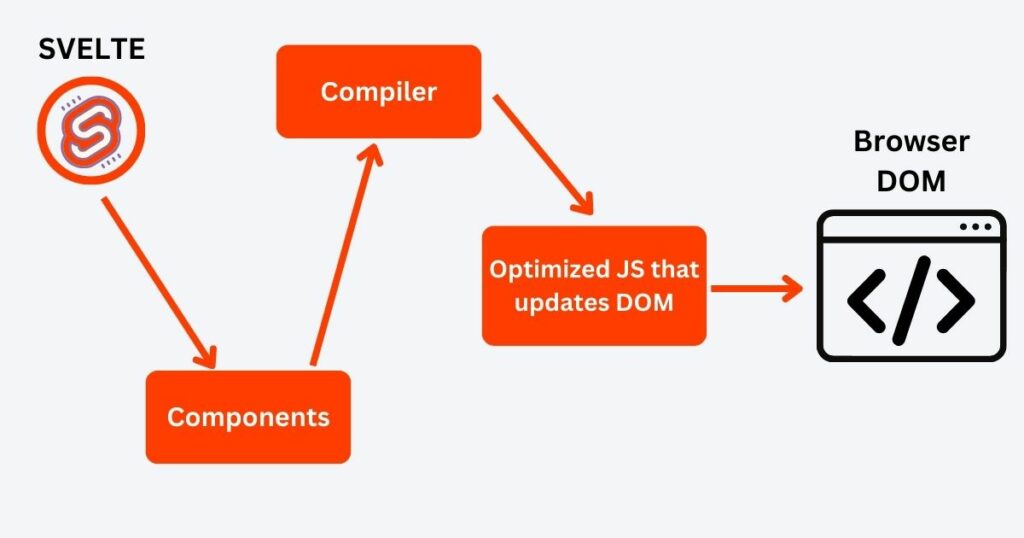
Svelte compiles your code into vanilla JavaScript BEFORE the page is rendered, reducing the overall size of your bundles!
The framework abstracts much compilation complexity, which is generally good practice (encapsulation is good!). However, this can make it challenging to trace issues back to their source and requires more effort in debugging.
Svelte optimizes your code during compilation, resulting in JavaScript code that behaves not as you may expect.
Anyway, I think the compilation magic process outweighs the debugging occasions, lol!
Svelte Reactivity
The reactive design pattern is at the heart of the Svelte framework.
Reactivity allows you to create variables and expressions that automatically update when their dependencies change. Define a variable based on another variable, and if the original variable changes — Svelte will update the dependent variable automatically!
To get this reactivity, use the Svelte $: syntax reactive declaration.
<script> let count = 5; $: doubledCount = count * 2; </script> <p>{doubledCount}</p>
I created a count and a doubledCount reactive variable. doubledCount will automatically update whenever the count changes to reflect the new value.
The most popular reactive usecase — create a state (reactive) variable, display it on UI, and the compiler will automatically update it on UI and keep your data in sync.
Svelte’s reactivity pattern also applies to component props and stores. For example, I can share the state between components, and Svelte updates it everywhere the components use the state.
When a value changes in a Svelte component, the framework traces its dependencies and updates (propagates) only the affected parts of the DOM. This avoids unnecessary re-renders and leads to more efficient applications.
Svelte Pros And Cons Of Svelte
Svelte Pros
Simplicity | Simple and intuitive building approach — easy for developers to learn and use! |
Performance | Compile your components to fast and pure vanilla JavaScript! Svelte provides excellent performance with small bundle sizes and fast runtime! |
Reactivity | Simple state management! Create dynamic UIs with less boilerplate code! |
No Virtual DOM | No need for a virtual DOM! With the compiler, Svelte code results in faster initial load times and more efficient updates. |
Svelte Cons
Ecosystem Maturity | Still evolving tooling... In some cases, this leads to limitations or functionality gaps. |
Community Support | Relatively small community. As a result, we have fewer resources and support options. |
Debugging | Debugging can be challenging because of the compilation. Even with Svelte debugging tools, you may spend some extra time and effort resolving issues. |
When Use Svelte
Use Svelte for applications where performance is a top priority! The compiler speeds up the load times and improves runtime performance.
A reactivity pattern provides a smooth and responsive user experience (UX). The small bundle size makes it deliverable over low-bandwidth networks or on devices with limited resources!
Svelte is a solid choice for prototyping and rapid development! Focus on building features rather than managing state and updates. And no need for tons of boilerplate code!
I find Svelte powerful enough to build a proof of concept or minimum viable product (MVP). I use it when exploring new design concepts or validating a product idea.
While many devs use Svelte for large-scale applications, I noticed it well suits small to medium-sized projects. The kind of projects that can fully leverage Svelte’s simplicity and performance without the overhead of more complex frameworks.
What is React JS Library
React JS is an open-source JavaScript library for building user interfaces (UIs) for single-page web applications. It was developed by Facebook and maintained by a community of individual developers.
I love React for creating reusable UI components! I encapsulate the structure and compose them together to build complex UIs!
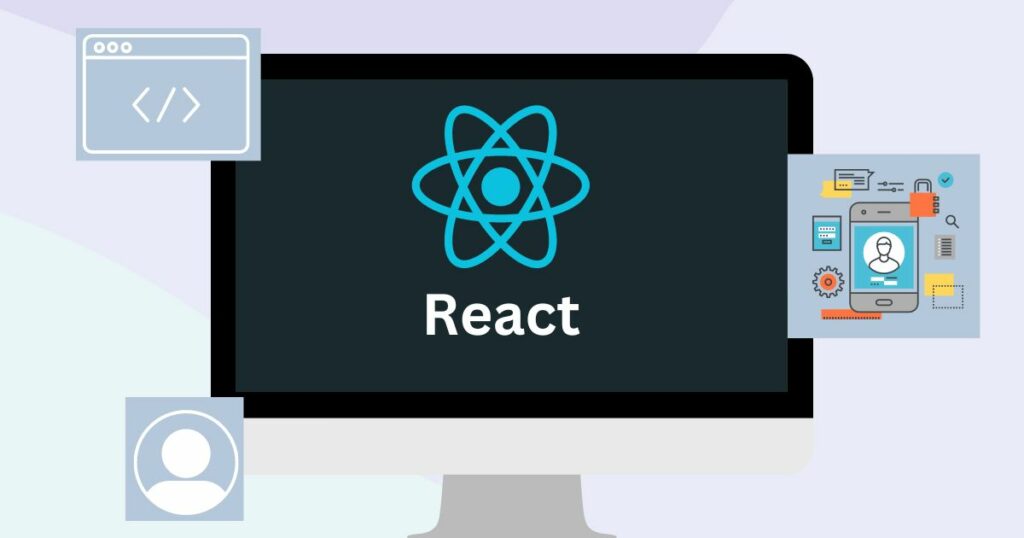
React is a JavaScript-only library that uses only JavaScript inside its core. It does NOT introduce its own templating languages! Instead, React uses JSX, a JavaScript extension that allows you to write HTML-like code directly in your JavaScript files.
You can still use a React component from 10 years ago in a modern SSR (server-side rendering) app! This compatibility is one of the reasons why React is so popular, as it saves code refactoring time! However, it also stifles improvements in the framework… and worsens the developer experience (DX)…
React can be challenging due to its complex API and additional tools like JSX or virtual DOM. However, React’s design patterns allow developers to create highly complex UI.
React app and Virtual DOM
Virtual DOM (Document Object Model) is an in-memory representation of the actual DOM.
Operations with actual DOM are computationally expensive due to the way browsers reflow (calculating the positions and sizes of elements) and repaint the page. Especially on complex web pages!
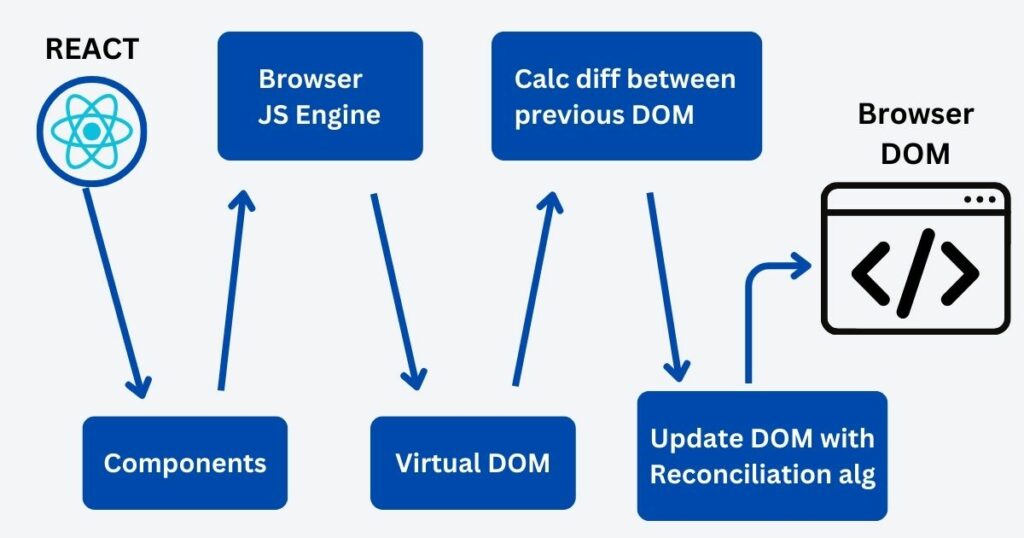
React uses Virtual DOM to update the UI, reducing the number of costly actual DOM operations.
Initially, this idea seemed like a breakthrough, and React heavily relied on its virtual DOM to update the actual DOM. However, as applications grew more complex, React had to introduce additional layers of complexity to address performance issues.
The downside is that virtual DOM rerenders EVERYTHING on each update and then compares it against the previous version. Plus, the virtual DOM operates during runtime — additional computational overhead!
useMemo() and useCallback() hooks were the React answers, but both require developers to manage them carefully! For me, it took a quite while to figure out how to use them properly, lol!
React and JSX
JSX (JavaScript XML) is another React feature that allows you to write HTML-like code directly within your JavaScript files.
I believe that JSX is the best way to design layouts!
A standard way to create a simple div in React is
React.createElement('div,' null, 'Hello, world!').
JSX transformed this to
<div>Hello, world!</div>
and then tools like Babel or SWC transform this code into regular JavaScript to run in the browser.
JSX stands out as a key feature (No doubt here!) that puts React at the top! It is flexible, easy to write and read, and powerful for dynamic values and expressions.
React Pros And Cons Of React
React Pros
JSX | A syntax that blends HTML — easier to write and visualize UI components! |
Large Ecosystem | The largest ecosystem (probably) of libraries, tools, and community support! You'll find a component (or ten, lol!) for any common problems! |
JavaScript-only Library | No unique templating language! Everything is written in JavaScript, so learning React is essentially learning JavaScript. |
React Cons
Steep Learning Curve | React is challenging to learn, especially for new JavaScript or Front-End developers. |
Tooling Complexity | Various mandatory tools, such as Webpack, Babel, React-Router, and various global state libraries, add complexity to the development process. |
No Build-In Pure Reactivity | React's reactivity isn't fine-grained (sadly) and affects performance quite a bit!... |
When Use React – Best Use Case
I recommend React for large-scale projects with complex user interfaces. If your app requires many pages or involves complex computations — React can do it!
React makes the large codebase maintainable, and component-based architecture improves collaboration among team members.
Many enterprise-size companies use React for their web applications. Facebook, Instagram, Netflix, Airbnb, and Uber chose React for their large-scale applications.
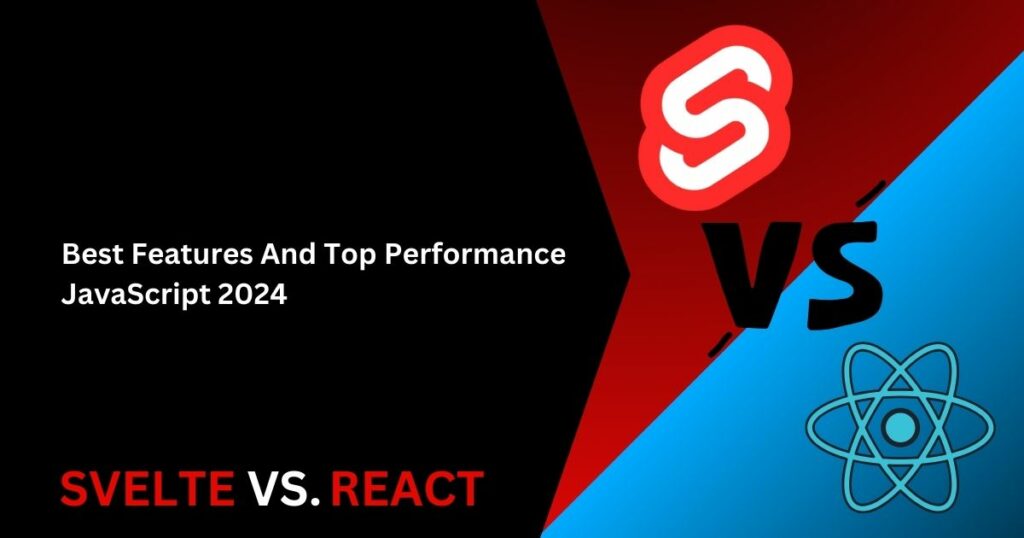
Detailed Comparison Differences Between Svelte And React For Developer
Svelte and React stand out as two popular web dev tools with their own strengths and approaches to building user interfaces.
React has established a JavaScript ecosystem, while Svelte is a game changer with its innovative compiler-based approach.
Seeking the ideal framework for your project? Explore the differences between Svelte and React in performance, development speed, mobile app development capabilities, and code structure.
Performance: Svelte vs. React
Svelte and React take different approaches and cater to different priorities.
React’s virtual DOM proved a way to update the actual DOM with minimal changes. While the virtual DOM is effective for many applications (especially those with complex UIs), it brought performance overhead (particularly in apps with frequent updates).
Compare Performance
Svelte | React | |
Consistently interactive - a pessimistic TTI | 1,877 | 2,477 |
Script boot time - the ms required to parse/compile/evaluate | 16,0 | 65,9 |
Main thread work cost - the amount of time spent on main thread | 308,5 | 431,3 |
Total byte weight - network transfer cost | 149,7 | 251,9 |
Svelte’s compiler approach speeds up the runtime. It reduces the amount of runtime work by shifting the heavy lifting to the build step. Svelte code manipulates the DOM directly, improving runtime performance.
Development Speed: React and Svelte
Both packages have features letting you build and iterate on web applications.
React’s component-based architecture and ecosystem help you build any application you can imagine. The largest community provides resources and support 24 hours!
JSX syntax is even more important than the ecosystem. Try it once — you’ll stick to it for many years (just like I did).
On the other hand, Svelte’s simplified syntax and reactive pattern lead to faster initial setup and prototyping. You need much less boilerplate code to implement similar functionalities compared to React. This results in faster development times, especially for smaller projects.
Mobile App Development with Svelte and React Native
React Native is a popular framework for building native mobile. I write pure JS code with React and deploy it across iOS and Android platforms.
Reusing the same components, routing, and dependencies in React Native apps is a massive plus for me!
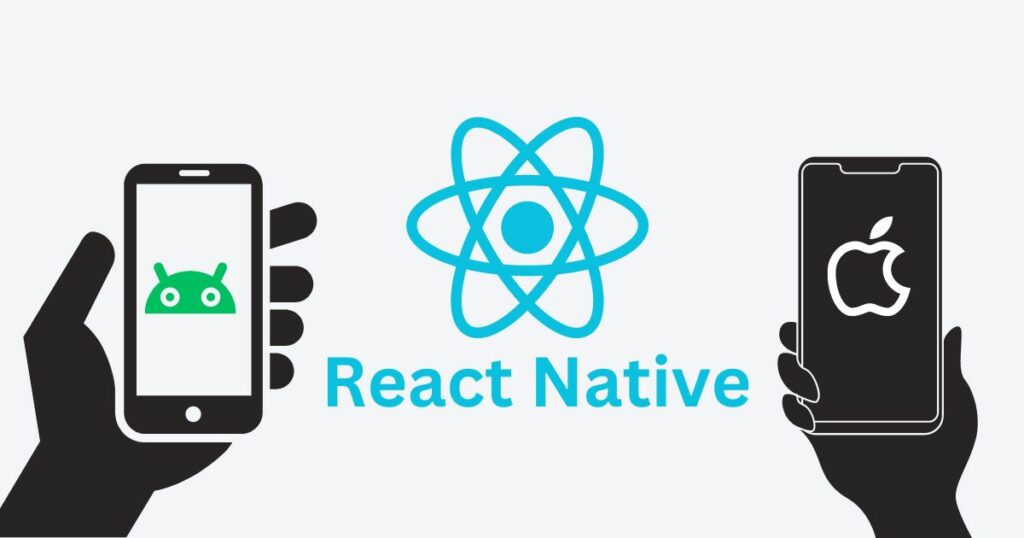
React can target mobile, desktop, and web platforms with a single codebase! Sometimes, you’d need to duplicate the code « if mobile – render this, if web – render that,» but it’s rare.
Svelte does not have built-in support for mobile development and was never designed for native applications. It does not offer the same level of support and tooling as React Native.
React Component vs Svelte Component
React and Svelte both use a component-based architecture, but there are some differences in how components are defined and used.
Svelte’s reactive blocks are often easier to use than React’s hooks. There is no need to manage dependencies manually — reactive pattern simplifies writing and maintaining code.
However, you can’t create Svelte components via pure JavaScript — they must be defined in a .svelte file! In my opinion, this is the biggest downside of Svelte!
Another limitation is that only one component is allowed per .svelte file, causing issues in some cases.
React does not limit the number of components in a single file. Create as many as you need and organize the maintainable codebase.
You can create generic and polymorphic components with React since React components are essentially JavaScript functions. Create generic and polymorphic components easily, for free, and right out of the box!
TypeSctipt Support: React or Svelte?
Both React and Svelte have good support for TypeScript, but React seems to have a deeper/better integration (In my opinion).
Svelte requires some additional TS configuration compared to React.
One limitation in Svelte is that I can use types only within the script tag. You can declare and initialize variables in the script tag and reference them in the markup. But this can lead to unnecessary code fragmentation.
Some operations (for example, complex types mapping) look cleaner in React than in Svelte.
React has excellent support for TypeScript. It comes with official TypeScript definitions for React and React DOM. From the start, you get the type checking for all React components, props, and states!!
Community and Ecosystem: React vs Svelte
React has a mature ecosystem with a large community of developers! Facebook still supports it, delivering regular updates and improvements. We have tonnes of online forums, meetups, and conferences with an active and supportive community!
On the other hand, the Svelte core team backs Svelte’s community. While it is not as extensive as React, Svelte ecosystem is gaining popularity!
Let’s examine the numbers. Svelte’s usage has almost tripled in just three years from 8 to 21 percent of usage.
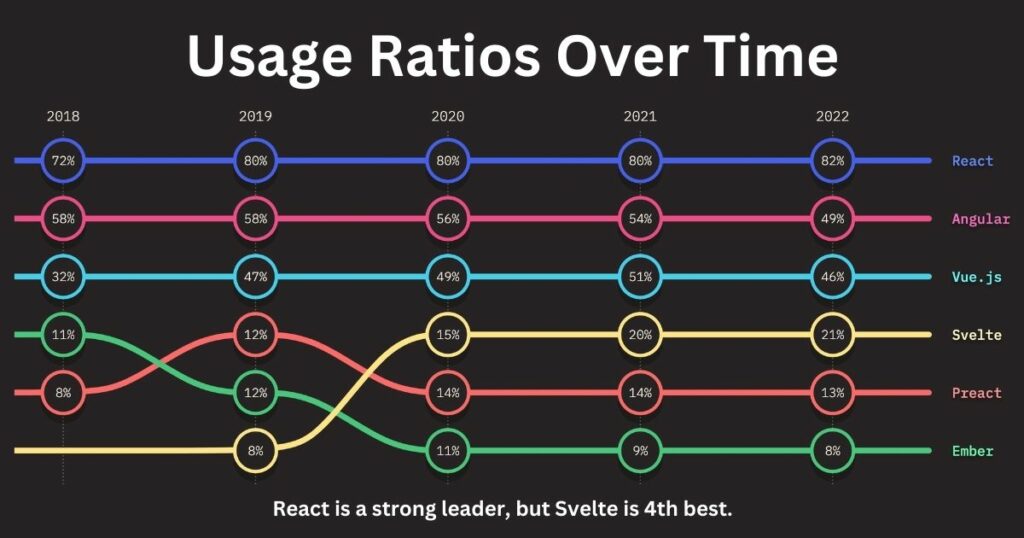
However, React has been a strong leader for the past eight years. It remains a top library and is my personal choice as well!
Web Development And Job Market: Svelte and React
React is downloaded on average 20 times more than Svelte.
While Svelte’s download numbers keep growing, they are still significantly lower than React’s.
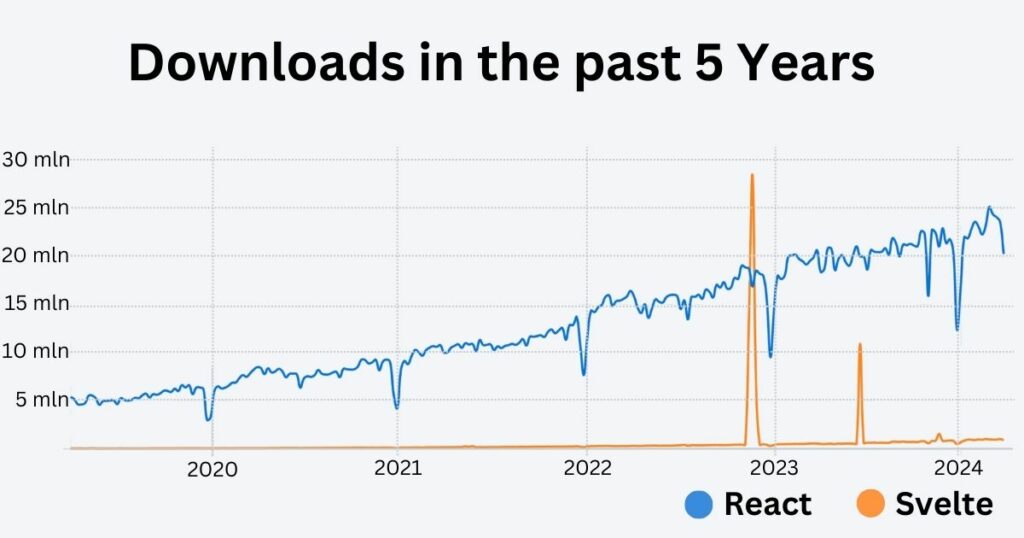
The demand for React developers is significantly higher, resulting in a more extensive candidate pool. It’s easier for companies to find React developers, but there is also more competition, meaning higher salaries for experienced developers!
React = job = money = food
Another research from StackOverFlow also ranked React first, while Svelte is in fifth place among JS libraries like React, jQuery, Angular, and Vue.
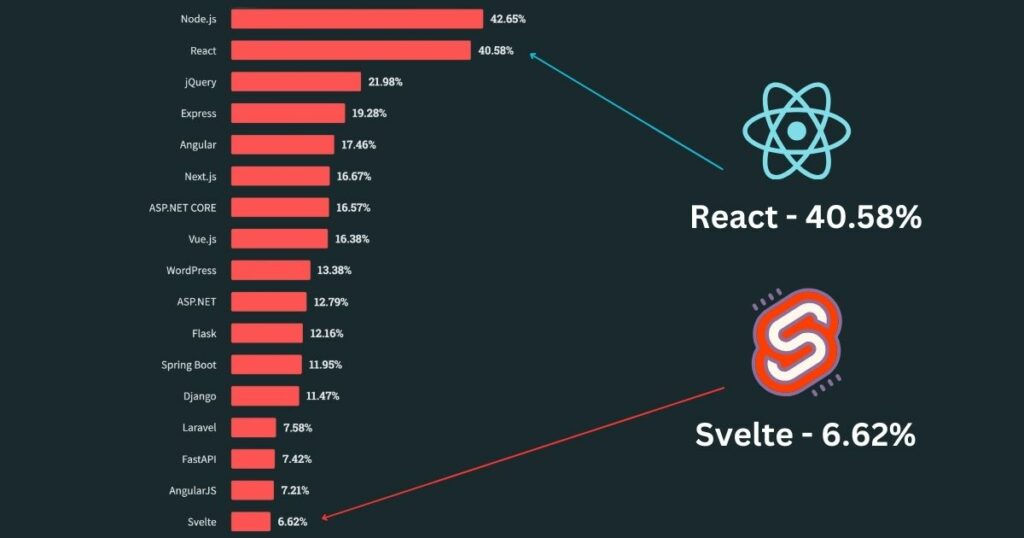
Despite being lower, Svelte’s presence in the top ten shows its growing popularity! Continuous learning is a key for web developers to stay competitive in the ever-changing tech world.
Code Structure and Syntax: Svelte vs. React
In this section, I’ll show React and Svelte’s code structure and features. Compare their syntax differences to understand how each framework handles processes!
Computed/Reactive State
The state creation in React differs significantly from that in Svelte.
In React, we use the useState hook to define a computed state. For example, here, I create a name state and input component:
const [name, setName] = useState("John"); const input = <input value={name} onChange={(e) => setName(e.target.value)} />;
However, this code will run every time the component is rendered. If the component contains an expensive computation, you’ll have to refactor this code…
…Wrap the function with the useMemo() hook — it will prevent unnecessary reruns!
const input = useMemo( () => <input value={name} onChange={(e) => setName(e.target.value)} />, // Set name as useMemo dependency [name] );
This name variable in array brackets is the useMemmo dependency. Now, React recreates the input only when the name state changes, finally!!
In Svelte, the code is much simpler:
<script> let name = "John"; </script> <input bind:value={name} />
In this example, I directly bound the input value to the name variable! Svelte will update the name variable whenever the input value changes — very simple!
Props
I have a parent component that passes a name prop to a child component (Greet).
import Greet from './Greet'; const App = () => { const name = "Alice"; return <Greet name={name} />; };
In React, we pass props to a child as function arguments. Then, we can restructure and display it like so:
const Greet = (props) => { const { name } = props; return <h1>Hello, {name}!</h1>; }; export default Greet;
Svelte templating looks different! You need to put the export keyword to each variable so that Svelte can pass it in from the parent:
// Greet.svelte file <script> export let name </script> <h1>Helo, {name}</h1> // App.svelte file <script> import Greet from './Greet.svelte' let name="Alice"; </script> <Greet name={name} />
Props usage inside a component is similar for both tools, but Svelte has a nice magic trick (syntactic sugar) to match props with their names:
// This works in Svelte only and does not work in React <Greet {name} />
Conditional Logic
In React, we use the ternary operator (condition ? true : false) within JSX to implement true-false situation.
const ConditionalComponent = ({ condition }) => ( <div> {condition ? <p>Condition is true</p> : <p>Condition is false</p>} </div> );
On the other hand, Svelte lets me simply write the “{#if} {:else} {/if}” statement, very similar to vanilla JavaScript code:
<script> export let condition; </script> <div> {#if condition} <p>Condition is true</p> {:else} <p>Condition is false</p> {/if} </div>
Passing Children Elements
In Svelte, pass child elements using the <slot> element. You can place any content inside the component’s tags, and Svelte renders it at the location of the <slot> element.
// Parent.svelte file <script> import ChildComponent from './Box.svelte'; </script> <ChildComponent> <p>Child text 1</p> <p>Child text 2</p> </ChildComponent> // ChildComponent.svelte file <div class="box"> <slot /> </div>
In Parent.svelte file, I am importing the Box component and using it in the template. Inside the <ChildComponent>, I put two <p> child elements that Svelte will pass into the ChildComponent file.
The <slot> inside the Box component is a placeholder for the content passed from the parent App component. Svelte inserts the <slot> element in the Box.svelte file when dynamically rendering a page.
Like Svelte, React also lets us pass child components. I wrote the equivalent of Svelte code that renders parent and child components with some content inside the child.
const ParentComponent = () => ( <div> <ChildComponent> <p>Child text 1</p> <p>Child text 2</p> </ChildComponent> </div> ); const ChildComponent = (props) => ( <div> <h1>Children:</h1> {props.children} </div> );
This is how you’d pass the children from the parent in React. React uses props.children as the equivalent of <slot> element in Svelte.
Array Iterations – Loops
We use the map() method in React to iterate over arrays and render components dynamically. It also lets us assign callbacks on each array item.
const numbers = [1, 2, 3, 4, 5]; const NumberList = () => ( <ul> {numbers.map((number) => ( <li key={number}>{number}</li> ))} </ul> );
In Svelte, you can achieve a similar result using the {#each} block.
<script> let numbers = [1, 2, 3, 4, 5]; </script> <ul> {#each numbers as number} <li>{number}</li> {/each} </ul>
In this example, Svelte calls the handleClick function when you click a list item.
Side Effects
The way to create a side-effect in React is to use the useEffect() hook. Put a variable in the dependencies array to make React watch the state change.
const MyComponent = () => { const [input, setInput] = useState(null) useEffect(() => { fetchData() }, [input]); return <div>I call fetchData function on every {input} change</div>; };
React will call the fetchData function every time the input state changes.
Svelte has a reactive statement ($:) that watches the name variable for changes. Whenever the variable changes, the compiler notices it and triggers related code.
<script> let input = ''; $: input, fetchData(); </script> <div>I call fetchData function on every {input} change</div>;
This mechanism differs from React useEffect(). Svelte defines a reactive relationship between the name and the fetchData() and executes it only when the name changes.
I love reactive design patterns for reducing unnecessary calls and keeping the code declarative!!
Lifecycle
The useEffect() hook helps us perform actions when the component mounts, updates, or unmounts. For example, you can show alerts on component mount and unmount stages.
const AlertComponent = () => { useEffect(() => { // React calls this function when the component mounts alert('Component mounted'); return () => { // React calls this function when the component unmounts alert('Component unmounted'); }; }, []); // Empty dependency array means this effect runs only once, on mount return <div>This is an alert component</div>; };
I used the useEffect() hook with an empty dependency array ([]), meaning the effect runs only once — on the component mount.
The return statement in useEffect() defines a cleanup function. React will call it when the component is unmounted — displaying the second alert message.
I can achieve similar effects in Svelte using reactive statements ($:) and onMount() and onDestroy() lifecycle functions.
<script> import { onMount, onDestroy } from 'svelte'; onMount(() => { // Svelte calls this function when the component mounts alert('Component mounted'); }); onDestroy(() => { // Svelte calls this function when the component unmountes alert('Component unmounted'); }); </script> <div>This is an alert component</div>
I define a callback within the onMount function. Svelte will run it when the component mounts, displaying an alert message. Similarly, the onDestroy function runs when Svelte unmounts the component, throwing another alert message.
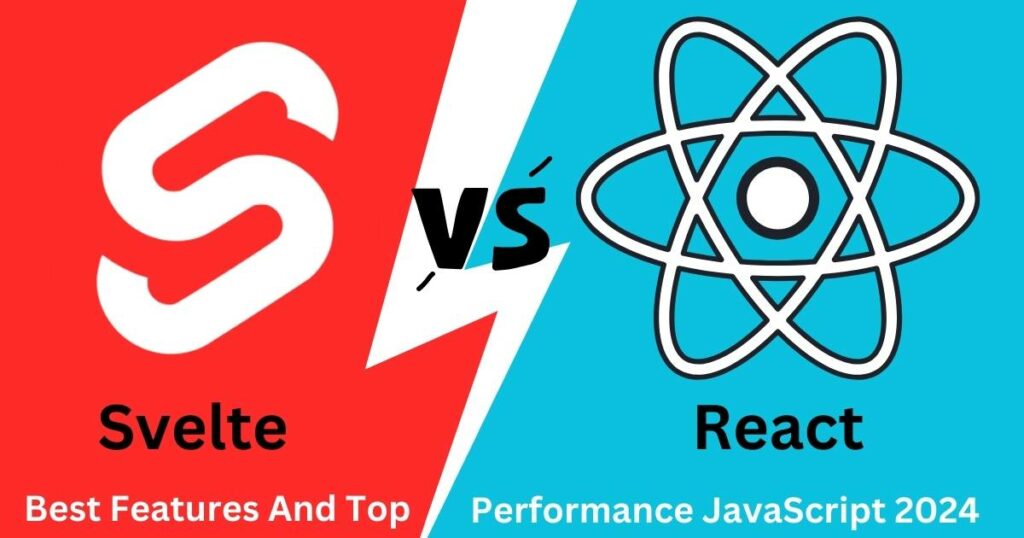
Conclusion: Choosing between Svelte and React
Svelte might be better if you prioritize simplicity, performance, and rapid development.
It reduces boilerplate code and offers excellent runtime performance. However, its ecosystem is still evolving, leading to some limitations…
On the other hand, React has a mature ecosystem, larger community and robust job market demand! I believe React is a reliable choice for large-scale projects with complex UI requirements.
The virtual DOM and JSX syntax provide dynamic user interfaces despite a steeper learning curve.
Both frameworks have their strengths and weaknesses.
Make your choice depending on your project’s needs and your team’s familiarity with the frameworks.