Anywhere near a React project, you’ve heard of Redux vs Context API. These are the tools for handling state across React app components. Some think they do the same thing, but that ain’t exactly true!
I’ve worked with both and know Redux and Context API are NOT twins. They kinda overlap, but they solve the problems in different ways.
Both tools are solid — don’t get me wrong — but they shine in different projects. The trick is knowing when to use which.
I’m breakin’ down “5 key differences” that every React dev should definitely know. Especially if they want to get a new role! BTW, read more about “15 Real React Interview Questions I Was Asked in Recent Job Interviews“!
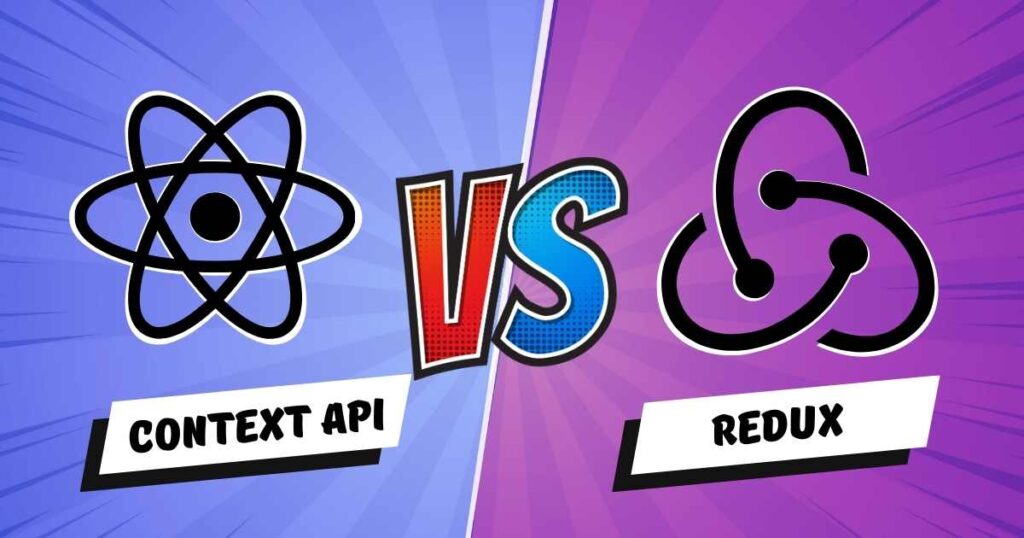
Redux vs Context — What Is Better?
Choosing between Redux vs Context depends on what you’re building. It depends on how complex your state is and how fast things change. There’s no single winner here!
State management in React starts simply with props. Then, boom—your app grows. Props drilling everywhere. Chaos! You need a better way to share state between components!
Redux and the Context API solve this core problem — managing shared state. But they do it in very different ways.
“People throw around ‘Redux vs Context‘. But they’re built for different jobs!!“
Redux is a heavy-duty toolbox with tons of extra setup — but powerful once it’s rolling! Context is lighter and built into React! Great for simple stuff, but it struggles with bigger apps.
Feature | Redux | Context API |
---|---|---|
State Management | ✅ Centralized | ❌ Needs useState/useReducer |
Prop Drilling Solution | ✅ Not its purpose | ✅ Main use |
Built-in to React | ❌ Needs install | ✅ Yes |
Performance Control | ✅ Fine-tuned via selectors | ❌ Can re-render a lot |
Debugging Tools | ✅ DevTools, time-travel | ❌ Basic console logs |
For complex state, global logic, or tons of API calls — Redux is better. Redux middleware (Thunk, Saga, RTK Query) make async things possible and smooth. And the Redux DevTools? (aka “time-travel debugging”) — chef’s kiss. It’s built for scale (aka future-proofing your app).
To handle lots of async data fetching, Redux with middleware wins!
For small apps or specific features, React Context works just fine. It is chill and clean! Use Context API when state changes infrequently and it doesn’t need extra libs. Context can slow things down. It rerenders everywhere, and debugging it is such a pain!
“Context is perfect for simpe features — authentication, theme switcning, language settings, so on…”
Don’t know where to start? Pick Context API! Context works until it does not. When you start reinventing the wheel, switch to Redux — it saves you when things blow up.
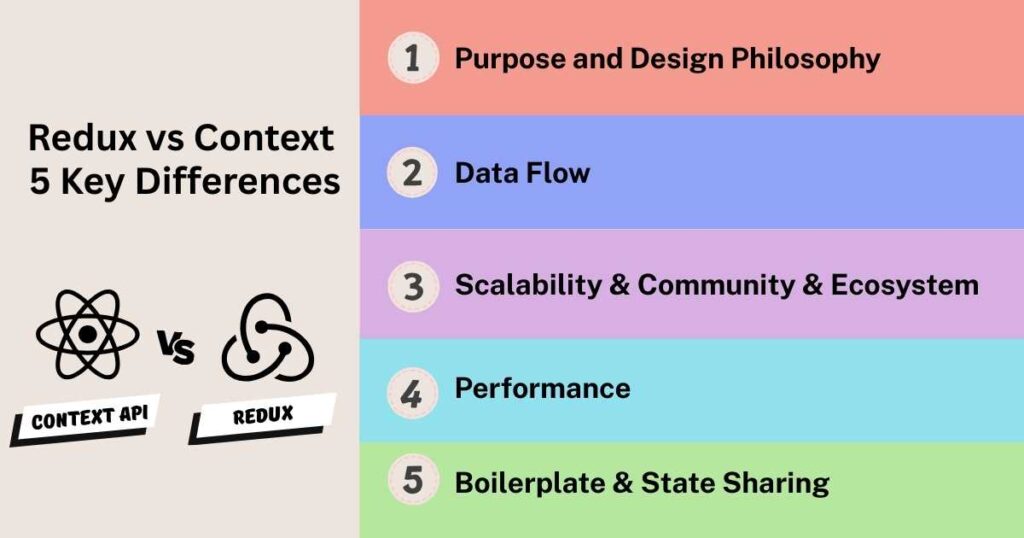
1 Purpose and Design Philosophy
Redux is a state management library. Period!! It controls app-wide state in one predictable place. You get one single read-only source of truth and a single way of processing data.
Redux follows the Flux pattern where data only flows in one direction. You don’t edit it directly! Instead, you use actions and reducers. That makes debugging, testing, and tracking state changes way easier.
It’s not just for React either — Redux is framework-agnostic! This means it is abstracted enough so you can swap it out anytime.
React Context API was built for prop drilling problems. Context is a dependency injection tool — it delivers state to ANY component in the app.
Context doesn’t manage state. It just passes it around. You still need useState()
and useContext()
to handle state.
Context is a dependency injection tool for a single value, used to avoid prop drilling. Redux is a global state managment tool.
Unfortunately, Context API is slow for large state logic. It rerenders React components every time state changes. So, use it for static or rarely changing values — dark mode, language setting, auth user.
Also, Context is React-only. It’s baked into React, so if you’re switching frameworks, it ain’t going with you.
2 Data Flow — Redux vs Context API
I mentioned the data flow difference — Redux uses Flux flow, while Context uses useState()
+ useContext()
. Cool! Now, let’s break it all down.
Redux’s Unidirectional Data Flow
Redux keeps things strict. It has one global store — your single source of truth. Data only flows in one direction.
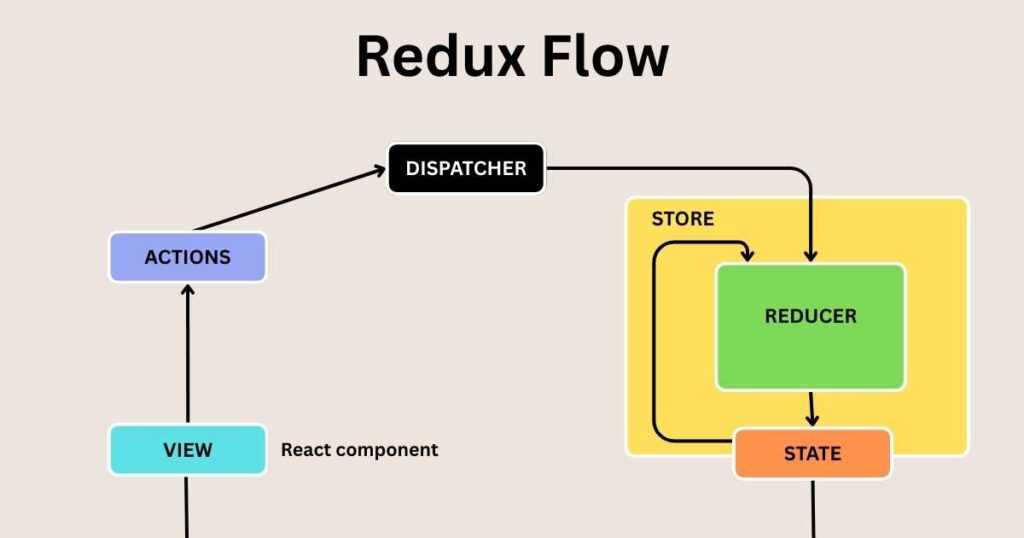
Let’s say a button gets clicked. Dispatch fires an action (a plain JS object) with a type
field. That action hits the reducer — a pure function that generates a new state. Finally, the state in the store changes, and your components use Selectors to pull in the fresh state and rerender.
Redux data always flows one way: Component ➜ Dispatch ➜ Action ➜ Reducer ➜ New State ➜ Selector ➜ Component.
Redux basic flow:
- Store – Holds the global state.
- Dispatch – Dispatches an action to change the store.
- Action – A plain JavaScript object with a
type
field. - Reducer – A pure function takes old state + action → returns new state.
- Selector – Grab specific state data.
Because the state change only happens in reducers, and nowhere else, Redux is predictable. You can track what happened, when, and why (with Redux devtools).
Redux just feels more scalable once async hits the fan. Middleware like redux-thunk
or redux-saga
handle async stuff, like API calls. Learn more about “What Is an API Call? Everything You Need to Know in 5 Minutes“!
React Context API Component Tree Flow
Now, the Context API vibe is TOTALLY different. Context is NOT for global state management — it’s more like a “dependency injection tool”. It skips the whole action -> reducer -> store dance unless you bring in useReducer()
manually.
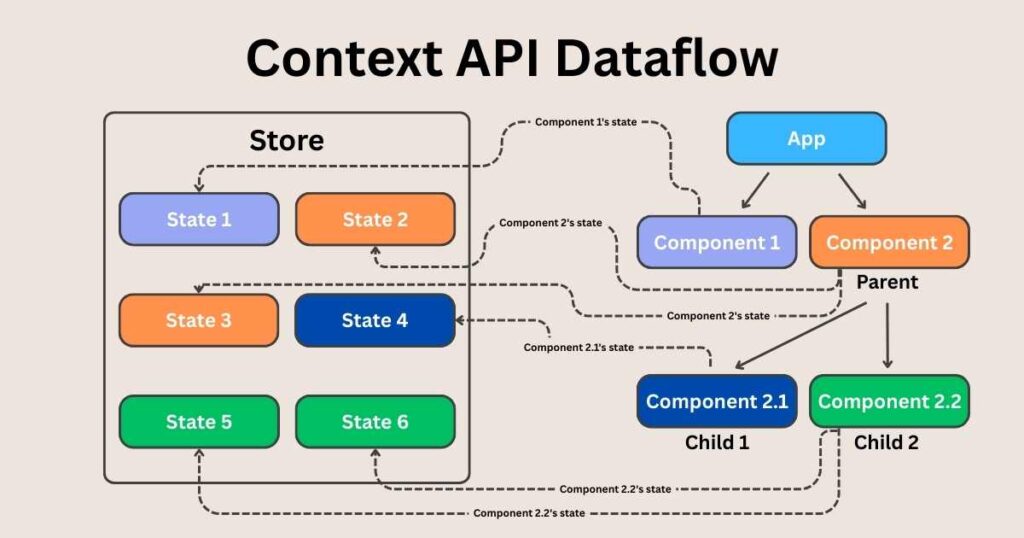
You pass data down your tree with the Context <Provider
/>. Now, any component below can access it with useContext()
— NO passing props all the way down!!
React Context API flow: Provider ➜ State Value ➜ Consumers via useContext
There’s no official async support for Context API! You can mix useContex()
with useReducer()
, but still don’t get middleware out of the box. So for async stuff, you’re gonna need use-context-selector
or Zustand!
3 Scalability & Community & Ecosystem — Redux vs Context
When you’re building big apps — lots of screens, features, moving pieces — Redux handles it better. It’s built for scale. Context? It couldn’t keep up there. (Not hating, just facts!)
Redux’s Scalability and Ecosystem
Redux’s been around a long minute. That means it’s packed with tools!
Redux stays stable and is easier to track because it keeps all state in one spot. And the tools that come with Redux make it even better!
Need to debug something weird with app state? Redux DevTools lets you rewind, inspect, and see exactly where things went sideways!
For async logic, there’s middleware. These tools help you intercept the Flux flow and await the remote data:
- redux-thunk keeps things simple.
- redux-saga gives more control, like canceling requests or handling retries.
With Redux middleware, you can write cleaner code and avoid spaghetti logic. Learn more about “What Is Spaghetti Code – How To Avoid And Fix“!
Redux Toolkit makes Redux way less annoying to set up. You get slices and reducers and store straight out of the box — cleaner code, less stress! Period.
Context’s Growing Patterns
Context struggles when you scale. Passing down many contexts? Messy. Updating too often? Laggy.
React Context API is cool, but gets clunky in bigger apps.
When you use it in complex apps, you’ll run into too many re-renders or deeply nested <Providers/> (Provider inside a Provider inside a Provider). You can try workarounds — custom hooks and split contexts. It helps, but only so much.
Problem | Context Workaround |
---|---|
Too many re-renders | Use useMemo and split contexts |
Shared state logic | Extract to custom hooks |
Nested providers | Use composition or ProviderComposer pattern |
When you’ve got a complex state with a ton of actions, it’s probably time to switch to Redux.
Community & Support
Both tools have good support, but Redux wins on size and depth. It’s older, battle-tested, and way more integrated. You’ll find tutorials, libraries, and even dev tools built around it.
Dev communities love Redux. Tons of guides, examples, and Stack Overflow answers. You’re never coding in the dark — even the hardest stuff someone’s already solved.
Redux plays nice with everything — React Router, GraphQL, REST, whatever!! Plug it with WebSockets, animations, and even React Native!
Context API is newer and more lightweight, so it doesn’t have that depth yet.
Context is part of React, so it’s always gonna be there — built-in, lightweight, and simple. But the ecosystem is smaller. Fewer tools, fewer patterns.
4 Performance — Redux vs Context
Redux and Context are not the same regarding performance. Not even close! Let’s break it down so your app doesn’t become a laggy mess.
Feature | Redux | React Context API |
---|---|---|
Fine-grained re-renders | ✅ connect/useSelector | ❌ all consumers re-render |
Memoized state handling | ✅ Reselect, normalize trees | ✅ useMemo (limited help) |
Best for frequent updates | ✅ Built for it | ❌ Can slow things down |
Easy to scale | ✅ Handles big state | ❌ Gets tricky fast |
Setup complexity | ❌ More boilerplate | ✅ Plug-n-play simplicity |
Redux’s Performance Optimizations
Redux is built to avoid unnecessary re-renders. It uses connect()
and useSelector()
to only update what actually needs updating.
Redux re-renders only when that exact part updates. You can listen to one tiny piece of state (say, user.name
) and Redux won’t care what else changes.
Bonus tip: You can memoize selectors with Reselect! That keeps expensive calculations from running when nothing has changed.
Redux wants your data flat. Flat = fast! The more normalized your state, the faster things run.
Context’s Performance Challenges
Whenever the context value changes, React rerenders ALL components that use that context! Yep. Even if they are not subscribed to the changed value.
<MyContext.Provider value={{ theme, user }}> <ComponentA /> <ComponentB /> </MyContext.Provider>
Every time user
changes, Context makes React re-render both <ComponentA/>
and <ComponentB/>
. Brutal!!
To fix that, you can split up your contexts:
<AuthContext.Provider value={user}> <ThemeContext.Provider value={theme}> <App /> </ThemeContext.Provider> </AuthContext.Provider>
This way, <ThemeContext/>
doesn’t care about user
changes. Memoization is another trick that lets you cache your context value.
5 Boilerplate & State Sharing
Redux vs Context ain’t even close when we’re talking about boilerplate. Redux comes packed with a ton of setup code. Like actions, reducers, selectors, thunk middleware — you name it. Meanwhile, React Context API rolls up with just a createContext()
and a <Provider/>
, and boom — you’re already sharing state!
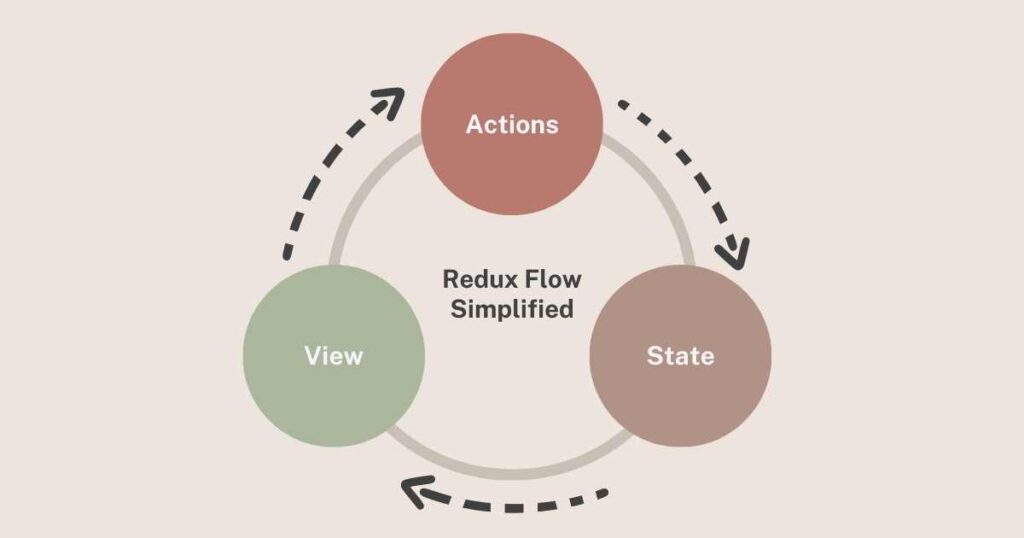
Redux’s Setup and Organization
I won’t lie — Redux has more boilerplate. That’s just facts! But that boilerplate gives you control, power, and organization
In Old-school Redux (pre-Redux Toolkit), you need like 6 separate files before your app does anything! For that, you need to:
- Define action types
- Write out action creators
- Manually build reducers
- Combine everything in a rootReducer
- Wire up the store
- Then, connect components using HOCs or hooks like
useSelector()
Then came Redux Toolkit (RTK). It abstracted like 80% of that boilerplate. Now you write a slice, and it gives you actions + reducer in one.
// From Redux Toolkit const todosSlice = createSlice({ name: 'todos', initialState: [], reducers: { addTodo: (state, action) => { state.push(action.payload); } } });
Redux slices + thunks are easy to isolate. You can test pure functions without needing the DOM or React.
Folder structure matters in Redux — we often use feature-based folders:
/features /todos - todosSlice.js - todosSelectors.js - todosThunks.js
You keep your logic separated — actions in one file, reducers in another. It sounds like more work, but it actually makes things easier to read and test.
Context’s Simpler Approach
Now, let’s talk about Context’s boilerplate. Wanna share state across your app? All you need is this:
const ThemeContext = React.createContext(); <ThemeContext.Provider value={{ theme: 'dark' }}> <App /> </ThemeContext.Provider>
Call createContext()
and wrap your app in a <Provider/>
. Use useContext(ThemeContext)
wherever you need the value.
Sounds simple, right? But when your app grows? You’ll end up juggling five, six, maybe even ten different contexts. Nesting hell!!
To organize multiple contexts, the best bet is to create a separate file per context:
/contexts - ThemeContext.js - AuthContext.js - CartContext.js
It keeps things clean, easy to follow, and easy to fix. DO not cram all your contexts into one file or one folder! Splitting them makes testing, updating, and replacing one easier WITHOUT messing up the others!
Conclusion — Redux Vs Context API
When you’re deep in React, choosing Redux vs Context isn’t just some side gig decision. It matters — big time!
I always say: Start with Context, scale with Redux!
Don’t let anyone tell you one’s better for everything — that’s just lazy thinking. It’s about choosing the right tool for the job.
Try both. For real. Whip up the same component using Redux and Context. Know your tools. Own your stack. Build dope apps. That’s the move!!