TypeScript interview questions are more practical and hands-on.
Recent surveys show that around 70% of developers prefer TypeScript for large-scale applications. And that is what matters for your next interview — companies are ACTIVELY hunting for TS pros!
This list of the 10 best TypeScript interview questions is what I’ve been asked recently! It’s perfect for interviewers and candidates prepping.
Looking for more insights? Read my “Most Recent Frontend Interview Questions in 2025 With Answers.“
Understanding TypeScript and Its Role in Interviews
Think of TypeScript as JavaScript’s smarter cousin who always checks their homework twice. It adds a safety net to your code by catching bugs before they happen.
Understand that TypeScript is a game-changer. TypeScript brings static typing to JavaScript.
function greetUser(name: string) { return `Hey ${name}, welcome to the party!`; }
In plain JavaScript, you could accidentally pass a number name, and things would get weird. But TypeScript’s got your back — it’ll wave a red flag before you even run the code.
Here’s what makes TypeScript a total boss:
- Better error catching
- Awesome code hints
- Makes refactoring way less scary
- Self-documenting code
Talk about real problems TS solves. Like I debugged a massive JS project — TS would’ve caught those issues in seconds. Switch from plain JS to TypeScript to drop the number of runtime errors like crazy!!
Find here “What Does A Front End Developer Do: 7 Core Responsibilities“
15 Popular TypeScript Interview Questions
These typescript interview questions test your understanding of TypeScript’s core concepts — types, interfaces, and generics. We cover the basics and some advanced topics.
I faced these TypeScript interviews questions myself. These questions show what companies are looking for right now!
When answering this in interviews, don’t just list features — share a story about how TypeScript solved a real problem in your experience.
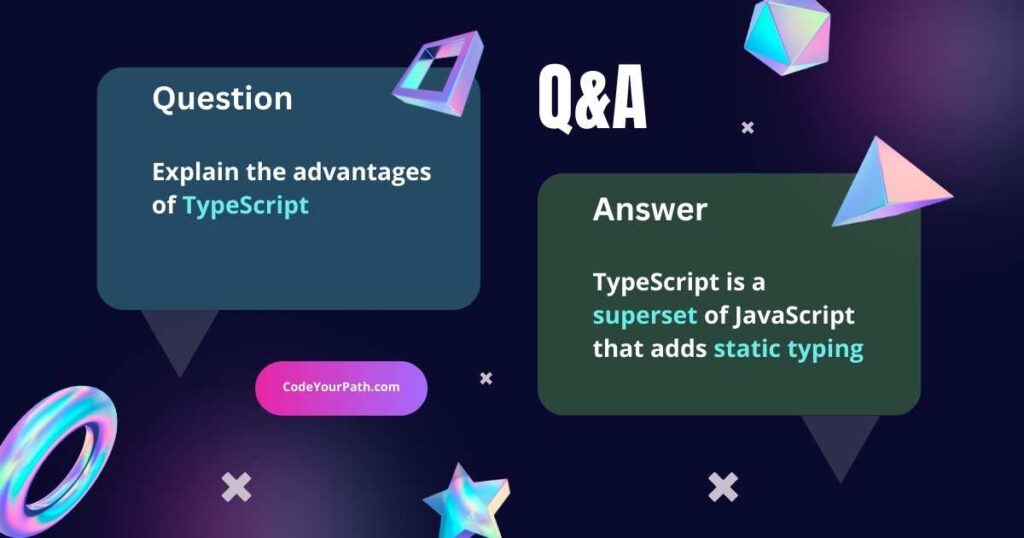
1. Explain the Advantages of TypeScript
Interviewers love this question! They want to hear about real benefits, not just textbook answers.
TypeScript is a superset of JavaScript that adds static typing to JS, so you catch errors before running your code.
// JavaScript way (risky!) function addNumbers(a, b) { return a + b; } // TypeScript way (safe!) function addNumbers(a: number, b: number): number { return a + b; }
Many devs think TypeScript is just about adding types, but it’s way more than that. TS catches errors at compile time, unlike JS, which only throws errors during runtime.
Imagine you’re working on a large JavaScript project with hundreds of files. A teammate renames a function but forgets to update all instances. In JavaScript, you would miss this until runtime (yikes!). But in TypeScript, the compiler instantly warns you about broken references.
Use TypeScript with tools like ESLint and Prettier for even cleaner code.
Real-World Benefits:
- Improves code quality — Easier refactoring without breaking things
- Your code becomes self-documenting
- Better IDE support with autocomplete suggestions
- Scales well for large apps
Find more app improvements in “Front End Optimization Techniques — Best 12 Options“
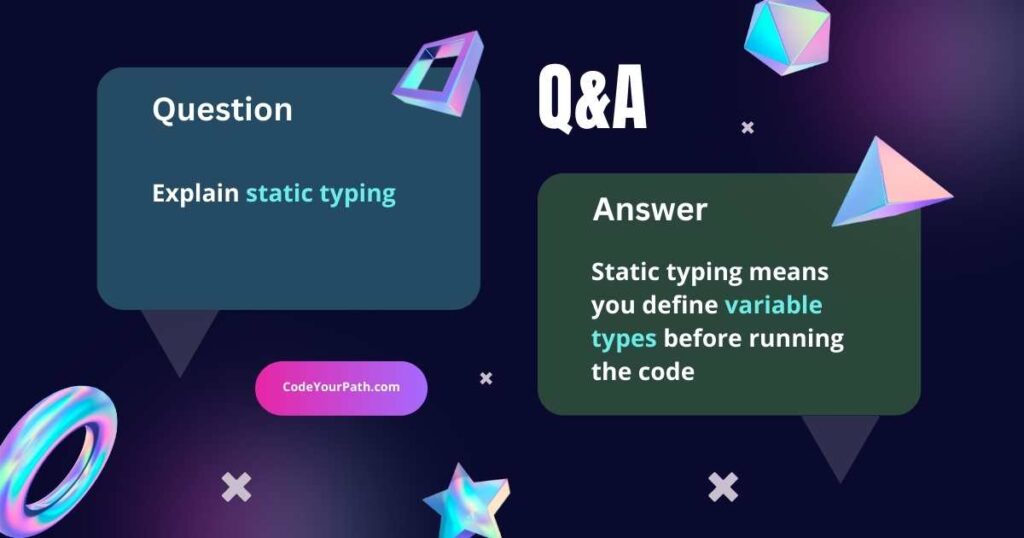
2. Explain Static Typing and Why It Matters When Coding In TypeScript
Static typing means you define variable types (like string
, number
, or boolean
) before running the code — at compile time (not runtime). This helps catch errors like passing a string where a number is expected.
Static typing makes your code more predictable. Once you say a variable is a number, it stays a number — no surprise allowed!
Feature | Static Typing | Dynamic Typing |
---|---|---|
Error Detection | At compile time | At runtime |
Type Declaration | Required | Not required |
Code Optimization | More optimized | Less optimized |
Flexibility | Less flexible | More flexible |
Static typing is important for larger projects where multiple developers work together. It helps teams make changes with confidence and understand each other’s code.
TypeScript approach is optional — you can use static typing as much (or as little) as you want. Add types to your JavaScript projects gradually.
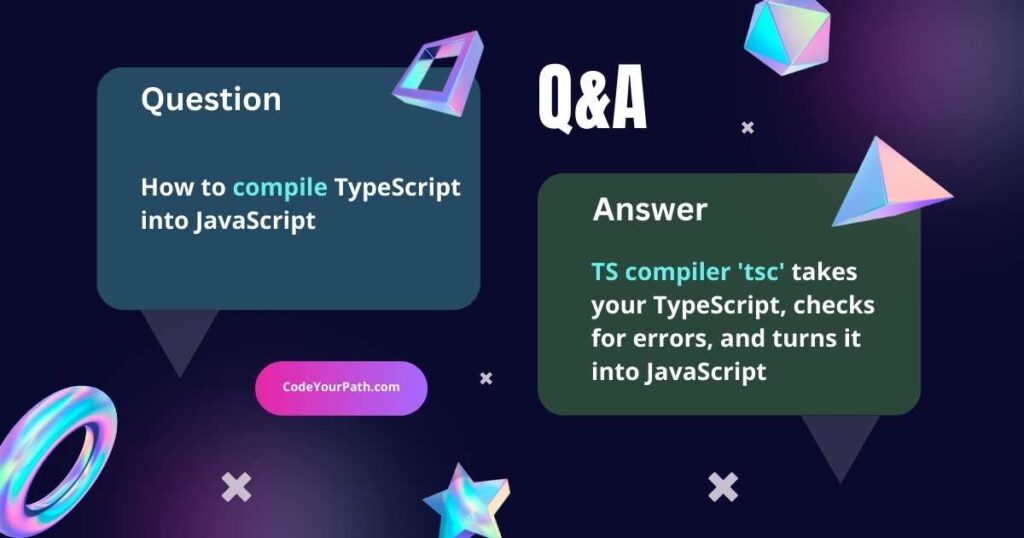
3. How Do You Compile TypeScript Code into JavaScript?
TypeScript introduces features like static typing. But browsers only understand JavaScript. So, TS code must be compiled into JS before it can run in browsers or Node.js.
The TS compiler (‘tsc’) takes your TypeScript, checks for errors, and turns it into regular JavaScript.
You’ll want to create a special file called tsconfig.json. This file tells TypeScript how you want your code compiled. You can set things like where your files go (outDir) and what version of JavaScript you want (target).
Use --watch
to auto-compile when files change:
tsc --watch
Now your TypeScript files turn into JavaScript without you lifting a finger!!
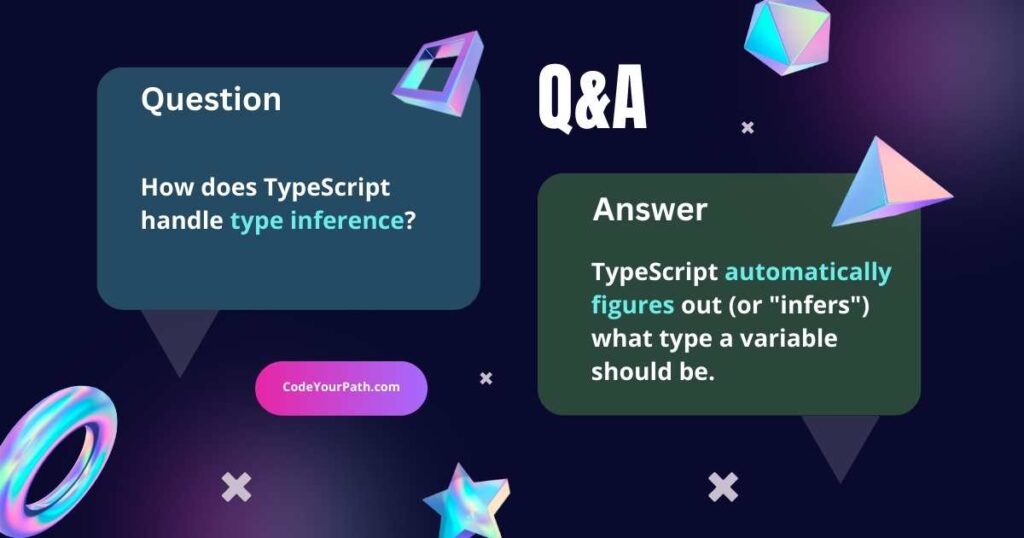
4. How Does TypeScript Handle Type Inference?
TypeScript automatically figures out (or “infers”) what type a variable should be without you telling it.
When you create a variable with a value, TypeScript automatically knows its type:
let name = "John" // TypeScript knows it's a string let age = 25 // TypeScript knows it's a number let isActive = true // TypeScript knows it's a boolean
TypeScript can figure out what type a function returns based on what’s inside it:
function multiply(a: number, b: number) { return a * b; // TypeScript knows this returns a number }
Type inference makes your life easier (NOT harder). Use it when it makes your code cleaner, but don’t be afraid to add explicit types.
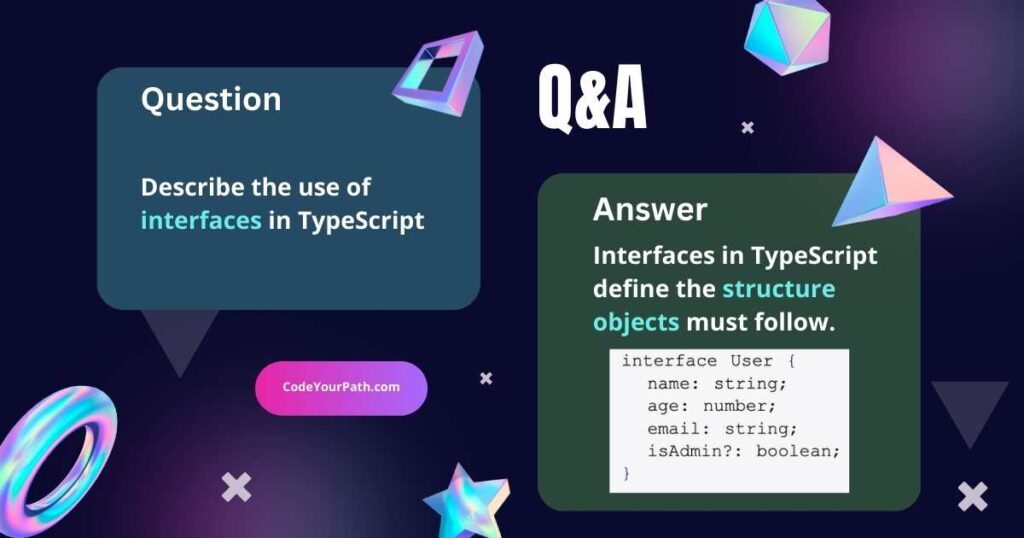
5. Describe the Use of Interfaces in TypeScript
Interfaces in TypeScript define the structure objects must follow. It defines a syntactical contract that specifies what properties and methods an object must have.
interface User { name: string; age: number; email: string; isAdmin?: boolean; // Optional property } const user1: User = { name: "John Doe", age: 30, email: "john@example.com" };
This ensures user1
has name
, age
, and email
but isAdmin
is optional.
Remember, interfaces DON’T generate objects at runtime. They’re just for type-checking.
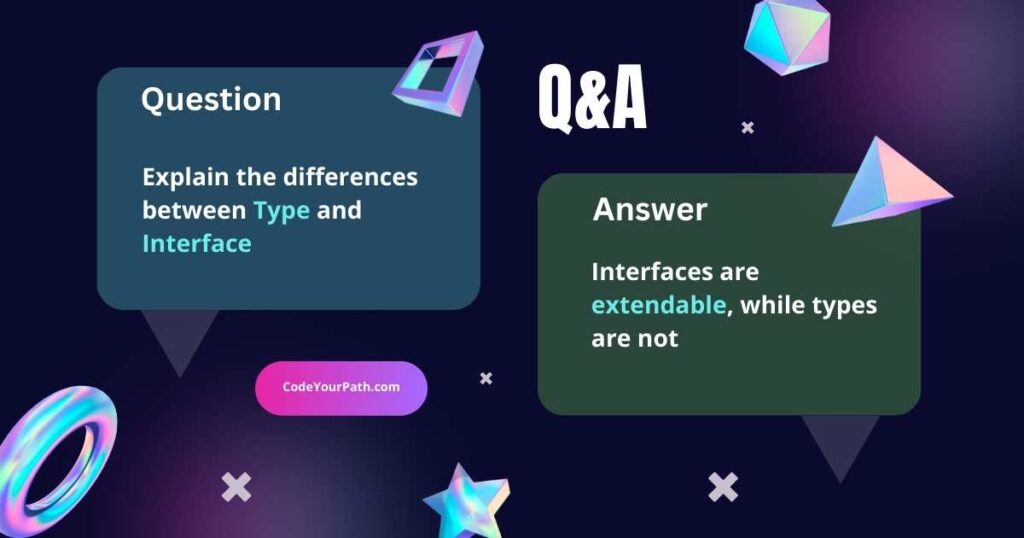
6. Explain the Differences Between Type and Interface
Types and interfaces might look similar at first glance.
// Using Type type User = { name: string; age: number; } // Using Interface interface User { name: string; age: number; }
TypeScript team actually prefers interfaces over types when possible. Interfaces are extendable, while types are not (this is super important for OOP):
interface Animal { name: string; } interface Dog extends Animal { bark(): void; } // With types, you'd need to use intersections type Animal = { name: string; } type Dog = Animal & { bark(): void; }
Some devs use type
for everything, but interface
is better for object shapes. When working on larger projects (like the ones I’ve handled at FOX), I typically use interfaces for public APIs.
Feature | Interface | Type |
---|---|---|
Extensible | ✅ Can be extended with extends | ❌ No built-in extension (but can use & ) |
Merging | ✅ Auto-merges when redeclared | ❌ Doesn’t merge (overwrites instead) |
Union & Intersection | ❌ Nope | ✅ Supports |
Performance | ✅ Faster (better for large codebases) | ⚠️ Slightly slower (edge cases only) |
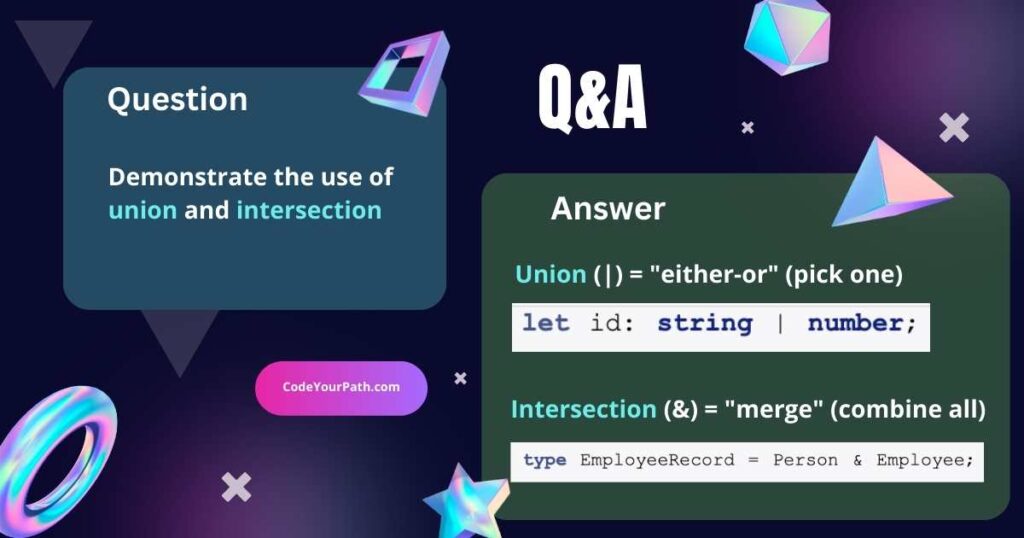
7. Demonstrate the use of union and intersection types
Union (|
) and intersection (&
) types help make TypeScript flexible.
A union type lets you use more than one type for a value. Think of it as saying “This can be either this OR that.”
let id: string | number; // Union type - can be either string OR number id = "ABC123"; // ✅ Works fine id = 123; // ✅ Also works id = false // ❌ Error: 'boolean' not allowed
id
is either string
or number
— not both at once.
A union type ( | ) lets a variable hold multiple types.
An intersection type ( & ) merges multiple types into one.
type Person = { name: string }; type Employee = { id: number }; type EmployeeRecord = Person & Employee; const employee: EmployeeRecord = { name: "John Doe", id: 101 };
Now, EmployeeRecord
has both name
and id
— two types in one!!
Union (
|
) = “either-or” (pick one)Intersection (
&
) = “merge” (combine all)
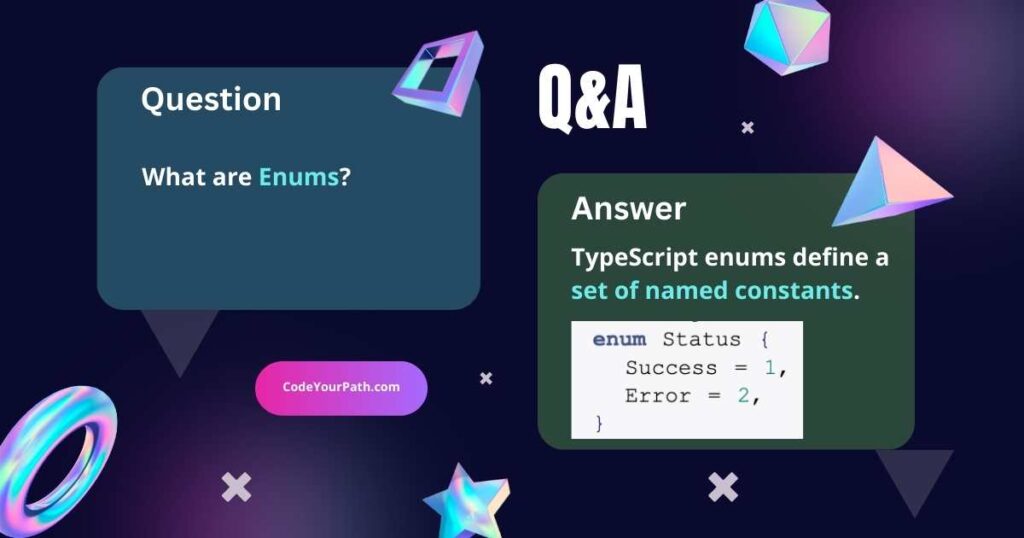
8. What Are Enums and Why Choose Them? — TypeScript Interview Questions
TypeScript enums are special data structures that let you define a set of named constants.
When you create a numeric enum, TypeScript automatically assigns numbers starting from zero:
enum Direction { North, // 0 East, // 1 South, // 2 West // 3 }
You can also create enums with string values:
enum Direction { North = "NORTH", East = "EAST", South = "SOUTH", West = "WEST" }
Enums make code way easier to understand. They give you awesome type checking and your code editor (VSCode) helps you with smart suggestions. Plus, enums allow reverse mapping.
Enums’re perfect for status codes (SUCCESS, ERROR, PENDING) or user roles (ADMIN, USER, GUEST).
// Using Enum enum Status { Success = 1, Error = 2, } // Using Plain Object const Status = { Success: 1, Error: 2, };
In this example, Status.Success
is clearer than Status['Success']
. (Plus, enums are just cooler, IMHO.)
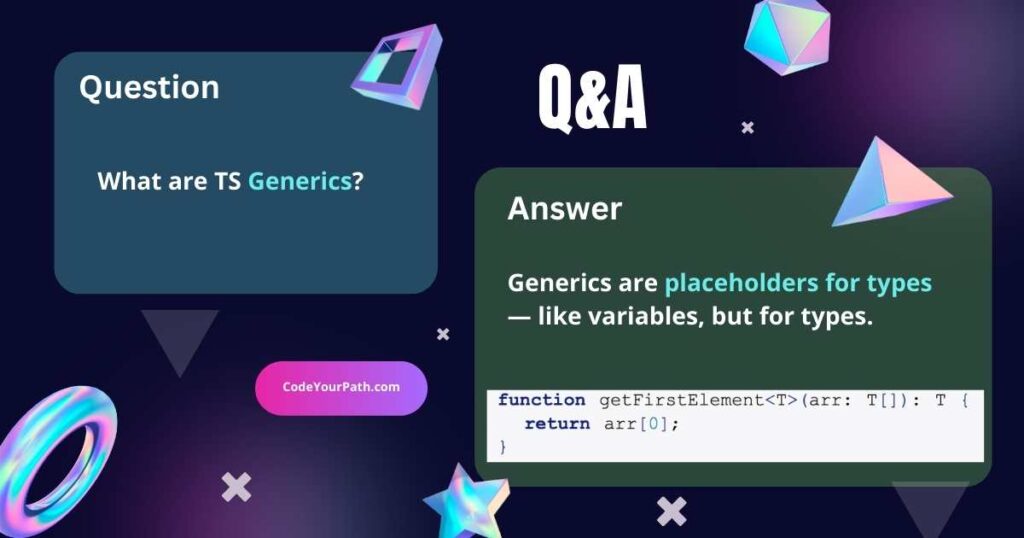
9. What Are TS Generics and How Do You Use Them? — TypeScript Interview Questions
Generics help you create components that work with different types.
Think of generics as placeholders for types — kinda like variables, but for types.
Let’s say you’re building a function that returns the first element of an array. Without generics, you’d write something like this:
function getFirstElement(arr: any[]): any { return arr[0]; }
Looks fine, right? Nah, not really…
…It returns any
, so you lose type safety. Here’s how generics fix this:
function getFirstElement<T>(arr: T[]): T { return arr[0]; } const array = [1, 2, 3] const firstNum = getFirstElement(array); // TypeScript knows it's a number!
Here, T
is a generic type. You can swap number
array with string
, boolean
, or any type you want
Common TypeScript generics pitfalls:
- Overusing generics — Don’t make things complicated for no reason!
- Forgetting to constrain generics — use
extends
when needed.
10. What Are Decorators in TypeScript? — TypeScript Interview Questions
Decorators are special markers (starting with @
symbol) that modify classes, methods, or properties. They add extra powers to your code without changing its core structure.
Imagine you have a class that needs logging. You can create a decorator that logs every method call automatically.
function log(target: any, key: string) { console.log(`Method ${key} was called!`); } class MyClass { @log myMethod() { console.log("Doing something..."); } }
Here, the @log
decorator logs a message every time I call myMethod
.
Decorators DO NOT change the method itself — they just wrap it! Decorators look weird if you’re not used to them.
Not all browsers support TS decorators natively. Use a compiler like Babel if needed.
Use them to apply the same logic across multiple methods. Decorators are great for cross-cutting concerns (like security or error handling).
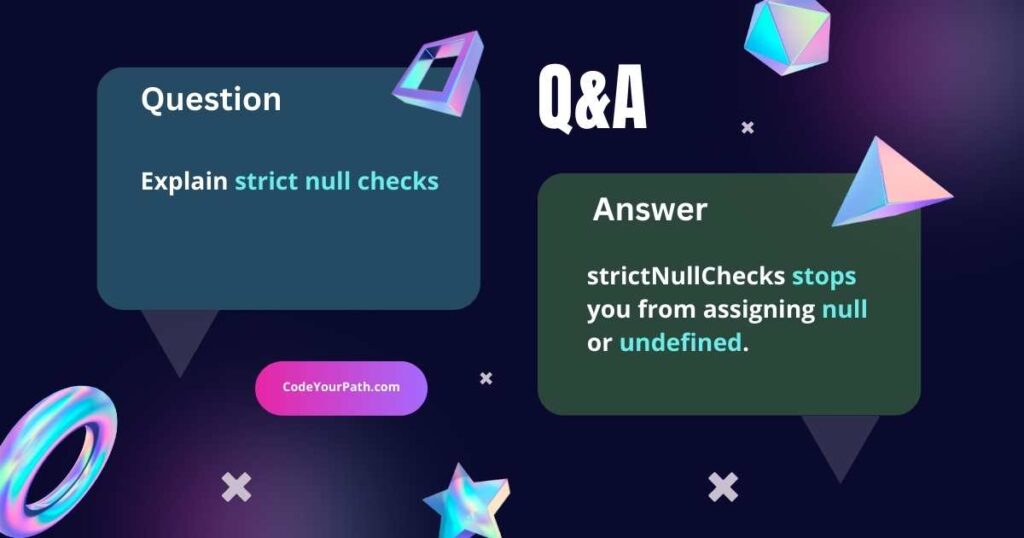
11. Explain Strict Null Checks and How They Prevent Runtime Errors — TypeScript Interview Questions
These typescript interview questions are classic in TypeScript interviews.
By default, TypeScript lets you assign null
or undefined
to any variable. This lead to “cannot read property of undefined” errors that pop up in JavaScript.
When you turn on strict null checks setting strictNullChecks: true
in tsconfig.json, TypeScript gets more strict about how you handle null and undefined values.
// Without strict null checks - dangerous! let name: string = null; // This works 😱 // With strict null checks - safer! let name: string = null; // Error! 🚫 let safeName: string | null = null; // This works ✅ if (safeName !== null) { console.log(name.length); // Safe to use now! }
When strictNullChecks
is on, TypeScript stops you from assigning null
or undefined
to variables unless you explicitly allow it. Just add this in your tsconfig.json
:
{ "compilerOptions": { "strictNullChecks": true } }
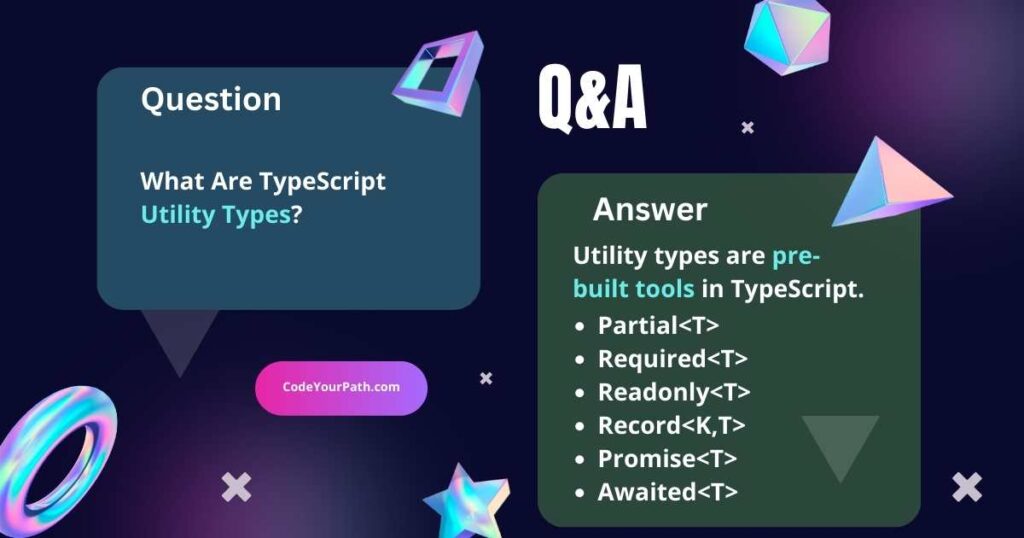
12. What Are TypeScript Utility Types? — TypeScript Interview Questions
Interviewers love asking about utility types because they show your depth of TypeScript.
Utility types are pre-built tools in TypeScript.
Utility types save time, reduce errors, and make your code cleaner. Instead of writing repetitive type definitions, you use them.
For example, Partial<T>
makes all properties of a type optional.
interface User { id: number; name: string; email: string; } const updateUser = (user: Partial<User>) => { console.log(`Updating user: ${user.name}`); }; updateUser({ name: 'Gene' }); // Works fine without id and email
The Required<Type>
does the opposite — it makes all properties mandatory.
Readonly<Type>
prevents properties from being changed after assignment.
Record<Keys,Type>
creates an object type with specific key and value types.
Utility Type | Purpose | Example | Common Use Case |
---|---|---|---|
Partial<T> | Makes all properties optional | Partial<User> → { name?: string, age?: number } | Form updates, PATCH requests |
Required<T> | Makes all properties mandatory | Required<User> → { name: string, age: number } | Data validation, complete objects |
Readonly<T> | Prevents property modification | Readonly<User> → immutable User object | State management, constants |
Record<K,T> | Creates key-value mapping | Record<string, number> → { [key: string]: number } | Dictionaries, dynamic objects |
Promise<T> | Represents async operations | Promise<string> → async string result | API calls, async functions |
Awaited<T> | Extracts Promise result type | Awaited<Promise<string>> → string | Async function returns |
Pick<T,K> | Selects specific properties | Pick<User, 'name'> → { name: string } | Creating subset types |
Omit<T,K> | Removes specific properties | Omit<User, 'password'> → User without password | Excluding sensitive data |
Extract<T,U> | Keeps matching types | Extract<string | number, string> → string | Type filtering |
Exclude<T,U> | Removes matching types | Exclude<string | number, string> → number | Type removal |
These utilities save tons of time. They’re especially useful in API integrations!!
13. How Does TypeScript Support Asynchronous Programming? — TypeScript Interview Questions
Interviewers want to know if you can handle long-running tasks (like fetching data) without blocking the app.
TypeScript supports async programming through Promises and the async/await syntax.
What You Should Cover:
- Async/Await: A cleaner way to handle Promises
- Promises: The backbone of async operations in JavaScript/TypeScript.
- Error Handling: How TypeScript’s static typing helps catch errors early
interface User { id: number; name: string; } async function fetchUser(id: number): Promise<User> { try { const response = await fetch(`https://api.example.com/users/${id}`); const user = await response.json(); return user; } catch (error) { throw new Error(`Failed to fetch user: ${error}`); } }
fetchUser
function:
- Uses
async
to define an asynchronous function. - Uses
await
to pause execution untilfetch()
completes. - Wraps it in
try/catch
to handle errors properly.
Async/await makes the code easier to read — no more .then()
chains!
14. Integrating TypeScript into a JavaScript Project — TypeScript Interview Questions
First things first — add TypeScript to your project.
Create a special tsconfig.json file. This file tells TypeScript how to handle your code — start with basic settings.
Don’t have to change everything at once! Start with your simplest, like utility or helper functions. Just rename them from .js
to .ts
. Take your time and keep converting files one by one!
Fix type errors as they pop up. Use any
if needed (but DONT overdo it).
Take it slow. Keep your old JavaScript files in one folder (usually ‘src’) and let TypeScript create new ones in another folder (usually ‘dist’).
Use allowJs
in tsconfig.json
. Add this option to let TypeScript work alongside JavaScript files:
{ "compilerOptions": { "allowJs": true } }
Keep your build process simple and set up basic scripts in your package.json
. This way, everyone on your team can work with the codebase easily.
Looking for other improvements? Read “UI UX Optimization: 9 Proven Strategies“
Conclusion: Most Important Typescript Interview Questions
TypeScript’s all about making JavaScript better. I’ve found that understanding TypeScript’s fundamentals is more valuable than memorizing complex features
TS type system, interfaces, and generics are fundamental typescript interview questions. Interviewers often ask about TS relationship with JavaScript and its benefits for large-scale applications.
Keep reading “How To Get Into IT Without a Degree in 2025: A Comprehensive Guide“