Do you want to ace your next tech interview? You need to know about front end optimization techniques.
Every tech company now asks about making websites faster. I see this in interviews all the time.
Most coding tests now include questions about speed and performance. Companies want to know if you can make their websites run better.
Front end optimization techniques makes websites faster and smoother for users. It’s like tuning up your car — when done right, everything just works better.
Understanding Front End Optimization Techniques
Let’s break this down into simple ideas. Think of optimization as cleaning up your room.
First, we look at how fast a website loads. This is like timing how quickly you can find things.
Next, we check when users can click buttons. It’s similar to making sure toys work when you pick them up.
We also watch for things jumping around on the page. Nobody likes it when stuff moves while they’re trying to click.
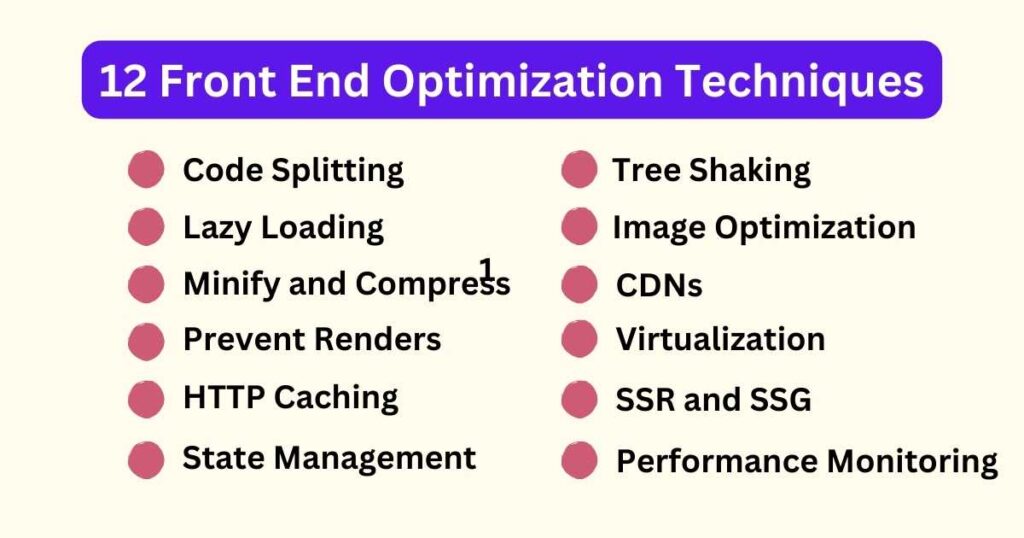
Core Front End Optimization Techniques
Here’s what companies want to know: “Can you make websites load faster?” They’ll ask how you’d fix a slow website. The answer isn’t always about writing faster code.
Prepearing for an interview? Read my “Most Recent Frontend Interview Questions in 2025 With Asnwers” post.
Sometimes, it’s about loading things in a smarter order. Other times, it’s about making files smaller.
I’ve helped many students prepare for interviews. The ones who get jobs know these tricks well.
Poor front end optimization techniques directly impacts your bottom line:
- Users abandon slow sites
- Search engines rank faster sites higher
- Mobile users expect near-instant loading
- Every second of a delay cost conversions
When you interview, they might ask you to spot performance problems. It’s like finding bugs but for speed.
Remember: Practice explaining these ideas in simple words. That’s what interviewers want to hear.
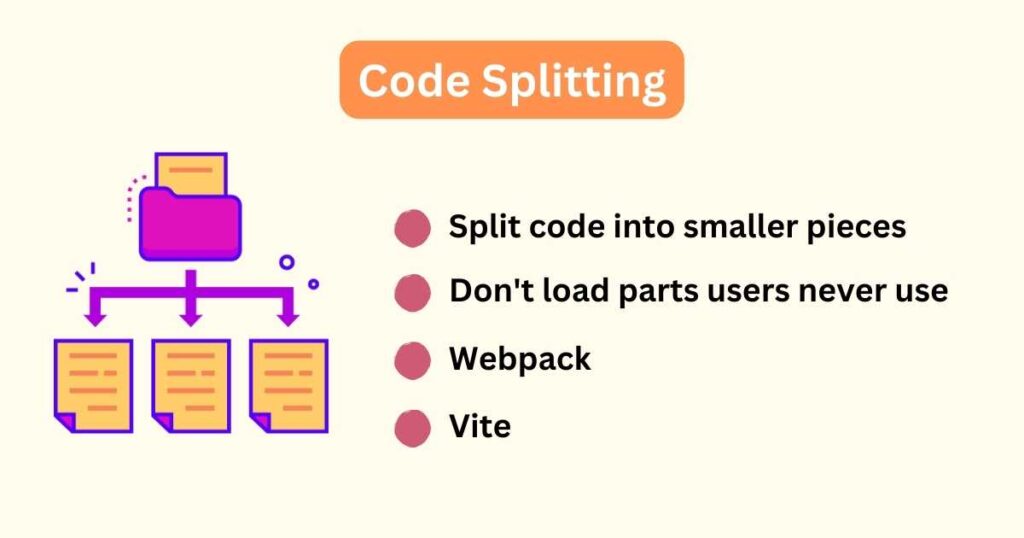
1 Modern Bundling and Code Splitting
Let’s talk about making websites load faster by splitting them into smaller pieces.
Think of your website like a big book. Instead of giving someone the whole book, you give them one chapter at a time. That’s code splitting!!
Modern tools like Webpack and Vite help us split our websites automatically. When someone visits your website, they only get the parts they need. The rest loads later when they need it.
For example, imagine a shopping website. The home page loads first, very quickly. The shopping cart only loads when someone clicks “Add to Cart” or “Go to Cart.”
This is super helpful for people using phones. They don’t waste data downloading parts of the website they might never use.
Bundling makes websites feel much faster. Users don’t wait for unnecessary stuff to load. They can start using the website sooner.
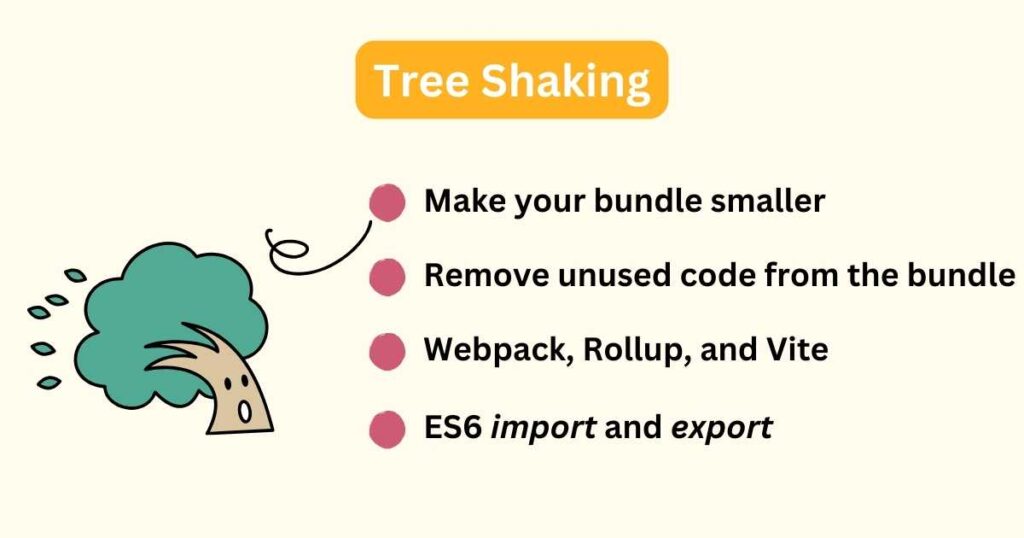
2 Tree Shaking and Dead Code Elimination
Think of tree shaking like cleaning out your closet. You remove clothes you never wear to save space.
Tree shaking helps make websites faster by removing unused code from your final package. It’s like only packing what you’ll ACTUALLY use for a trip.
Modern tools like Webpack, Rollup, and Vite can automatically remove unused code. But they need your help to work properly.
To make tree shaking work, use import and export statements. The old way of sharing code using “require” doesn’t work for tree shaking.
Here are some common mistakes that break tree shaking:
- Using the wrong type of imports or exports. Always use the new ES6 style instead of the old one.
- Having side effects in your code. Side effects confuse the cleaning process with unexpected changes.
- Not telling your tools which files are safe to clean up. Mark files that you can remove safely.
Remember: smaller packages load faster and make your visitors happier. Every bit of unused code you remove helps make your site speedier.
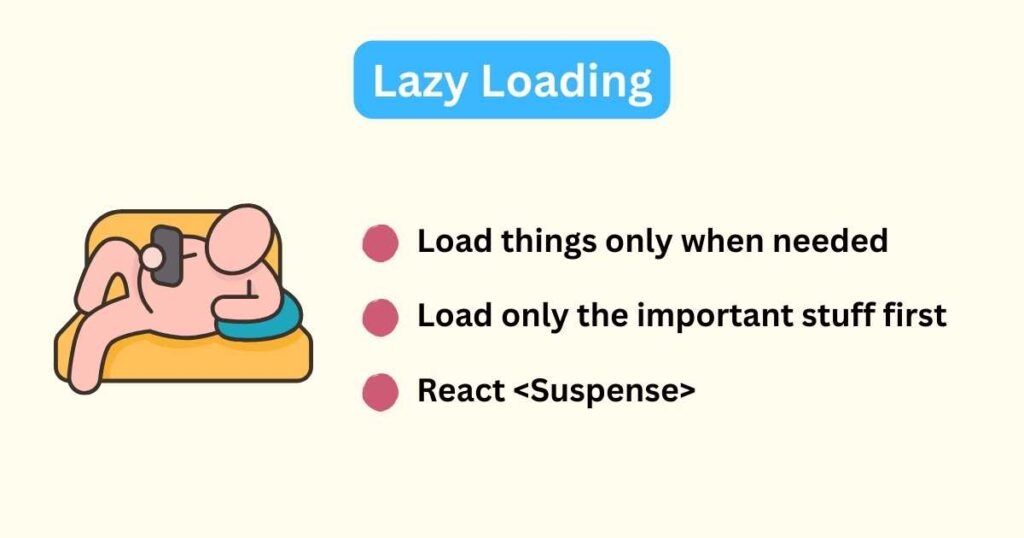
3 Lazy Loading
Lazy loading is like getting groceries from your car. Instead of carrying all bags at once, you bring them in as needed.
Lazy loading makes websites faster by loading things only when you need them. It’s like opening a book one page at a time instead of all at once.
This works great for things like:
- Pictures that are lower (not visible yet) on the page
- Videos that need to be clicked to play
- Parts of the website you only see after clicking buttons
When someone visits your website, only the important stuff loads first. Everything else waits its turn. This makes your website feel super quick and smooth.
For pictures, it’s really simple nowadays. Most modern browsers support lazy loading out of the box. You just need to tell the browser which pictures wait to load.
If you need more control, there are special tools that help. React (a popular tool) has a <Suspense> component that makes lazy loading SUPER easy. Look into “5 Real-World Examples of React Suspense“
The best part? Users love it! Pages load faster, and they use less internet data. This means happier visitors who stay on your website longer.
For example, imagine a shopping website with hundreds of product pictures. Without lazy loading, you’d wait forever for everything to load. With lazy loading, you immediately see the first few products.
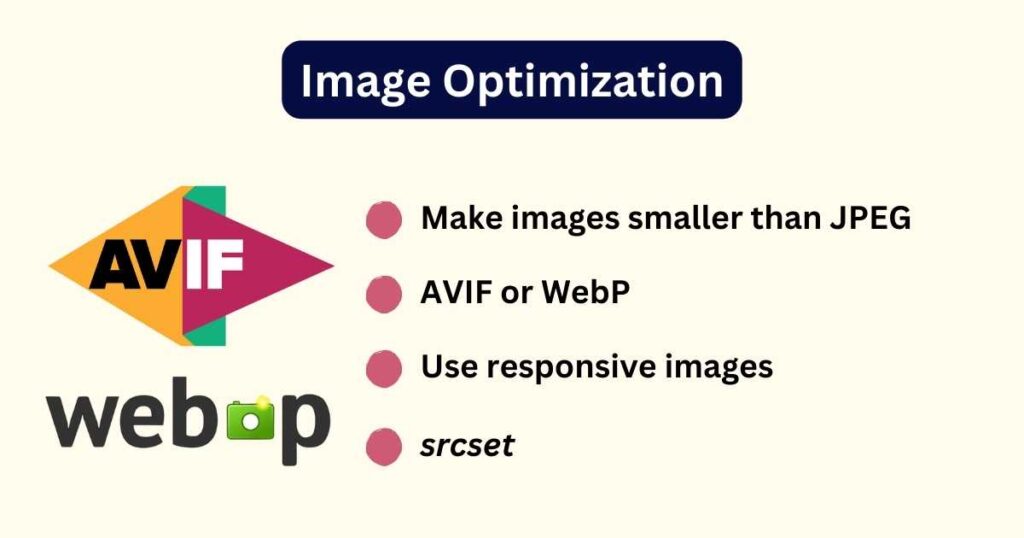
4 Image Optimization
Let’s talk about making your website’s pictures load super fast. Modern image formats — like AVIF and WebP — help with this.
AVIF is the new star of image formats. It makes files about 50% smaller than regular JPEG files. That means your website loads much faster!
WebP is an AVIF’s older cousin. It’s not quite as good at making files small, but more browsers support it. When you can’t use AVIF, WebP is your next best choice.
Making images work well on phones is super important too. That’s where responsive images come in. Think of it like having t-shirts of different sizes — one size doesn’t fit everyone.
Tell browsers to pick the right image size using srcset. It’s like giving the browser a menu of pictures.
Here’s a simple tip: start with AVIF for your images. If that doesn’t work, try WebP. Always keep a regular JPEG as a backup. This way, everyone can see your pictures (regardless of their browser).
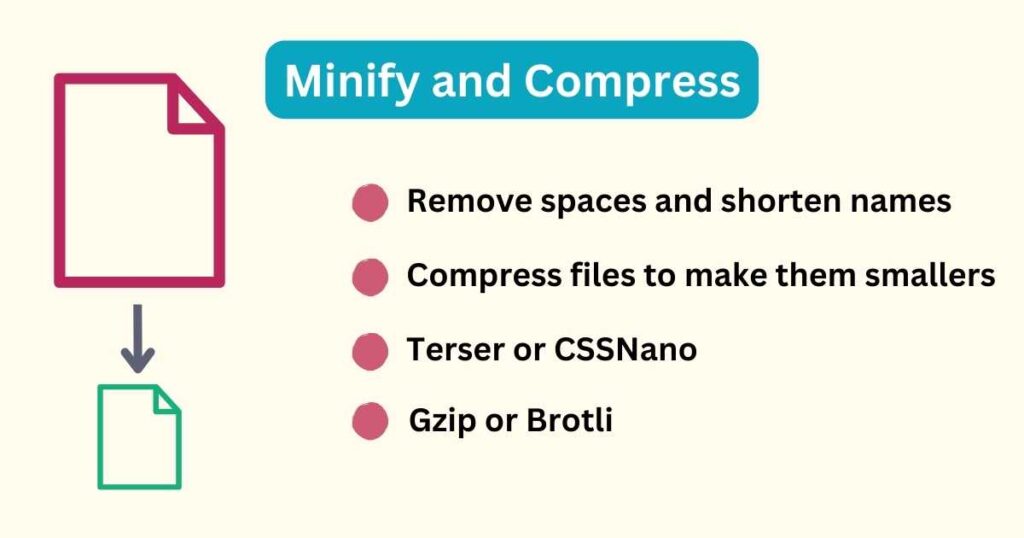
5 Minify and Compress Assets
Minification is like packing a suitcase really well. You remove all the extra space between clothes. That’s what minification does with code — it removes spaces and makes names shorter.
Compression is different. It’s like using a vacuum bag to make clothes even smaller. Your browser needs to “unzip” these files before using them.
When you use both together, you make files MUCH smaller. For example, a CSS file that’s 100KB shrinks to 20KB after minification and compression.
Modern tools make this easy. Terser helps with JavaScript files, while CSSNano works on CSS files.
For compression, most websites use either Gzip or Brotli. Brotli is newer and usually makes files about 20% smaller than Gzip.
You don’t need to do all this by hand. Modern website tools handle all this automatically. Just make sure to check that your website uses both — minification and compression.
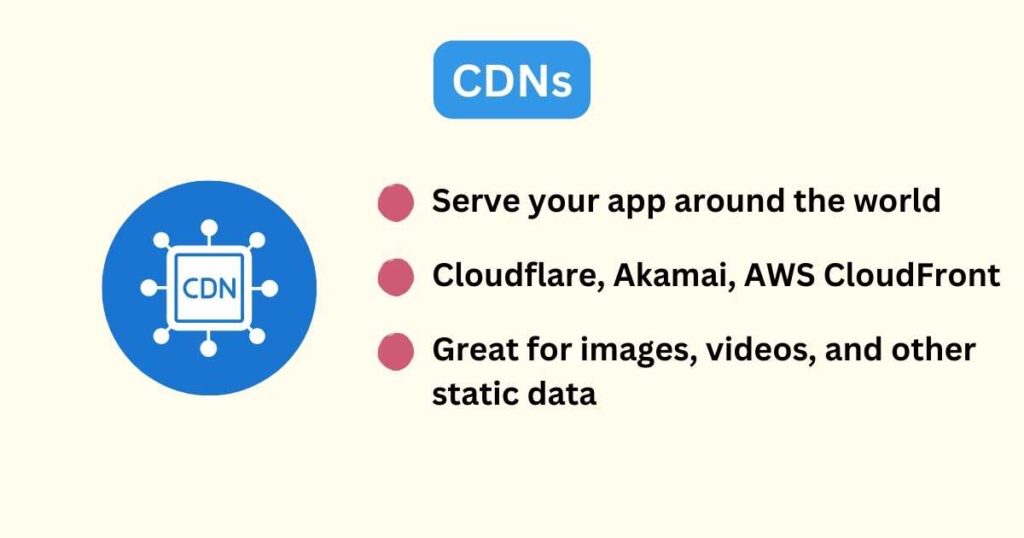
6 CDNs — Front End Optimization Techniques
A Content Delivery Network (CDN) is a group of servers spread around the world that deliver your website content. When someone visits your site, they get the files from the nearest server, not your main one.
Without a CDN, if someone in New York wants to see a website hosted in London, they must wait longer. With a CDN, they get the content from a nearby server in New York instead.
CDNs work best with content that doesn’t change often, like:
- Pictures and videos
- Style files (CSS)
- JavaScript files
- Downloads and documents
The most popular CDN choices are Cloudflare, Akamai, and AWS CloudFront.
Think about using a CDN when your visitors come from different countries. It’s also great if your website has lots of images or videos.
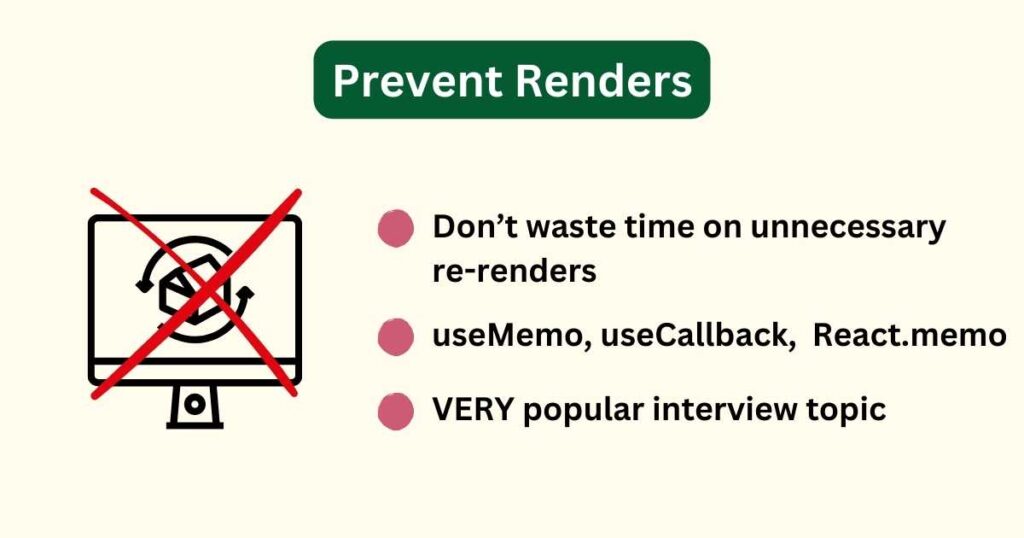
7 Prevent Unnecessary Renders in React.js
React checks your screen many times to see if anything needs to change. Sometimes it does this checking even when NOTHING is different. This wastes time and makes your app slower.
Imagine you have a calculator on your screen. Every time you press a button, React checks everything on the page. But really, it only needs to check the calculator numbers.
React has special tools to help stop this extra work — useMemo, useCallback, and React.memo. Find examples in “React useCallback Hook: Super Easy Guide in 5 Min!” post.
Don’t use these tools everywhere – that can actually make things slower! Use them when:
- Your page feels slow
- You have lots of things changing at once
- You notice the same calculations happening over and over
These tools are VERY popular interview topics!!
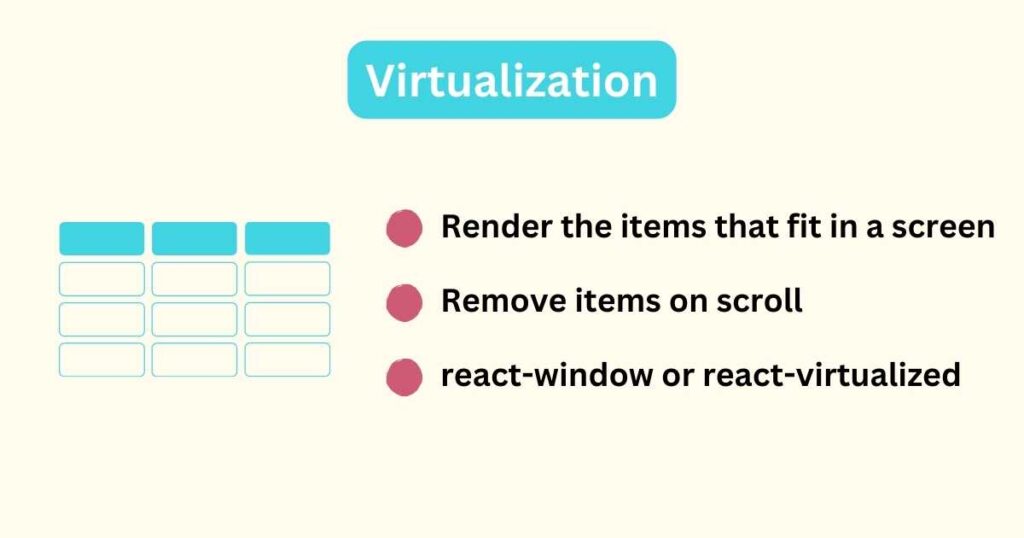
8 Virtualization for Large Lists
When you have a really long list, like 10,000 items, showing everything at once makes your website slow. It’s like trying to carry all your groceries in one trip – it’s too heavy.
Virtualization only creates the items that fit in your screen. When you scroll, it removes items you can’t see anymore and creates new ones. This is much faster than showing everything at once.
Two main tools help with this in React.js: react-window and react-virtualized. React-window is newer and faster, while react-virtualized has more features.
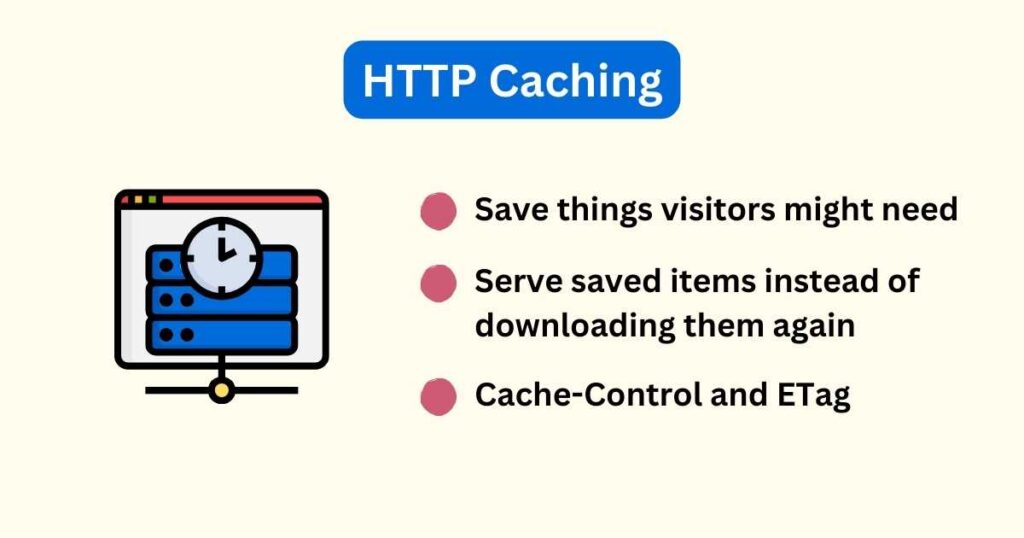
9 Implement HTTP Caching
HTTP caching is a smart storage box for your website stuff. It saves things your visitors might need again later.
When someone visits your website for the first time, their browser saves certain things. Next time they visit, the browser use these saved items instead of downloading them again.
You control what gets saved and for how long using special instructions — Cache-Control and ETag.
Cache-Control tells browsers how long they can keep things.
ETag works like a price tag on items in a store. If the tag hasn’t changed, the browser knows it can use what it saved.
For the best results, save unchanging files for a long time. But for things that might change (like your main page) tell browsers to check for updates more often.
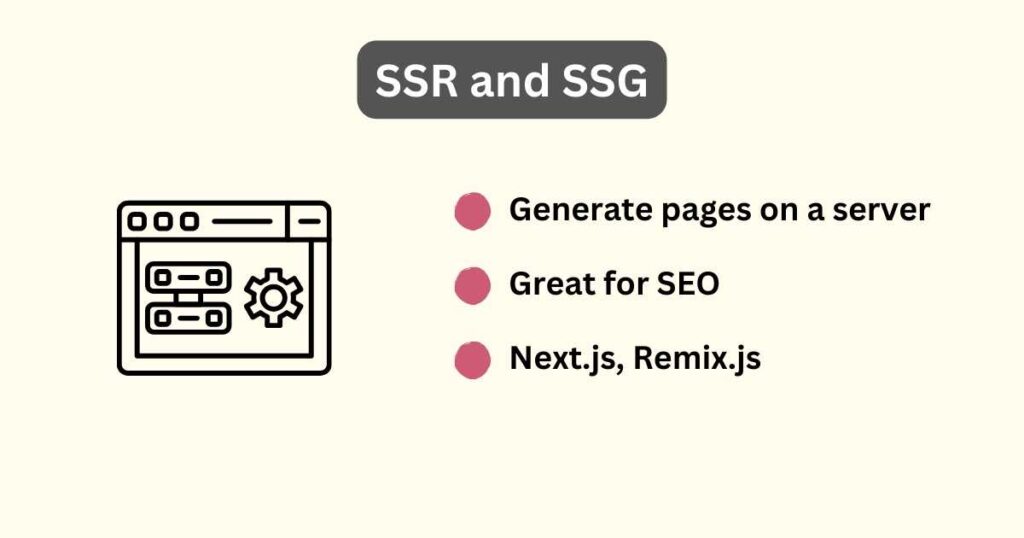
10 SSR or SSG: Front End Optimization Techniques
Server-Side Rendering (SSR) is like a chef cooking your meal when you order it. The server makes the page fresh each time someone asks for it.
Static Site Generation (SSG) is more like meal prep on Sunday for the whole week. Your pages are made ahead of time and ready to serve instantly. This makes your website super fast because everything is ready to go.
Regular websites (CSR) are like getting ingredients and cooking at home. Your browser has to do all the work, which is slow and BAD for search engines.
Next.js and Remis.js are popular tools that lets you use both SSR and SSG.
Modern tools let you mix and match. Some pages can be super fast with SSG, while others stay fresh with SSR.
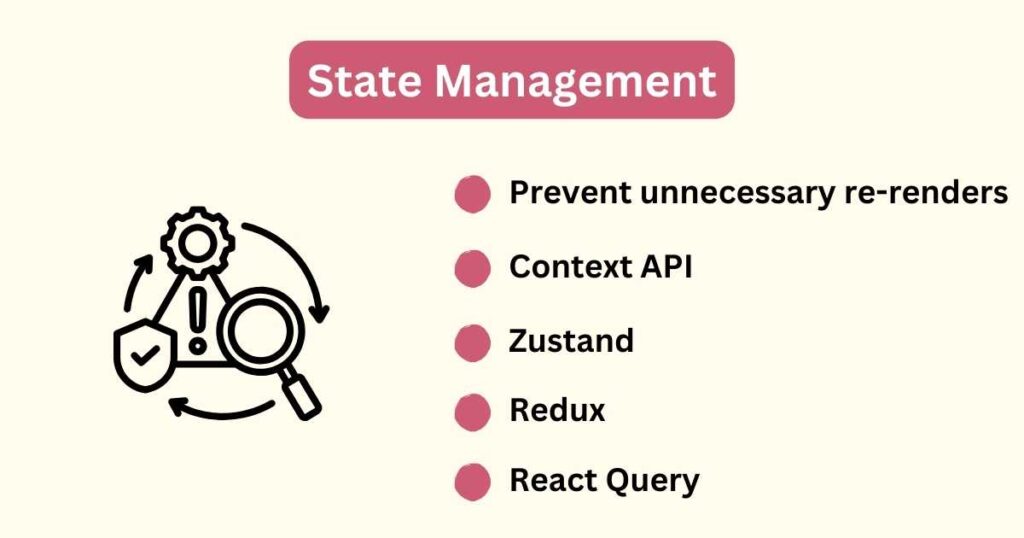
11 State Management and Data Fetching
State management tools are different types of storage boxes fir you app’s data.
Poor state management triggers unnecessary re-renders across your application. When state (data) changes, React.js re-renders components that depend on that state. If your state structure isn’t optimized, even small changes cause large component trees to re-render.
Keep state as local as possible to minimize unnecessary re-renders. Split large components into smaller ones to better manage state updates. Use appropriate tools (Context API, Zustand, Redux) and implement proper caching strategies.
Tool | Setup Effort | Bundle Size | Performance | Best For | Learning Curve |
---|---|---|---|---|---|
Context API | Very Easy | 0KB (built-in) | Poor for large apps | Small apps, simple state | Very Easy |
Zustand | Easy | 4KB | Excellent | Medium apps, quick setup | Easy |
Redux | Complex | 42KB | Good | Large apps, big teams | Hard |
Modern tools — like React Query — improves performance through smart caching. They cache server data and dedupe network requests.
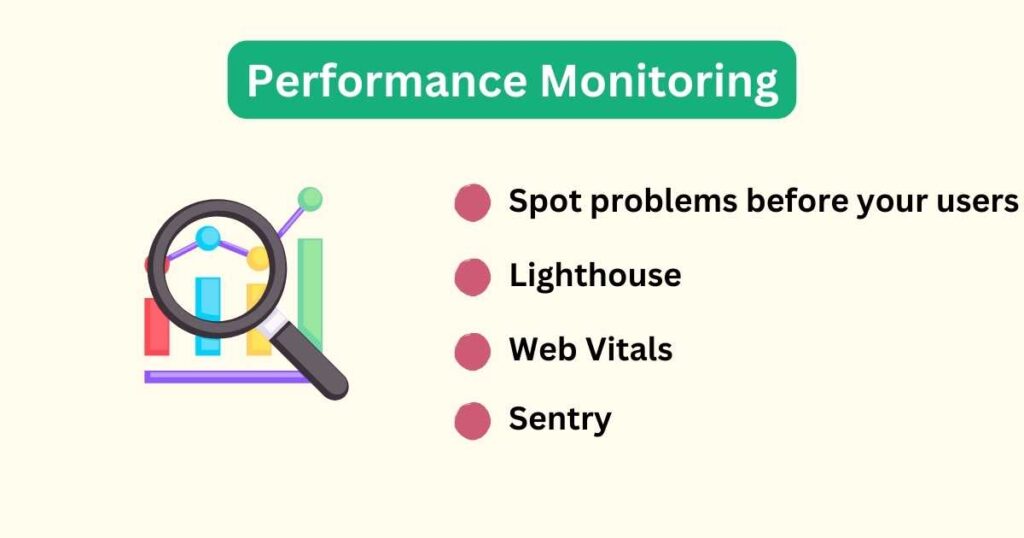
12 Implement Performance Monitoring
Performance monitoring let you have a health check-up for your website. It helps you spot problems before your users do.
Without monitoring, your website could be slow and you wouldn’t know it.
Popular tools
Lighthouse — a popular tool — checks your site and gives you a score from 0 to 100. You can find it right in your Chrome browser.
The Web Vitals extension — another popular tool — shows you how your site performs as people use it. It’s great for catching problems that happen while people browse around.
Sentry helps catch mistakes in your code before they cause trouble.
LogRocket records how people use your site. When someone has a problem, you can watch a video of what happened.
Conclusion: Front End Optimization Techniques
Making your website faster isn’t just about better user experience – it’s about getting hired too! Modern tech interviews often focus on these optimization techniques.
Front-end optimization techniques help your website load faster and run smoother. This means happier users and better search engine rankings. Plus, knowing these skills makes you more valuable as a developer.