Having switched from retail to tech, I remember struggling with this question. Many of my students ask, “What does a front end developer do?” Through years of web dev experience and teaching at bootcamps, I can tell you it’s much more than just writing code…
Front end developers build everything users touch in web applications. If you’ve ever clicked a button, filled out a form, or scrolled through a website — you’ve experienced front end development in action.
In this post, I’ll break down:
- What front end development actually means in 2025
- Common tasks at tech companies
- What employers look for (hint: it’s not just coding skills)
What Is Front End Development
Front end development creates the interactive elements users see and interact with on websites and applications.
Think of it as building the digital storefront of the internet — everything from buttons and menus to forms and animations.
Front-end developers act as translators, turning design mockups into functional interfaces. We collaborate with designers to ensure the app visually appeals and works efficiently.
Companies want developers who can create smooth, fast experiences for their users. Front end developers make technology useful for real people. It’s where code meets human needs. Find here “Most Recent Frontend Interview Questions in 2025 With Asnwers“
Modern front end development uses tools like React, TypeScript, and CSS frameworks. But don’t worry — you don’t need to learn everything at once. I tell my students to start with the basics: HTML, CSS, and JavaScript. Everything else builds on these foundations.
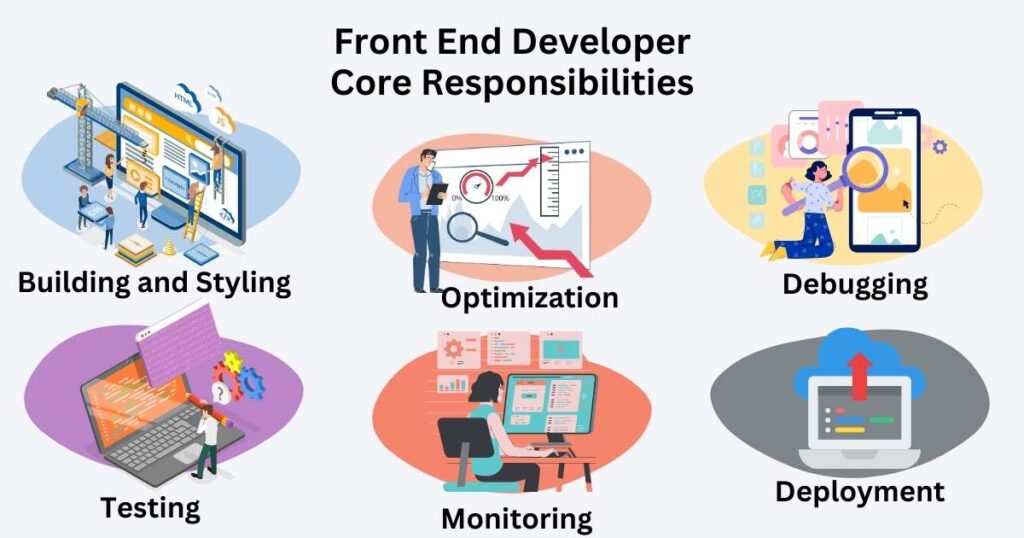
What Does a Front End Developer Do — Core Responsibilities
I switched from retail to tech years ago, and after teaching hundreds of students, I know exactly what front-end developers do.
Responsibility | Daily Tasks | Tools Used | Key Skills | Real Impact |
---|---|---|---|---|
Building Components | - Creating forms and menus- Styling layouts- Making responsive designs- Building animations | - React/Vue/Angular- CSS/SASS- HTML5- TypeScript | - Component architecture- Responsive design- Cross-browser compatibility | At Adobe, our team rebuilt the image editor UI, improving user engagement by 40% |
Testing | - Writing unit tests- Integration testing- End-to-end testing- Test automation | - Jest- Cypress- Playwright- React Testing Library | - Test planning- Bug identification- Test automation | Found 23 critical bugs before launch at Fox Entertainment using comprehensive testing |
Performance Optimization | - Code splitting- Lazy loading- Caching setup- Bundle optimization | - Webpack- Vite- Chrome DevTools- Lighthouse | - Performance metrics- Loading strategies- Memory management | Reduced load time from 8s to 2s for CapitalGroup's dashboard |
Debugging | - Error tracking- Performance profiling- Browser debugging- Network analysis | - Chrome DevTools- React DevTools- Performance Monitor- Error tracking tools | - Problem-solving- Root cause analysis- Performance profiling | Fixed a memory leak affecting 100k users in a streaming service |
Deployment | - Build configuration- CI/CD setup- Version control- Environment management | - Git- Jenkins/GitHub Actions- AWS/Vercel- Docker | - DevOps basics- Build processes- Deployment strategies | Automated deployment reduced release time from 2 days to 2 hours |
Building and Styling Components
We make the parts of websites you use every day. Every button, menu, and form needs careful planning and implementation.
At Fox, I built forms that millions of people use. Each form had to work on phones, tablets, and computers.
Testing Our Work
Nobody likes broken websites. That’s why we test everything we build.
Good developers check their work twice. We use special tools to find problems early.
Recently, my team caught a big problem before going live. Our e-2-e tests saved the company from a costly mistake.
Making Websites Fast – Optimization
Speed matters more than ever. Slow websites lose customers fast.
We make our apps load faster by:
- Loading only what users need
- Saving files in the browser
- Making images smaller
- Caching
Debugging and Fixing Problems
Sometimes, web apps break, and we need to fix them. When an app breaks, we find out why. It’s like being a detective for code.
Last month, I helped fix an app that kept crashing. The problem? A tiny mistake in the code.
Putting Websites Online – Deployment
Getting a website online is the final step. Front end developers make this process automatic and safe for launching new features daily.
Getting websites to users safely is crucial. We make sure everything works before launch.
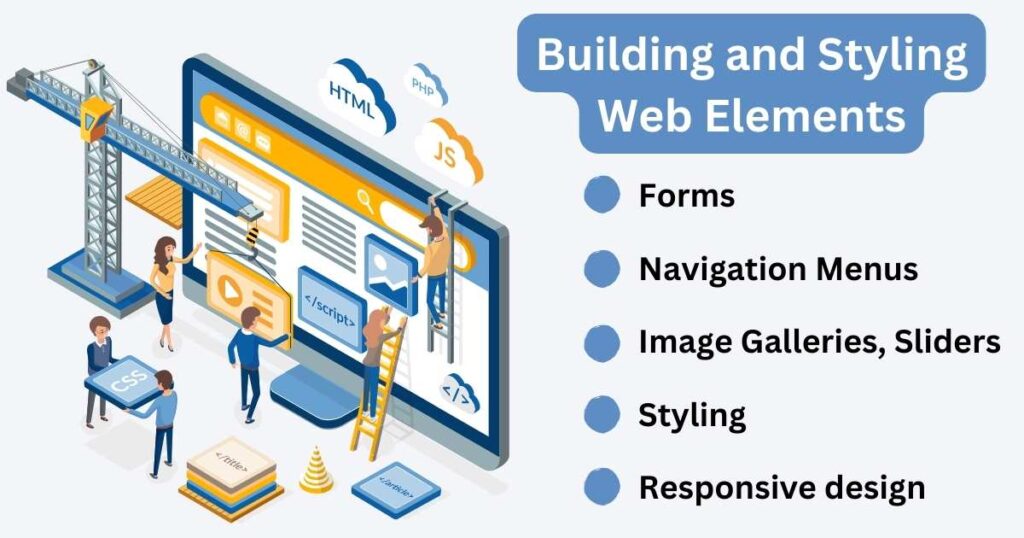
1 Building and Styling Components – What does a front end developer do
A web developer knows what makes web elements work well. And knows how to create them and make them efficient.
Forms
Forms are the heart of most websites. They collect user information and help people take action. Good forms tell users what went wrong and help them fix mistakes.
Forms also save information safely and work with just a keyboard. Users know exactly what to fix when something goes wrong.
Navigation Menus
Think of navigation menus as road signs for websites. They guide users where they need to go.
Good navigation feels natural. Users must find what they need in no time.
During interviews, companies often ask about mobile navigation. I share my experience from Fox, where our menu adjusts its size automatically for different screens.
Image Galleries and Sliders
Picture galleries need to be fast and smooth. We built a gallery that loads images only when needed. For example, load images as users scroll. This makes apps much faster, and users love the smooth experience.
Feature | Before | After |
---|---|---|
Loading Speed | 5 seconds | 1 second |
Image Quality | Blurry | Crystal Clear |
Phone Performance | Crashes | Smooth |
Mobile-First Design
Today, phones matter most. Most people now use phones to visit websites. Many enterprises design everything for small screens first.
Mobile-first websites naturally perform better because they:
- Work smoothly on slower connections
- Load faster on all devices
- Use less data
Accessibility
Websites should work for all people. Good accessibility helps thousands of people use our products.
We make sure everyone can use our site. Whether using a keyboard, screen reader, or mouse, every user mattered. Colors must be easy to see, and text must be clear to read.
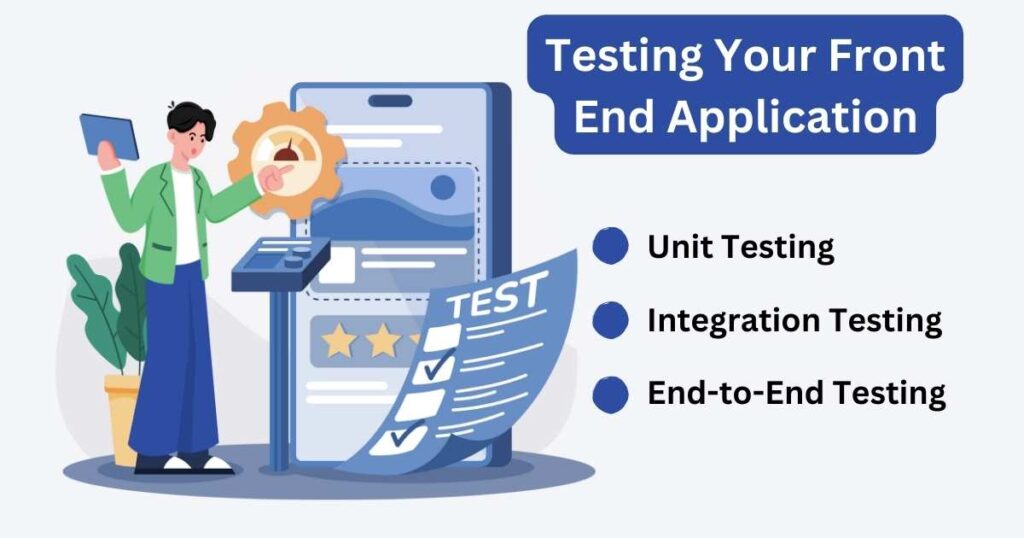
2 Testing Your Front End Application – What does a front end developer do
Testing isn’t just about finding bugs — it’s about confidence in your code.
Think of testing like checking a new car. Unit tests check each part separately, like testing just the brakes. Integration tests make sure parts work together, like the engine and transmission. End-to-end tests drive the whole car to make sure everything works.
Testing Level | What It Tests | Time to Fix Issues |
---|---|---|
Unit | Single functions | Minutes |
Integration | Component groups | Hours |
End-to-End | Full features | Days |
Unit Testing
Unit tests check small pieces of code. Think of building a bike – you check each part before assembly.
Unit tests help us:
- Find bugs early in development
- Make sure each function works correctly
- Save time on debugging later
Jest has become the standard for JavaScript testing. Vitest is much faster ( up to 4 times) than Jest while maintaining compatibility with Jest’s API
Integration Testing: Putting Pieces Together
Integration tests check how parts work together. You can catch a bug between your login and payment systems.
Integration tests:
- Detect interface issues early
- Ensure component compatibility
- Improve overall software quality
At Fox, we relied heavily on Postman for API testing. It validated complex APIs across teams in large projects.
End-to-End Testing: The Full Journey
E2E tests are like test-driving a car. They check everything from start to finish.
E2E testing matters because it:
- Identifies system bottlenecks
- Ensures seamless user experiences
- Reduces time to market
Cypress offers real-time testing feedback and excellent debugging tools. Use Cypress for end-to-end testing because it runs tests in the ACTUAL browser environment.
Playwright is a good choice for cross-browser testing. It supports multiple browser engines, including Chrome, Firefox, and Safari.
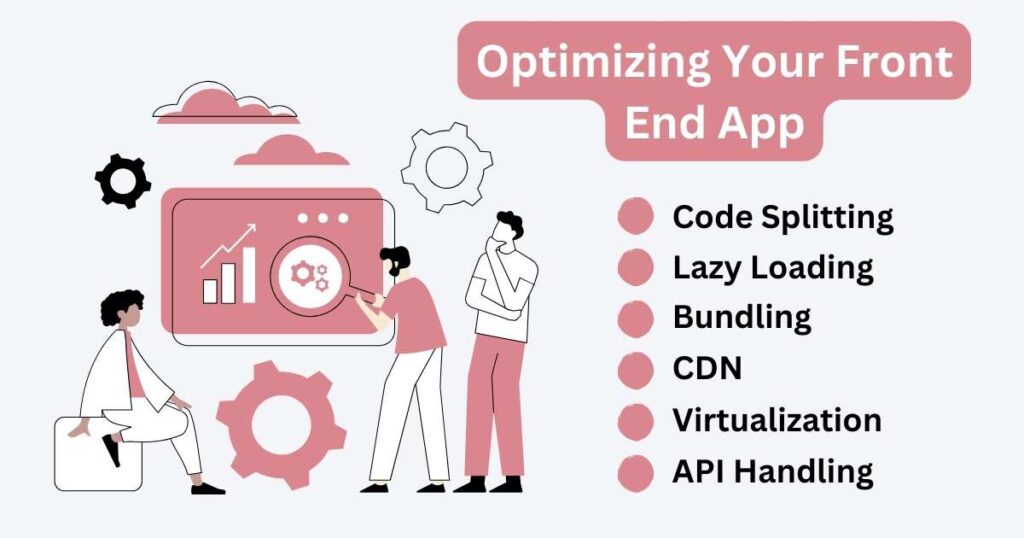
3 Optimizing Your Front End Application – What does a front end developer do
Applications optimization is what really makes websites fast. These are the six most effective techniques we use:
Code Splitting: Breaking Down Your App
Think of code splitting like chapters in a book. Instead of loading the whole book at once, users only get the chapter they’re reading.
Modern tools like Webpack and Rollup handle code splitting automatically. Newer frameworks like Next.js and Remix include it by default
At Fox Entertainment, we split our video player code from the main website. This made the homepage load 60% faster. Users only downloaded the video player when they wanted to watch something.
Before Splitting | After Splitting |
---|---|
8s load time | 3s load time |
2MB download | 800KB download |
Frustrated users | Happy users |
Lazy Loading
Lazy loading means waiting to load things until they’re needed. For example, use this for images below the screen. Load pictures only when users scroll down to see them.
For tools, we use Intersection Observer API for images and React.lazy() for components. Modern browsers support native lazy loading with the ‘loading=”lazy”‘ attribute. For more control, libraries like react-lazyload provide advanced features.
Lazy loading works great for:
- Image galleries
- Dashboard widgets
- Comment sections
- Video players
Bundling
Bundling combines many files into optimized packages. We use tools like Webpack and Vite to create efficient bundles.
A proper bundling can reduce your JavaScript size by 60%! You can organize your platform into:
- Essential features (loads first)
- User preferences (loads second)
- Advanced features (loads when needed)
Modern bundlers like esbuild and SWC make this process incredibly fast. They can process thousands of files in milliseconds.
CDN
Content Delivery Networks (CDN) store your site’s content worldwide. We use Cloudflare and Akamai to serve content from locations close to users. This made our websites faster globally.
Tools like Cloudfront provide detailed analytics about content delivery. This helps identify and fix performance bottlenecks.
Table Virtualization: Handling Big Data
Table virtualization (including Virtual scrolling) renders only visible rows in large datasets. Using react-window and react-virtualized, we handled tables with 100,000 rows smoothly.
These tools automatically manage memory and performance. They’re essential for data-heavy applications.
Smart API Handling: Dedupping Requests
Modern tools like React Query and SWR make API handling efficient. They cache responses, deduplicate requests, and update data automatically.
These tools also provide real-time updates and offline support.
API caching:
- Makes the app feel faster
- Saves repeated (dedup) requests
- Reduces server costs
4 Processing Async Data
Think of async operations as ordering food at a restaurant. You place your order (make a request) and wait while doing other things. When your food arrives (data returns), you handle it. The chef (JavaScript) handles multiple orders (operations) at once without getting stuck on any single task.
Every modern website uses async operations. Social media feeds load as you scroll. Maps load location data in the background. Shopping carts update without page refreshes.
In recent interviews, companies asked about handling race conditions and canceling stale requests. These scenarios happen daily in production applications.
Third-Party APIs
Modern apps connect with many external services. Payment systems handle double charges. Authentication services control token management. We also integrate analytics, ads, and content delivery networks. Each integration needed robust error handling and retry logic.
Common 3rd Party services:
- Payment processing for subscriptions
- Video content delivery networks
- Analytics for user behavior
- Authentication systems
- Cloud storage services
- Social media sharing
- Professional printing services
- Font libraries
Third-party integrations transformed our applications. Data providers give users real-time market information. Authentication services protect sensitive data. Analytics help us understand user behavior and improve features.
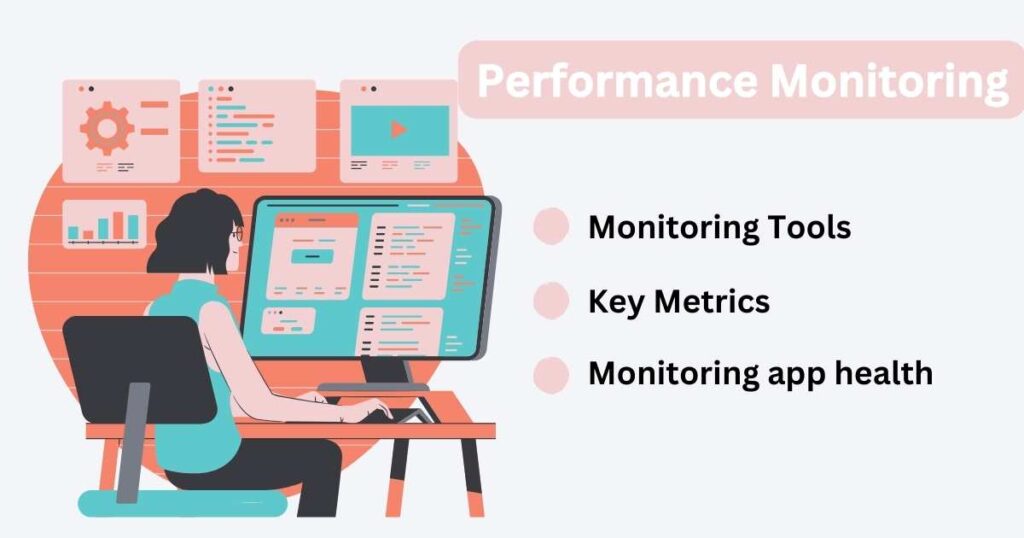
5 Performance Monitoring
Metrics matter for keeping websites fast and reliable.
Think of monitoring as your website’s health dashboard. Each metric has a specific purpose, like different instruments in a car’s dashboard.
Monitoring Tools
Different tools serve different monitoring needs. We use multiple tools to get a complete picture of our app’s health.
Tool | Primary Use | Key Strength |
---|---|---|
New Relic | System Performance | Full-stack monitoring |
Google Analytics | User Behavior | Traffic patterns |
Datadog | Distributed Tracing | Anomaly Detection |
Sentry | Error Tracking | Real-time error alerts |
New Relic works like a complete diagnostic system. IT can track everything from server response times to database queries.
Datadog does real-time monitoring and end-to-end request tracing. Its automated anomaly detection helps prevent many potential issues.
Sentry captures application errors, crashes, and performance metrics in real time. It provides context:
- Stack traces
- Environment variables
- User session data.
Key Metrics
Different applications need different monitoring. E-commerce sites need quick page loads and smooth checkouts. Content sites need fast initial loads and smooth scrolling. Social platforms need real-time updates and instant interactions.
Page load time directly affects user satisfaction. In my projects, we saw a 30% drop in users when pages took more than 3 seconds to load
MonitorAPI response! When response times exceed 200ms, customer satisfaction drops significantly!
6 State Management
Think of state management tools like different types of storage systems. State management directly affects user experience.
Tool | Best For | Learning Curve | Team Size | Setup Time | Debug Tools |
---|---|---|---|---|---|
Redux | Large apps | Steep | Large teams | Hours | Excellent |
Context API | Small/Medium apps | Gentle | Small teams | Minutes | Basic |
Zustand | Modern apps | Moderate | Any size | Minutes | Good |
Redux
Redux works well for large applications. We use Redux to manage complex data across hundreds of components. Its predictable state updates made debugging easier.
Context API
React’s Context API suits smaller applications perfectly. One of my student’s portfolio sites uses Context API for theme switching and user preferences. Simple, practical, and built into React!!
Zustand
Zustand represents the future of state management.
Zustand combines Redux’s power with Context API’s simplicity. Switching from Redux to Zustand makes the code cleaner and easier to maintain.
Conclusion: What Does a Front End Developer Do?
Now we know “What does a front end developer do”!! Front end devs combine technical skills, creativity, and business understanding.
Modern front-end development goes far beyond writing code. Performance, accessibility, and user experience all matter equally.
Ready to start learning? Start small but think big. Begin with core technologies like HTML, CSS, and JavaScript. And read my “How To Get Into IT Without a Degree in 2025” guide.