As someone who’s participated in over 100 frontend interviews in the last 4 years (and been on both sides of the table), I can tell you that frontend interview questions have evolved.
Just in the past 2 months, I’ve gone through dozens of technical interviews, and I’ve noticed some clear patterns.
What Frontend Interview Questions To Expect
Gone are the days of pure algorithmic puzzles and trivia questions – modern front-end interviews focus on practical skills and real-world problem-solving.
Frontend interviews today typically break down into three main components:
- 50% practical coding challenges
- 25% system design discussions
- 25% cultural fit conversations
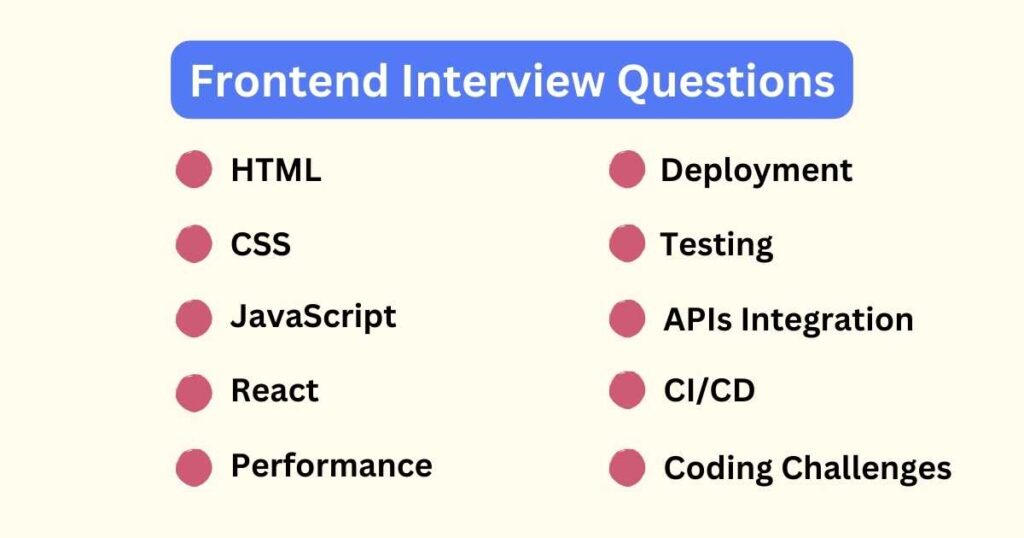
Common Technical Areas
The technical portion usually covers React, JavaScript, and CSS fundamentals. You’ll likely answer frontend interview questions about:
- performance optimization
- API interactions
- error handling
- React-related (Vue or Angular if your stack uses them) question like useEffect implementation and prop drilling
- testing
- debugging
Don’t know how to find interviews? Read my “How To Get Into IT Without a Degree in 2025 Guide“
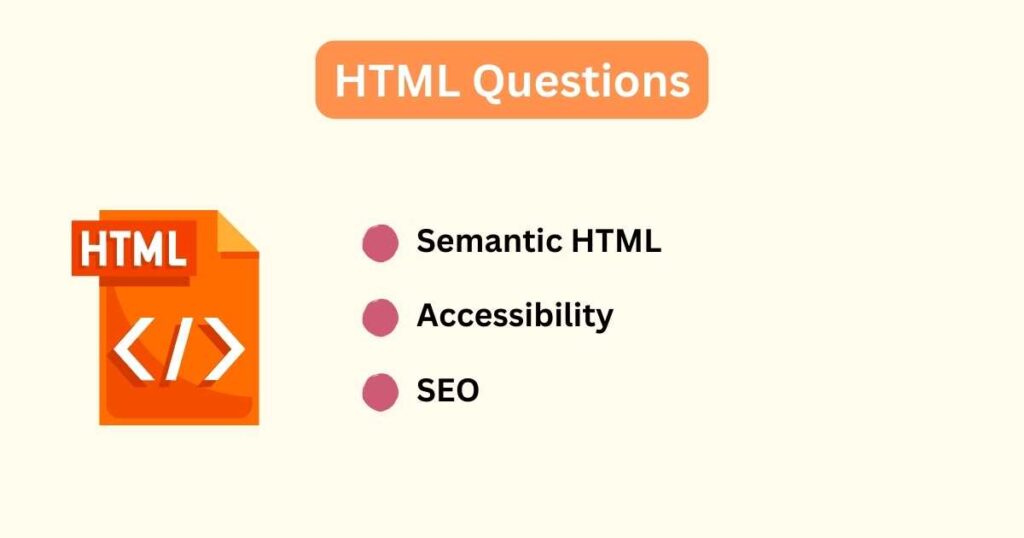
HTML Frontend Interview Questions
In my recent technical interviews, I’ve noticed a focus on semantic HTML questions. Q: “Can you explain what semantic HTML is and when to use it?”
In my experience, there are two main reasons why choosing the correct HTML tags matters: SEO and accessibility.
SEO Impact of Semantic HTML
Search engines rely heavily on proper HTML structure to understand your content. Here’s a real example I encountered during a recent code review:
<!-- Poor SEO Structure --> <div class="header"> <div class="title">Main Blog Post</div> </div> <div class="content"> <div class="article">Content here...</div> </div> <!-- SEO-Friendly Structure --> <header> <h1>Main Blog Post</h1> </header> <main> <article>Content here...</article> </main>
When using generic <div>
tags, search engines struggle to identify the importance and relationship of content. Using semantic tags like <header>
, <main>
, and <article>
helps search engines prioritize and categorize your content correctly.
Accessibility
Screen readers and assistive technologies depend on proper HTML semantics. Here’s how different approaches affect accessibility:
Semantic Element | Non-Semantic Alternative | Impact on Accessibility |
---|---|---|
< nav > | < div class="nav"> | Screen readers can't identify navigation |
< button> | < div onclick=""> | Keyboard navigation breaks |
< h1> to < h6> | < div class="heading"> | Content hierarchy is lost |
Remember, semantic HTML makes your content accessible to everyone and improves your site’s visibility in search results.
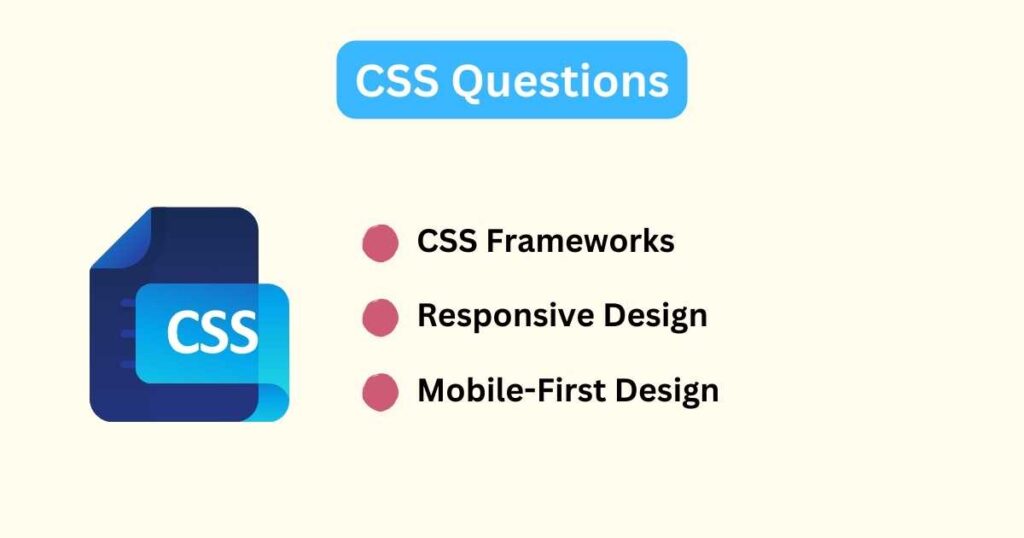
CSS and Styling Frontend Interview Questions
In my recent technical interviews across the Bay Area, I’ve noticed a shift away from writing raw CSS. Instead, interviewers focus on understanding fundamental concepts and practical experience.
CSS Frameworks
Most frontend interview questions focus on practical experience with:
- Bootstrap’s responsive classes (col-sm, col-md, etc.)
- Styled Components for component-based styling
- CSS Modules for scoped styles
- Tailwind CSS for utility-first approach
One of the most frequent questions is about Bootstrap’s 12-column grid system. To answer this, I explain:
- The page width is divided into 12 equal columns
- Elements can span any number of columns
- Column spans determine width percentage (e.g., 6 columns = 50% width)
Responsive Design
Q: “How do you approach responsive design?” – This topic comes up when companies care about mobile UX.
Responsive design makes websites work well on all screen sizes. To do so, we use media queries to target specific screen sizes and adapt CSS based on device screen size.
Images and videos need different sizes for mobile devices.
To test Responsive Designs, I use a combination of:
- Performance monitoring across breakpoints
- Browser DevTools
- Real devices for actual UX
- Automated testing
Mobile-First Design
Interviewers often ask about the mobile-first approach. Mobile-first design means starting your development process for the smallest screen first (mobile phone), then adding support for larger devices.
We design for small screens first because it:
- Forces content prioritization
- Results in lighter, faster-loading pages
- Improves SEO performance
Desktop-First | Mobile-First |
---|---|
Start with full features | Start with core features |
Use max-width queries | Use min-width queries |
Graceful degradation | Progressive enhancement |
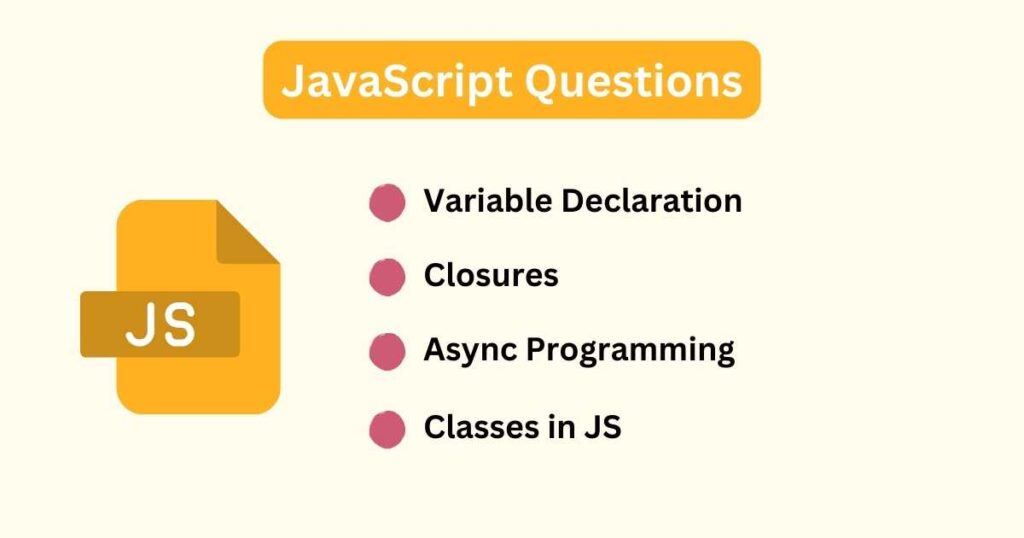
JavaScript Frontend Interview Questions
Now, I share the JS-related questions I’ve personally faced recently. I’ve noticed certain JavaScript concepts appear consistently.
Variable Declaration
I was asked multiple times to explain the differences between variable declarations. In short, var, let and const are different ways to declare variables, each with unique scoping rules and behaviors.
Desktop-First | Mobile-First |
---|---|
Start with full features | Start with core features |
Use max-width queries | Use min-width queries |
Graceful degradation | Progressive enhancement |
Closures
Interviewers usually want a demo of how closures access the outer scope even after the outer function has completed execution.
function createCounter() { let count = 0; return { increment: () => ++count, getCount: () => count }; } const counter = createCounter();
In this example, when you call createCounter
, JS creates a new scope for count
. The returned methods can access count
, even after createCounter
ends. This happens because closures allow inner functions to remember outer variables.
Async Programming
Async programming and Promise handling are probably some of the most frequently asked frontend interview questions in JS tech round.
In all three latest interviews, I was asked to explain Promises and demonstrate proper error handling. The best practices would be implementing timeout mechanisms for long-running operations (like a form field debounce) and custom Promise rejection scenarios (like a HTTP request wrapper).
To answer about async coding, you can also implement a sleep() funciton. Find examples in my “JavaScript Sleep Function — Best 7 Examples To Delay, Pause, and Revoke JS Code in 2025” guide
Async Related Interview Questions:
- How would you implement a timeout for async operations?
- What’s the Promise.all() and how you work with it?
- How do you handle multiple errors in a Promise chain?
In a recent interview at a startup, I was asked to implement a delayed greeting function. Here’s the solution that got me to the next round:
async function delayedGreeting(name) { try { await new Promise(resolve => setTimeout(resolve, 2000)); return `Hello, ${name}!`; } catch (error) { console.error('Error in greeting:', error); } }
Classes in JavaScript
A popular frontend interview question: “Create a User class with name and role properties, and add a method to display user information.”
My solution:
class User { constructor(name, role) { this.name = name; this.role = role; } getInfo() { return `${this.name} works as ${this.role}`; } } const developer = new User("Eugene", "Frontend Developer"); console.log(developer.getInfo()); // "Eugene works as Frontend Developer"
The constructor runs when we create a new object. It sets up the initial User values. Methods like getInfo can access (or modify) the object later.
Classes in JavaScript are templates for creating objects. They were introduced in ES6 to make object-oriented programming easier. But under the hood, they still use JS prototype inheritance.
What Interviewers Look For:
- Proper constructor implementation
- Correct use of this keyword
- Method definition within the class
- Understanding of instantiation
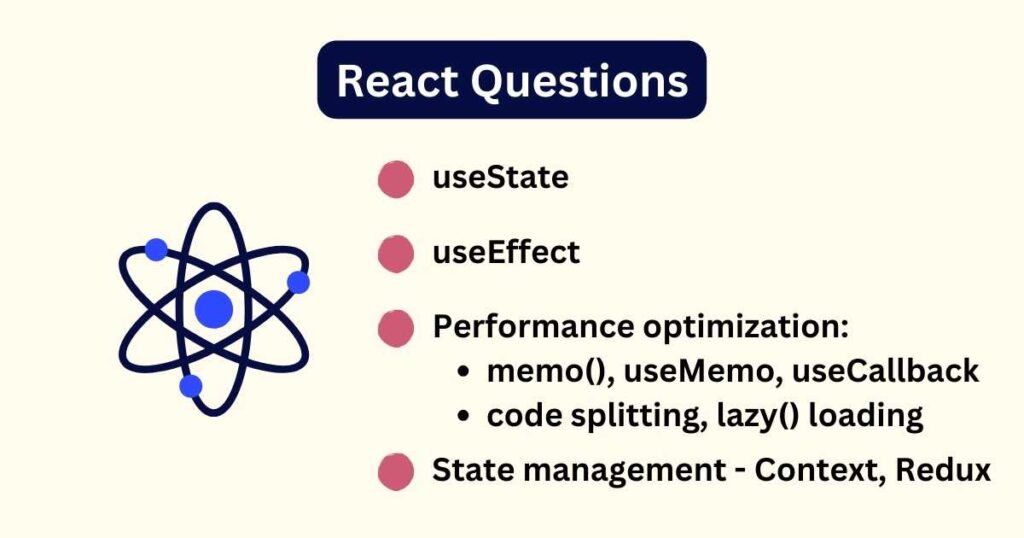
Frameworks and Libraries: React Focus Questions
Most React-related frontend interview questions revolve around state management and hooks.
useState Implementation
Expected question: “Explain useState and provide an example of proper implementation”.
I like to think of it like a component’s memory. When this memory changes, React updates what we see on the screen. useState is a React Hook that lets components remember information.
Key points to address:
- State immutability principles
- Functional updates for state changes
- Batching behavior in React 18
const [count, setCount] = useState(0); // Good Practice const handleIncrement = () => { setCount(prevCount => prevCount + 1); // Using callback for state updates }; // Bad Practice const handleIncrement = () => { setCount(count + 1); // Direct state mutation };
useEffect
The most common useEffect-related question is “What is useEffect, and when do you use it?”. In your answer, cover side effects, data fetching, and DOM manipulation.
Important to know:
- Proper dependency array usage
- Cleanup function implementation
- Understanding of mounting/unmounting behavior
Always implement cleanup functions for subscriptions. Have one error boundaries implementation (pick your favorite) and be ready to code it in an interview.
Another popular question is: “Explain the difference between useState and useEffect”. Learn when and why to use these different patterns.
React Performance Optimization
React.memo is crucial for preventing unnecessary re-renders. This is effective for static components that receive the same props frequently but don’t need to re-render.
I explain how I use useMemo for expensive calculations and to prevent unnecessary recalculations. For function memoization with useCallback, I discuss how to avoid unnecessary re-renders and stabilize child components. Read my “React useCallback Hook: Super Easy Guide in 5 Min!” for more examples.
Hook | Use Case | Common Mistakes |
---|---|---|
useMemo | Expensive calculations | Over-optimization |
useCallback | Function memoization | Incorrect dependencies |
memo | Component re-render prevention | Wrong comparison implementation |
Another popular production technique is code splitting. Code splitting breaks applications into smaller chunks to reduce initial load times. A popular pattern — route-based code splitting. Other common strategies are component-based splitting (for large features) and dynamic imports (for heavy modules).
Lazy loading for images and components not immediately needed improves initial page load times, too. These techniques are effective in projects with heavy data computation or compound/complex UI.
React State management
“Talk about useState vs Context API vs Redux” – These are common frontend interview questions. Interviewers expect an understanding and clear examples of when to use each one.
State Management Tool | Use Case | Complexity Level |
---|---|---|
Local State | Component-specific data | Low |
Context API | Shared app-wide state | Medium |
Redux | Complex global state | High |
When discussing React state management in interviews, I always start with the basics:
- useState for simple component state
- useReducer for complex state logic
- Component composition for shared state
Context API is the best for simpler state management scenarios. I find Context API particularly effective for managing application-wide preferences and settings.
We bring in Redux when dealing with large-scale applications. Primarily when multiple team members work on the same codebase. Redux provides a strict data flow for complex data states.
When implementing Redux in large applications, I focus on:
- Single source of truth
- Immutable state updates
- Action creators and reducers
- Middleware for side effects
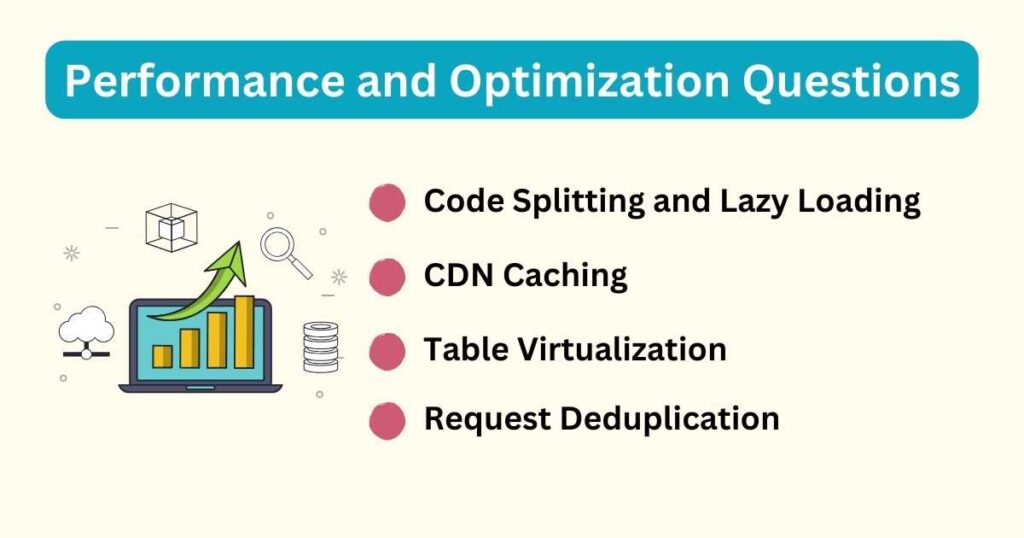
Performance and Optimization Frontend Interview Questions
Performance questions now take up almost half of each technical interview. I’ve never seen such a focus on performance before.
Companies care more about speed than ever. Just last week, three different interviews focused mainly on performance. They asked about load times, rendering speed, and code optimization.
Q: “How do optimize React applications?”
To answer this one, I measure real user experience. Use Web Vitals to see how quickly main content loads or racks visual stability. Optimize images (including sizes) and reduce server response time to improve UX.
Code Splitting and Lazy Loading
I love lazy loading (React.lazy) for routes, meaning pages load only when users visit them. You can load the main app quickly and then load heavy elements when needed.
Some interviews start with code-splitting questions. I explain that with code splitting, users load pages faster. You can split complex data grids load while scrolling or smooth transitions of heavy charts.
Mention a bundle size optimization through tree shaking and dependency management.
CDN Caching
CDN caching (Fastly, Akamai, Cloudflare) stores your static assets closer to users, making apps load faster. Usually, we cache our JavaScript bundles, CSS files, images, and videos at edge servers.
CDNs distribute content across multiple servers worldwide. This means users from different locations get faster access to our application.
CDN Caching Best Practices:
- Configure proper cache headers
- Implement version-based cache-busting
- Monitor cache hit ratios
- Use proper invalidation strategies
Table Virtualization
This topic appears in frontend interview questions for applications dealing with large datasets. Table virtualization renders only visible rows and columns, improving performance.
Table virtualization is not always necessary for smaller tables. Be ready to explain when and why to use virtualization.
Use virtualization when dealing with:
- Tables with more than 50 rows
- Many columns (horizontal virtualization)
- Frequent data updates
- Limited device resources
Request Deduplication
Request deduping is important when dealing with modern web applications that make numerous API calls. You may discover that your app is making the same API calls multiple times unnecessarily. Without proper deduplication, we face increased server load and higher API costs!
A solution is to use automatic request deduplication. I have experience Implementing React Query to solve these issues.
Request deduping creates a more efficient, responsive application. Understand when and how to implement deduplication effectively.
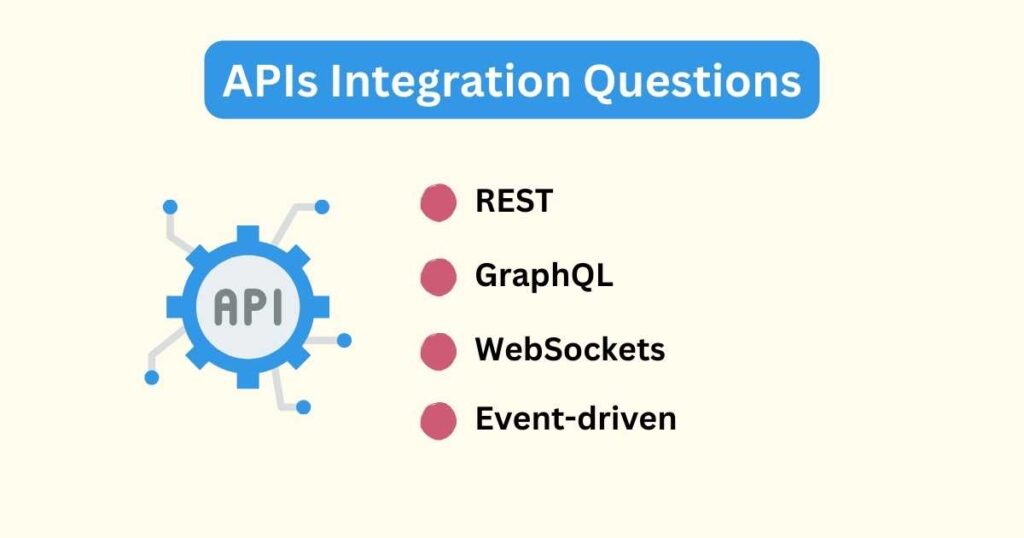
APIs Integration
Each API architecture — REST, GraphQL, WebSocket, and Event-Driven — serves different purposes and solves specific problems.
REST works best for simple CRUD operations and public APIs. We use it when endpoints are straightforward, and data needs are predictable.
Use GraphQL for complex data requirements. It helps mobile apps reduce data transfer and gives clients more control over data.
WebSockets are great in real-time features (like chat systems or live updates). They maintain an open connection for instant data transfer.
Event-driven architecture works on a publish/subscribe model. I used this for our notification system. It helps when multiple services need updates about certain events.
Always test your API calls thoroughly. Make sure they work even when the internet is slow. Keep all your API endpoints in one place for easier maintenance.
Watch for errors and problems. If too many errors happen — the system alerts your team. Have a backup plan for when APIs fail. Show users cached data (if possible) and let them know if something is wrong.
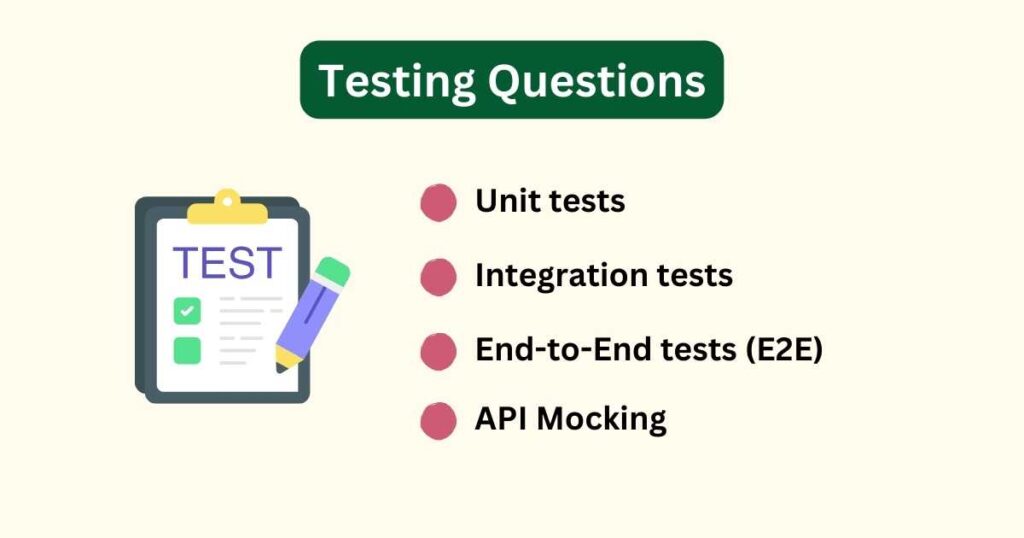
Testing
Testing is a crucial topic, especially for senior frontend positions. Testing questions from recent technical interviews.
In the last two months, in every single technical interview, I’ve had at least one testing question.
“What types of tests do you write for React applications?” is a question that comes up in almost every interview. To answer this one, I explain all three: unit, integration, and E2E testing.
Unit tests
We write unit tests for small pieces of code. A unit test tests a single component (module) separately. This helps catch bugs early. Jest makes unit testing easy and fast.
Unit tests verify that inputs work correctly, buttons respond to clicks, and the component renders the expected output. We also test error states and edge cases.
Unit tests tools: Jest and React Testing Library / Vitest/ Mocha and Chai / Jasmine
Integration tests
Integration tests check if parts work together. For example, we test if forms send data correctly. We also test if components share data properly and check the side effects.
Focus on key integrations such as form submissions with API calls and data flow between components.
We test if a search form sends data to a results component. And if filters update product lists correctly. I also verify if error messages show up when they should.
Integration test tools: Enzyme / Cypress / React Testing Library / Playwright / Karma
End-to-End tests (E2E)
End-to-End tests check the whole application. We add end-to-end tests for important user actions.
For example, we test if users can sign up and make payments. E2e test can check the entire checkout process. Make sure users can add items, enter payment info, and complete purchases.
Tools: Cypress / Playwright / Selenium / WebdriverIO / Nightwatch
“How do you approach testing React app?” aka “How do you decide what to test?“
Last week, at a fintech interview, they asked: “How do you approach testing React app?”. I explained that I start testing the most critical parts first. We first test features that directly impact businesses and users.
Money-related features have the highest priority. Payment processing must work every time. Shopping carts should calculate totals correctly. Order submissions must be reliable.
User data also needs careful testing. Login must be secure. User profiles should save correctly. Password changes must work perfectly.
Extra points for mention we focus on testing features that:
- Can lose money if broken
- Handle sensitive user data
- Have complex business logic
- Used most often
API Mocking
“How do you test components that fetch data?” – I usually explain what we mock and stab API calls in unit tests. We mock any external dependencies to keep the tests focused and fast. In e2e tests thought, we use real APIs.
MSW (Mock Service Worker) is one of the most effective solutions for API testing. It intercepts network requests and provides mock responses. Create realistic test scenarios and use mock data that matches the real API structure.
Test loading state, successful cases. Don’t forget error handling and test what happens when the API call fails.
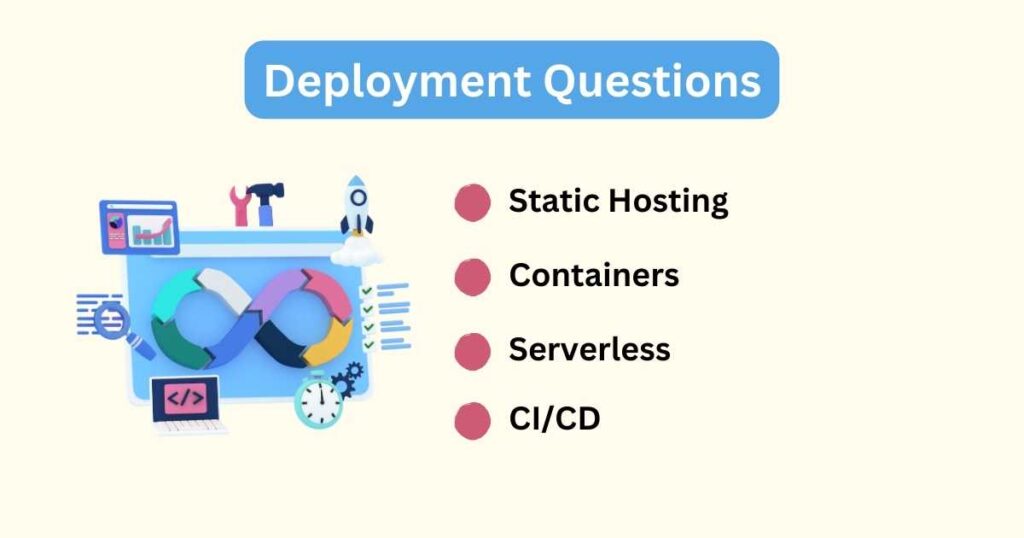
Frontend Application Deployment
“What deployment strategies would you recommend for a React app?”
Static Hosting Approach
For simple React applications, static hosting is the fastest deployment. Static hosting serves pre-built files directly to users through a CDN.
I use Vercel for many static sites. You connect your GitHub repository, and it handles everything else. Vercel provides automatic HTTPS, a global CDN, and instant rollbacks. Netlify is another excellent choice for static sites.
Container-Based Deployment
For larger applications, container deployment offers more control. You can use Docker with AWS. Docker packages your app (and its dependencies) into a standardized unit — a container!
With containers, you configure everything exactly how you need it. The app will run the same way in all environments — test exactly what would run in production.
Use environment variables properly and never commit sensitive data.
Serverless Deployment
Serverless means running your app without managing servers directly. The cloud provider handles all server management instead.
AWS Amplify and Vercel handle everything well. You don’t need to manage servers while the app scales automatically based on traffic.
With serverless, your costs can decrease because you only pay for actual usage.
CI/CD
Another important topic, especially for senior frontend positions. Almost every company now asks about automated deployments.
“How do you set up CI/CD for a front-end project?“
Continuous Integration (CI) means checking code changes frequently. We push code several times daily. Our tests run automatically with each push. This catches problems early.
Continuous Delivery (CD) means getting code ready for release automatically. When tests pass, our system builds the application. It then deploys to a staging environment.
Jenkins is an open-source veteran. Another popular option is GitHub Actions, which works directly in your GitHub repository. Build a pipeline that:
- Run tests when developers push code
- Check code quality with ESLint
- Build the application if tests pass
A simple pipeline would trigger linting on every pull request. This catches basic issues before code review.
Next, your pipeline runs unit and integration tests. If these pass, it builds the application. The build process optimizes assets and creates production bundles. The pipeline can also run performance checks at this stage.
A robust CI/CD system needs good monitoring. Track build success rates and deployment times. When issues occur — quickly roll back to previous versions.
First Production Deployment
“ What steps do you take for the first production deployment of a React application?” This question comes up in senior developer interviews. Companies want to know if you understand the complete deployment process.
Pre-Deployment Preparation
Choose the right hosting provider – AWS or Azure clouds (for complex apps), Vercel or Netlify. AWS offers more control. Vercel makes deployment simpler.
Then, carefully manage environment variables (ENVs). Separate production credentials from development (including QA and Staging envs) ones. Sensitive data never goes into code repositories. Use secure secret management systems (AWS Secrets Manager).
Security and Performance
Run security scans on all dependencies and check for known vulnerabilities. Review authentication systems carefully.
Do load testing across different devices! Check server response times under load. Give mobile performance some special attention.
Testing Strategy
Every feature needs test coverage. Run end-to-end tests for critical user flows and provide payment processing with extra attention.
Manual test on different browsers and devices. Real users help test the application. Manual QAs find things automated tests miss and usability issues early.
Monitoring and Analytics
Set up proper monitoring to prevent surprises with tools like New Relic or Datadog. These show us how our application performs. They alert us when problems happen.
Google Analytics (or Segment or Adobe) helps track user behavior. We set up custom events for important actions to understand how people use our application.
Launch Day And Post-Launch
Start with a soft launch. A small group of users tries the application first to reduce risk. This helps catch problems before full release.
Launch day checklist:
- Watch server performance
- Verify all environment variables
- Check database connections
- Test payment systems
- Monitor error rates
After launch, watch everything closely. Use error monitoring tools to find problems quickly. Collect user feedback actively to fix issues fast.
Coding Challenges
I’ve seen several challenge types. Some companies ask you to build something from scratch. Others show you broken code to fix. They also can ask you to explain the code snippet and refactor it.
One of the most recent challenges was to build a TypeScript form. This form handles user input and makes API calls. It sounds scary at first, but breaking it into small steps makes it manageable.
Another time, interviewers showed code with performance issues. They wanted to see how I think through problems and optimize the performance.
My Personal Approach
I go into every interview expecting to learn something new. This mindset helps me stay calm. I know that my perfect job is out there somewhere. Each interview brings me closer to finding it.
When I don’t know something, I say it honestly. Recently, an interviewer asked about a technology I hadn’t used. I explained that I’d be excited to learn while I hadn’t used it.
I use platforms like CodeWars to practice coding challenges. Each challenge teaches me something new. The more I practice, the more confident I feel in interviews.
Every interview teaches me something valuable. Sometimes, I learn new technical skills. Other times I improve my communication.
It’s okay to fail some interviews. That’s how we learn and grow. Stay positive and treat each interview as a learning opportunity.
Conclusion
Technical skills matter, but companies look for more than just coding ability. They want developers who can solve real problems.
Performance optimization comes up in almost every interview now. Companies care about fast, efficient applications. They want developers who understand how to make applications run smoothly.
Testing knowledge is crucial. Companies want to see that you care about code quality.
API integration skills matter more than ever. Learn different API types and best practices.
Remember that finding the right job takes time. Each interview brings you closer to your perfect position.