What is TypeScript? Let me share my experience teaching bootcamps and building enterprise applications at Fox, CapitalGroup, and Adobe — it’s the tool that transforms messy JavaScript codebases into robust apps.
The TypeScript ecosystem has evolved since Microsoft introduced it. Its type system has become one of the most available (compared to languages like Kotlin and Swift).
What is TypeScript And Why It Matters in Modern Development
TypeScript is a “strict syntactical superset” of JavaScript that adds optional static typing and class-based object-oriented programming. Its ability to catch errors before they reach production makes it valuable (something I’ve seen save countless hours of debugging in both small projects and large enterprise applications).
Here’s why TS matters:
- Immediate error detection through IDE integration
- Rich autocompletion and code navigation
- Improved refactoring capabilities
Feature | JavaScript | TypeScript |
---|---|---|
Type Checking | Runtime | Compile-time |
Error Detection | During execution | During development |
Refactoring Support | Limited | Comprehensive |
IDE Integration | Basic | Advanced |
In my experience teaching both JavaScript and TypeScript, students who learn TypeScript often develop better coding practices. One of my recent bootcamp graduates noted: “TypeScript forced me to think about data structures before writing code, which made my applications more robust”.
Real-World Impact
The benefits become apparent in larger codebases. The type system becomes invaluable when working on a project with over 300 components and thousands of TypeScript files. TS helps maintain code quality and preserves developer sanity.
TypeScript’s adoption in enterprise environments isn’t just about type safety – it’s about creating maintainable, scalable codebases. Working at CapitalGroup Bank, I saw firsthand how TypeScript’s static typing helped new team members quickly understand and contribute to existing projects.
The language continues to evolve with the 2025 updates that enhanced developer experience and sophisticated type inference capabilities.
Learning Curve
JavaScript offers a gentler entry point for beginners in web development. Its nature lets you code without worrying about type declarations (or complex configurations). In my experience teaching both languages, students typically grasp JavaScript fundamentals within 2-3 weeks.
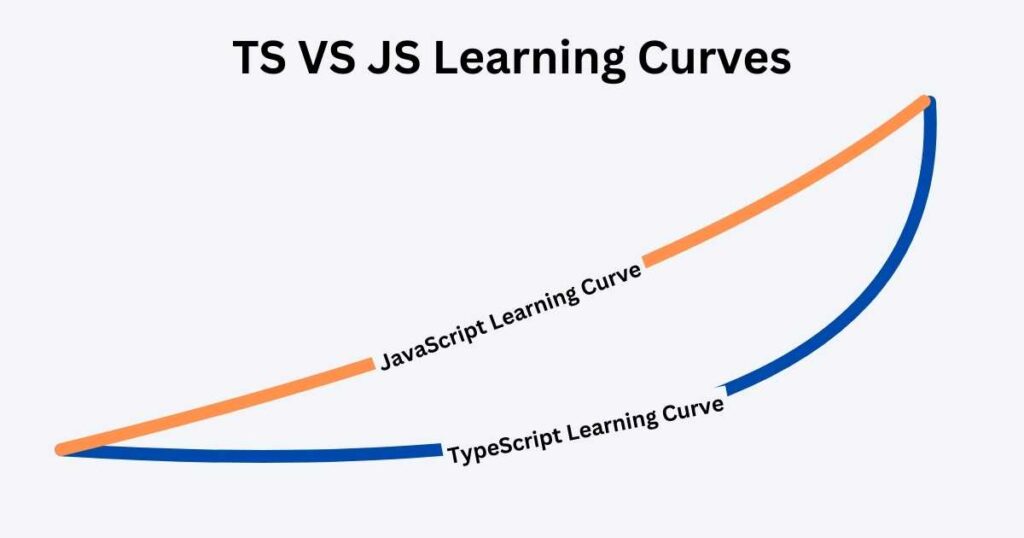
TypeScript’s learning curve is notably steeper for three main reasons:
- Understanding static typing concepts
- Learning interface and type declarations
- Mastering the TypeScript configuration system
However, the investment in learning TypeScript pays (significant) dividends. At FOX, new developers who learned TypeScript became more productive after the first month, making fewer runtime errors.
Compilation Process
The TypeScript compilation process (often called transpilation) converts TypeScript code into JavaScript that can run in any environment.
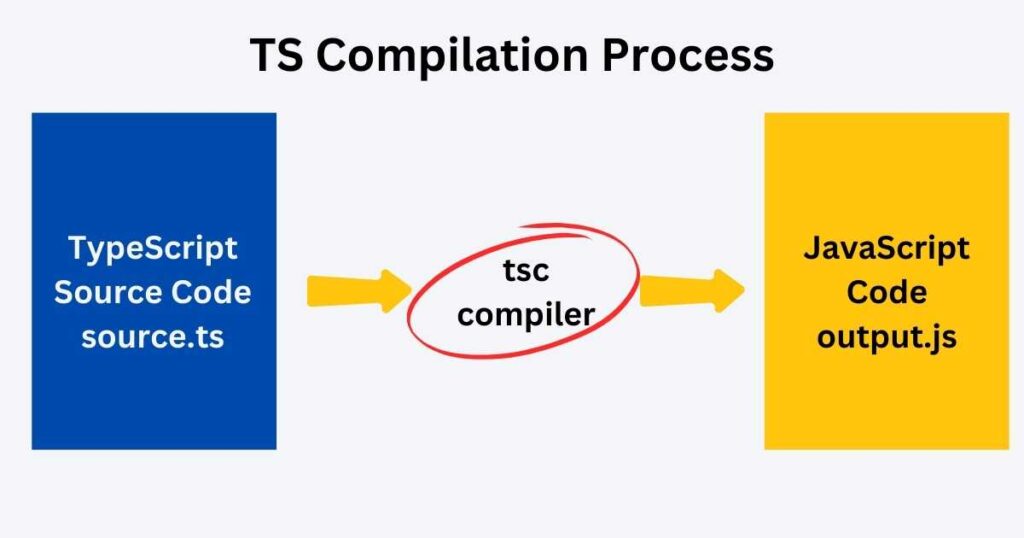
The TypeScript compiler (tsc) transforms your code through several stages:
Feature | JavaScript | TypeScript |
---|---|---|
Execution | Direct browser interpretation | Requires compilation |
Build Process | None required | Needs configuration |
Error Detection | Runtime | Compile-time |
Here’s a practical example of the TypeScript compilation process:
// source.ts function greet(name: string): string { return `Hello, ${name}!`; }
This TypeScript code compiles to JavaScript:
// output.js function greet(name) { return "Hello, " + name + "!"; }
This compilation process (while adding an extra step to development) catches errors early and ensures type safety across the codebase.
Core Features and Benefits
TypeScript’s power lies in its feature set that enhances JavaScript. Through my years of teaching and developing with TypeScript, I’ve seen how these features transform codebases from bug havens into maintainable, scalable applications.
Feature | Benefit | Real-world Impact |
---|---|---|
Static Typing | Catch errors at compile time | Fewer runtime bugs |
OOP Support | Better code organization | Faster onboarding for new developers |
Interfaces | Clear contract definitions | Improved team collaboration |
Generics | Type-safe reusable code | Reduced code duplication |
Modules | Better code organization | Simplified maintenance |
1 Static Type Checking
One of the most powerful features of TypeScript is its static type system. When teaching new developers, I always demonstrate this with a simple example:
function calculateTotal(price: number, quantity: number): number { return price * quantity; } // This will error at compile time calculateTotal("10", 5); // This correct usage calculateTotal(10, 5); // Returns 50
price: number
andquantity: number
— The function parameters are explicitly typed asnumber
, meaning they only accept numeric values.number
(Return Type) — The function is explicitly defined to return anumber
, ensuring type safety for the result.- Logic — The function multiplies
price
byquantity
to calculate the total cost and return it.
In TS, we call functions using the expected types. The type checker catches potential issues before they become runtime bugs.
- Issue: The first argument
"10"
is a String, not a Number. TypeScript will catch this as a compile-time error because it violates the function’s type definition (price: number
). - Error Behavior: TypeScript flags this mismatch BEFORE runtime, preventing potential runtime errors or unexpected behavior in your application. To fix the error, pass a number as the first argument:
typescript:calculateTotal(10, 5)
Errors are caught during development (compile-time) rather than during execution (runtime). In my experience at FOX, this feature alone reduced debugging time by approximately 30%.
Key Benefits of Static Typing:
- Catches type-related errors during development
- Provides better IDE support with accurate autocompletion
- Makes refactoring safer and more efficient
2 Object-Oriented Programming Support
Object-Oriented Programming (OOP) is a programming paradigm that organizes code into objects, which are instances of classes. Each object can contain properties (data) and methods (functions) to operate on that data.
TypeScript’s class-based OOP support brings clarity and structure to JavaScript applications.
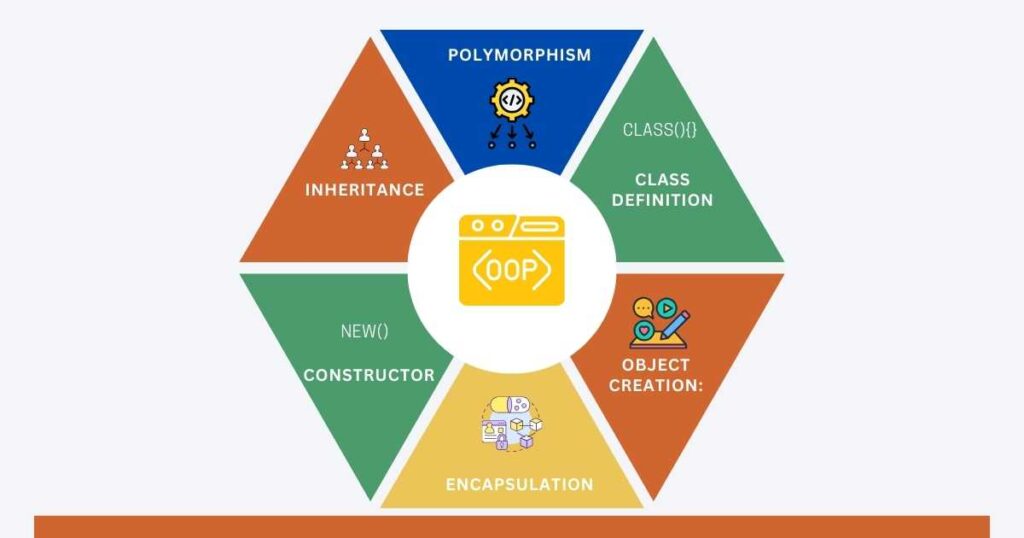
Here’s an example that covers OOP principles — encapsulation, inheritance, and polymorphism — in an approachable way for beginners:
class Person { // Properties private name: string; private age: number; // Constructor constructor(name: string, age: number) { this.name = name; this.age = age; } // Method: Get person details public getDetails(): string { return `${this.name} is ${this.age} years old.`; } } class Student extends Person { private grade: string; constructor(name: string, age: number, grade: string) { super(name, age); // Call parent class constructor this.grade = grade; } // Overriding a method public getDetails(): string { return `${super.getDetails()} They are in grade ${this.grade}.`; } } // Main Program const person = new Person("Alice", 30); console.log(person.getDetails()); const student = new Student("Bob", 16, "10th"); console.log(student.getDetails()); // Optut: // Alice is 30 years old. // Bob is 16 years old. They are in grade 10th.
Class Definition:
- A class in TypeScript defines a blueprint for creating objects.
- Example:
Person
is a class withname
andage
properties and a methodgetDetails
.
Encapsulation:
- Properties like
name
andage
are markedprivate
to restrict direct access from outside the class. This protects the data.
Constructor:
- The constructor is a special method used for initializing class objects.
- In
Person
, it initializesname
andage
.
Inheritance:
Student
extends thePerson
class, inheriting its properties and methods.- It adds its own
grade
property and overrides thegetDetails
method.
Polymorphism:
- The
getDetails
method inStudent
overrides the one inPerson
. - The
super.getDetails()
call accesses the parent class implementation, showcasing polymorphism.
Object Creation:
- Objects are created using the
new
keyword, and methods likegetDetails
are invoked on these objects.
3 Interface Definitions
Interfaces in TypeScript act as contracts that ensure consistency across your codebase. An interface specifies the shape an object must adhere to. It’s like a blueprint for defining properties and their types.
At CapitalGroup, we used interfaces extensively for API integrations:
interface PaymentTransaction { id: string; amount: number; currency: string; status: 'pending' | 'completed' | 'failed'; timestamp: Date; }
The provided code illustrates the use of interfaces in TypeScript, which are essential for defining the structure of objects and ensuring consistency within your codebase. Learn more about TS interfaces in my “TS Record types tutorial“
Explanation:
- Interface Purpose:
- An interface in TypeScript specifies the shape an object must adhere to. It’s like a blueprint for defining properties and their types.
- The
PaymentTransaction
Interface:- This interface defines the structure for a payment transaction object.
- The properties include:
id
: A string representing a unique identifier for the transaction.amount
: A number specifying the monetary value of the transaction.currency
: A string defining the currency type, e.g., ‘USD’ or ‘EUR’.status
: A union type indicating the current state of the transaction; can only be ‘pending’, ‘completed’, or ‘failed’.timestamp
: A Date object capturing when the transaction occurred.
Interfaces are a best practice in TypeScript development reduced integration errors and improved code.
4 Generic Types
Generics enable you to write flexible, reusable (and type-safe!) code. Here’s a practical example from my teaching:
function createState<T>(initial: T) { let value = initial; return { get: () => value, set: (newValue: T) => { value = newValue } }; } const numberState = createState(42); const stringState = createState("hello");
Explanation:
- Function Definition with Generics:
function createState<T>(initial: T)
— TheT
is a generic type parameter. It allows the function to work with any data type (number
,string
,object
, etc.), specified when the function is called.initial: T
means the function expects aninitial
value of typeT
.Internal State Management:
- Using the Function:
const numberState = createState(42)
- Here, the generic type
T
is inferred asnumber
, and the initial state is set to42
. - The returned object can now manage
number
values only.
- Here, the generic type
const stringState = createState("hello")
- Here,
T
is inferred asstring
, and the initial state is set to"hello"
. - This instance manages
string
values only.
- Here,
Generics ensure reusability and type safety, allowing createState
to handle any type. Read more about generics in my “TypeScript Generics Tutorial”
Type inference means you don’t need to explicitly declare the type (TS determines T
automatically by the initial
argument).
5 Module Support
TypeScript’s module system helps organize large codebases effectively. Use the TypeScript modules for organizing and reusing code, specifically in a feature-based architecture (commonly used in front-end applications.
At Fox Entertainment, we structured our applications like this:
features/auth/types.ts export interface AuthState { user: User | null; isLoading: boolean; } // features/auth/hooks.ts import { AuthState } from './types'; export function useAuth(): AuthState { // Implementation }
The features/auth/types.ts
file defines and exports a TypeScript interface, AuthState
, which describes the structure of an object.
The features/auth/hooks.ts
file imports the AuthState
interface and demonstrates a custom hook called useAuth
. This hook would return an object conforming to the AuthState
interface.
TS module support allows group files by features (auth
in this case) to keep the codebase neat. It also lets us encapsulate functionality within a feature
folder.
This modular approach made our codebase more maintainable and easier to navigate for new team members.
Conclusion
TypeScript has evolved from being just a JavaScript superset to an (essential) tool in modern web development. Through my experience teaching both languages, I’ve witnessed their props and cons.
When to Choose TypeScript
TypeScript excels in:
- Large-scale enterprise applications
- Team-based development projects
- Codebases requiring strict maintenance
- Projects using modern frameworks like Angular
When to Stay with JavaScript
JavaScript remains ideal for:
- Rapid prototyping
- Small to medium-sized projects
- Quick iterations
- Learning web development fundamentals
The decision between TypeScript and JavaScript ultimately depends on your project’s scope and team’s expertise. TypeScript’s learning curve is steeper, but it benefits in code maintainability and error prevention.