Learn how to check the GitHub Actions status in your code repository with these three best examples.
I’ll explore different ways you can check the status of your GitHub Actions workflows using Node. js, the CLI, and manual methods — Dive in and keep track of your Action jobs!
What are GitHub Actions and GitHub API Software?
GitHub Actions and GitHub APIs provide very strong tools for integrating external services and automating CI/CD workflows.
A workflow is an event-driven process you schedule and automate with a YAML file.
You define workflows in the “.github/workflows” directory (inside a repository), and GitHub runs a job (or multiple jobs).
For example, on each pull request, you can:
- Run tests and generate code coverage reports.
- Use linters.
- Run security and performance checks.
- Update and check for outdated dependencies.
- Send notifications.
- Trigger any external APIs for specific actions in your repository.
Or, you can:
- Deploy to production or staging environments every time code is pushed to the main branch.
- Create releases and generate release notes whenever you push a new tag to the repository.
- Deploy every time you release.
- Build and publish Docker images to container registries (Docker Hub, GitHub Container Registry, etc…)
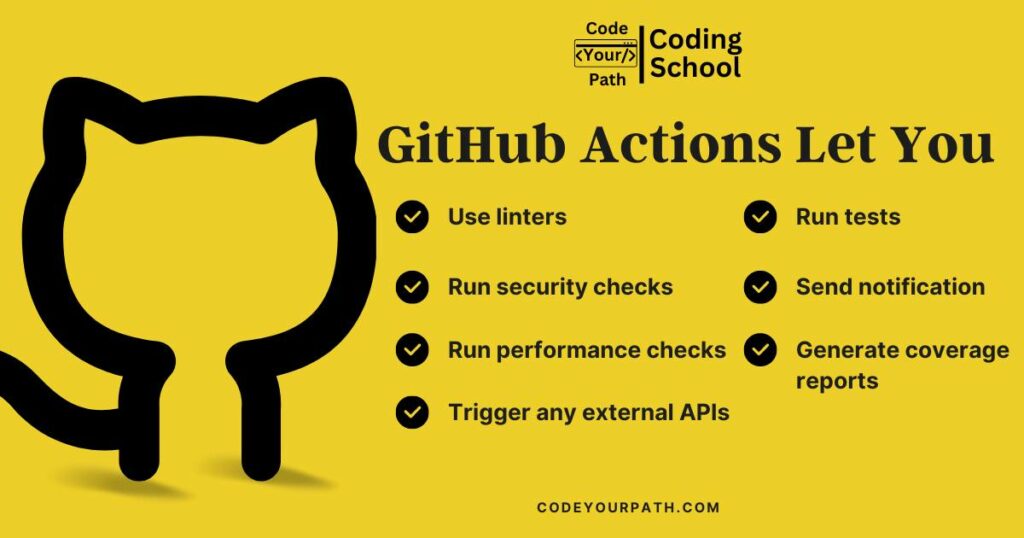
GitHub marks commits with statuses such as “error“, “failure“, “pending“, or “success” and reflects them in pull requests, making it easy to track the progress (and health) of your code.
You can use GitHub Actions to let CI (continuous integration) services (Jenkins, Docker, etc.) mark commits with either passing or failing builds. Note: GitHub provides the target_url param to specify the full build output.
When you push code to a repository, GitHub can automatically:
- Create a check suite with the last commit and send the check_suite event (with the action you request) to your GitHub App.
- Create new check runs for the last commit and add them to the appropriate check suite.
This check suite contains a collection of check runs, summarizing the status and conclusion of those check runs.
The status can be: queued, in_progress, completed, waiting, requested, pending.
Example 1: Manual Check Of a GitHub Workflow With UI
To check the status of a GitHub Action manually, go to the GitHub repo and click on the “Actions” tab (in the menu bar just below the repository name).
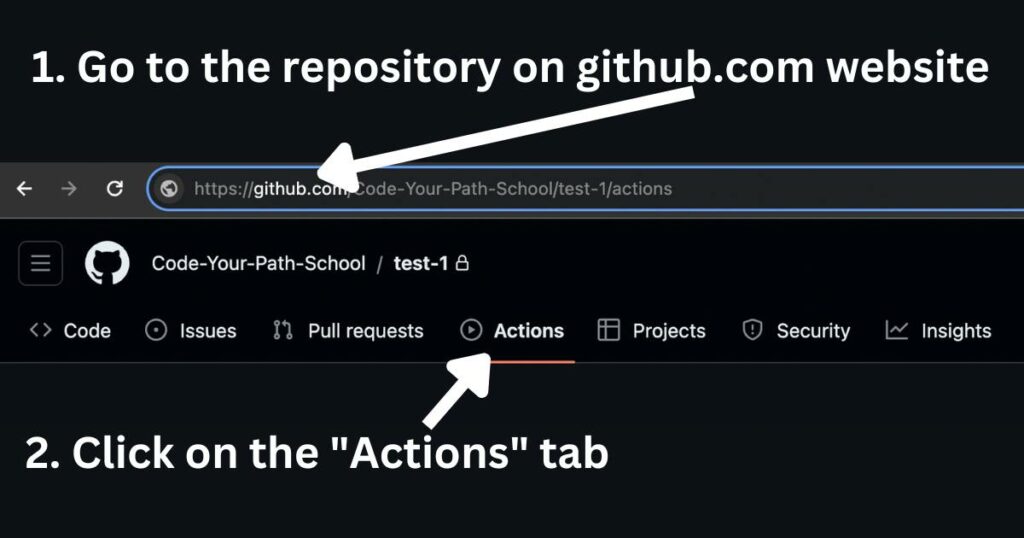
The “Actions” tab displays all workflows for the repository. Click on the workflow name you want to check, and GitHub will show a list of all runs for that workflow.
Each run is marked with a status indicator:
- A green check mark — success
- A red cross — failure
- A yellow dot — in-progress or pending
You can click on a specific run to view more details (all the jobs and steps).
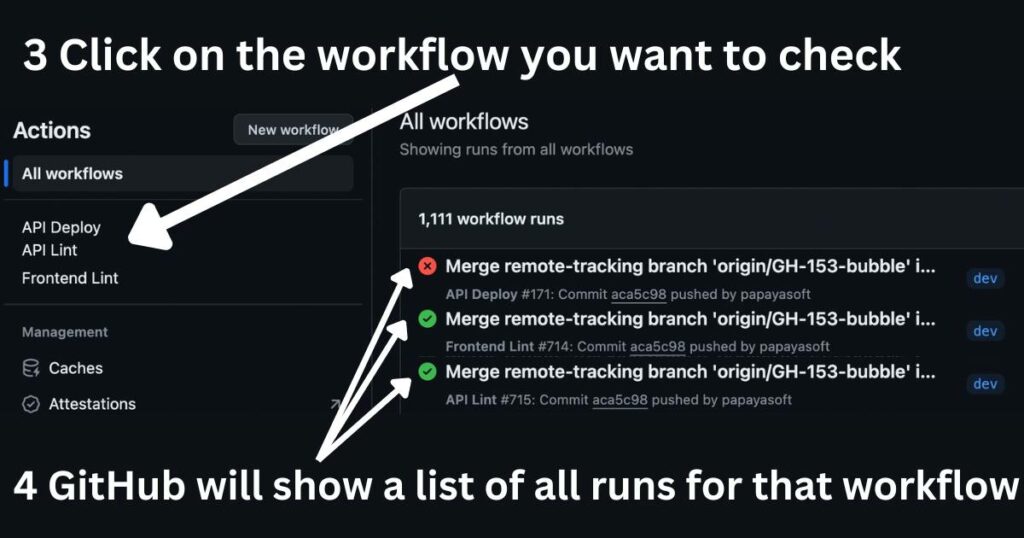
Example 2: Get an Action Status From A Check Run (With Node Js or Any Other Platform)
In the world of GitHub, there are categories of status checks: Commit Statuses and Check Runs.
When GitHub runs workflow Actions, it creates (triggers) check runs, not commit statuses. To get the state of a Github action, you can simply fetch a check run status.
Use the GitHub Status API to automate this process!
Here’s how you can do it using Node.js and the Octokit library, but you can also use other tools like Axios.
Initialize Octokit App With Your Personal Access github_token:
const { Octokit } = require("@octokit/rest"); // Your GitHub personal access token (PAT) const GITHUB_TOKEN = "your_github_token"; // Repository details const owner = "your_github_username_or_organization"; const repo = "your_repository_name"; // Initialize Octokit with the provided token const octokit = new Octokit({ auth: GITHUB_TOKEN, });
Find The Git Check Run ID — List All Check Runs:
You can list the check runs for a particular commit reference to fetch the check run ID and query its status.
The “ref” param must be a SHA, a branch name, or a tag name — here’s an Octokit snippet to do this:
await octokit.request("GET /repos/{owner}/{repo}/commits/{ref}/check-runs", { owner: 'OWNER', repo: 'REPO', ref: 'REF', headers: { 'X-GitHub-Api-Version': '2022-11-28' } });
Or you can list all check runs for a certain check suite and choose the one you want:
await octokit.request("GET /repos/{owner}/{repo}/check-suites/{check_suite_id}/check-runs", { owner: 'OWNER', repo: 'REPO', check_suite_id: 'CHECK_SUITE_ID', headers: { 'X-GitHub-Api-Version': '2022-11-28' } });
Please see the GitHub API documentation to learn more ways how you can retrieve the check_run_id.
Ensure The Status Of The Check Run Code
Once you have the ID, use an Octokit npm package to get a check run (you can use other tools like fetch or Axios):
const octokit = new Octokit({ auth: 'YOUR-TOKEN' }); const response = await octokit.request('GET /repos/{owner}/{repo}/check-runs/{check_run_id}', { owner: 'OWNER', repo: 'REPO', check_run_id: 'CHECK_RUN_ID', headers: { 'X-GitHub-Api-Version': '2022-11-28' } });
The “response” will contain the “status” field and other body fields:
{ "id": 5, "head_sha": "ce587453ced02b1526dfb4cb910479d431683101", "url": "https://api.github.com/repos/github/my-repo/check-runs/4", "status": "completed", // other fields... }
Important Notes:
- If you are looking for Actions on forked branches, Checks APIs won’t detect anything and send an empty pull_requests array instead.
- Enable the the
repo
scope for your OAuth app (or personal access) tokens to use Checks API.
Example 3: Get GitHub Status Of an Action From A Check Suite (With GitHub CLI API)
Another option to get the status of GitHub actions is to fetch a check suite status.
Here, I’m working directly in the command line, using the GitHub CLI tool — this method provides a clear, command-line-based interaction:
# GitHub CLI API details # Official docs: https://cli.github.com/manual/gh_api gh api \ --method PATCH \ -H "Accept: application/vnd.github+json" \ -H "X-GitHub-Api-Version: 2022-11-28" \ /repos/OWNER/REPO/check-suites/preferences
Or, here’s a simple cURL command that accomplishes the same task:
curl -L \ -X PATCH \ -H "Accept: application/vnd.github+json" \ -H "Authorization: Bearer <YOUR-TOKEN>" \ -H "X-GitHub-Api-Version: 2022-11-28" \ https://api.github.com/repos/OWNER/REPO/check-suites/preferences
When you execute either of the above commands, you’ll receive a JSON response with the check suite details (including its status):
{ "id": 5, "head_branch": "master", "head_sha": "d6fde92930d4715a2b49857d24b940956b26d2d3", "status": "completed", // other fields... }
Multiple Ways Of Programming Actions Workflows In Github Project
GitHub Actions is quite flexible, allowing you to run any command on your source code, catering to various needs and use cases.
But once you have that flexibility, here is the common dilemma: Do you do two distinct workflows with different jobs, or do you have two distinct steps within the same job?
The best will depend on the work context: performance considerations, execution time, metrics or maintenance.
There is NO one-size-fits-all — it is important to look at your project’s needs and constraints.
Review the following article on Best practices in CI/CD. BUT most of the time, there isn’t one right answer — I recommend trying to test BOTH ideas.
Use GitHub CLI To Monitor Workflow Executions
GitHub CLI lets you monitor your workflow executions and examine the exit status for them. Use the following command to watch the run to ensure it completes without error:
gh run watch --workflow "<workflow-name>" --exit-status && echo "run completed and successful"
GitHub will monitor the workflow (continuously updates the status in the terminal until the run completes) and print a success message for the success case.
Use CLI to better understand GitHub and how it supports Actions workflows.
Engineer Conclusion: Check GitHub Action For Protected Branch
Keep an eye on your GitHub Actions workflow statuses to keep development pipelines running smoothly.
It’s easy to monitor all your GitHub Actions workflows — GitHub offers flexible solutions that adapt to fit any preferences and needs!
Whether you do it manually or automate the process, GitHub cares of your CI/CD pipelines